When programming, it is inevitable that you will run into runtime errors. These are errors that occur during the execution of your program and can be caused by a variety of factors, from incorrect input to buffer overflows. In this article, we will explore different strategies for handling runtime errors and the statements to use.
Understanding Runtime Errors
As software developers, we all know that runtime errors can be a real headache. These errors can occur at any time during program execution and can be caused by a variety of factors.
Definition of Runtime Errors
Runtime errors, also known as exceptions, are errors that occur when a program is running. These errors can cause the program to crash or display unexpected behavior. They can be caused by a variety of factors, including incorrect input, incorrect code logic, and data corruption.
For example, a null pointer exception can occur when a program attempts to access an object or variable that has not been initialized. A division by zero error can occur when a program attempts to divide a number by zero. An array index out of bounds error can occur when a program attempts to access an array element that does not exist.
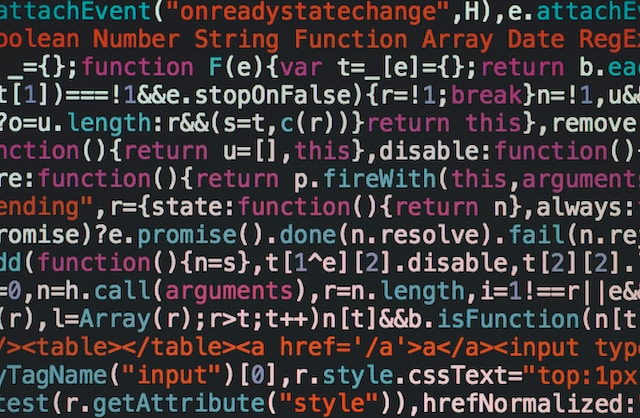
Common Types of Runtime Errors
Some common types of runtime errors include null pointer exceptions, division by zero errors, and array index out of bounds errors. These errors can be caused by a variety of factors, including incorrect input, incorrect code logic, and data corruption.
Another common type of runtime error is a stack overflow error, which occurs when a program’s call stack exceeds its maximum size. This can happen when a program has too many recursive function calls or when it allocates too much memory on the stack.
The Impact of Runtime Errors on Program Execution
When a runtime error occurs, the program may crash or display error messages. This can interrupt the user’s workflow and cause frustration. In some cases, a runtime error can also lead to security vulnerabilities if the error is exploited by malicious actors.
It’s important to note that runtime errors can be difficult to diagnose and fix. They often require careful debugging and testing to identify the root cause of the error. In some cases, it may be necessary to rewrite entire sections of code to eliminate the error.
However, with proper error handling and testing, many runtime errors can be prevented before they occur. By anticipating potential errors and incorporating error handling code into your programs, you can minimize the impact of runtime errors on your program’s execution.
Strategies for Handling Runtime Errors
Handling runtime errors is an essential part of software development. Runtime errors occur when a program is running and can cause the program to crash or behave unexpectedly. There are several strategies that programmers can use to handle runtime errors effectively.
Error Prevention Techniques
One effective way to handle runtime errors is to prevent them from occurring in the first place. This can be achieved by implementing input validation, error checking, and other techniques that catch potential errors before they occur. Input validation involves checking user input to ensure that it meets certain criteria. For example, if a user is asked to enter a number, the program can check that the input is a valid number and not a string or other type of data. Error checking involves verifying that the program is functioning as expected and that there are no logical errors in the code. These techniques can help catch errors before they cause problems.
Another way to prevent errors is to use try-catch statements. These statements catch exceptions and handle them before they cause the program to crash. For example, if a program is trying to access a file that does not exist, the try-catch statement can catch the exception and provide an error message to the user.
Error Detection Methods
If errors do occur, it is important to detect them quickly so they can be addressed before they cause further damage. This can be achieved by implementing error detection methods, such as debugging tools and logging mechanisms that track errors and provide information on why they occurred. Debugging tools can help identify the source of the error and provide information on how to fix it. Logging mechanisms can record information about the error, such as the time it occurred and the state of the program at the time of the error. This information can be used to diagnose the problem and develop a solution.
Error Recovery Approaches
In some cases, it may be necessary to recover from a runtime error and continue executing the program. This can be achieved by implementing error recovery approaches, such as restoring the program’s state to a previous point or providing alternate functionality. Restoring the program’s state involves returning the program to a previous point before the error occurred. This can be done by using checkpoints or saving the program’s state periodically. Providing alternate functionality involves providing the user with an alternative way to accomplish the task that caused the error. For example, if a file cannot be opened, the program can provide the user with the option to create a new file.
Overall, handling runtime errors requires careful planning and implementation of various strategies. By preventing errors, detecting them quickly, and recovering from them effectively, programmers can create software that is reliable and robust.
Programming Language-Specific Error Handling
When it comes to programming, errors are bound to happen. Whether it’s a syntax error, a runtime error, or a logic error, it’s important to have a plan in place for handling them. Fortunately, many programming languages provide built-in error handling mechanisms to help developers deal with errors in a structured and organized way. In this article, we’ll take a closer look at error handling in Python, Java, and JavaScript.
Error Handling in Python
Python provides a convenient way to handle runtime errors using the try-except block. This allows you to catch exceptions and perform specific actions based on the type of error that occurred. For example, you can catch a file not found exception and prompt the user to select a different file. The try-except block is structured as follows:
try: # code that might raise an exceptionexcept ExceptionType: # code to handle the exception
The code inside the try block is executed first. If an exception occurs, the code inside the except block is executed instead. You can also catch multiple exception types using multiple except blocks:
try: # code that might raise an exceptionexcept ExceptionType1: # code to handle ExceptionType1except ExceptionType2: # code to handle ExceptionType2
In addition to handling built-in exceptions, Python also allows you to create and raise custom exceptions. This can be useful when you want to add more specific error handling or provide more detailed error messages to the user. Custom exceptions are created by defining a new class that inherits from the built-in Exception class:
class CustomException(Exception): pass
You can then raise the custom exception using the raise keyword:
if some_condition: raise CustomException("Something went wrong.")
Error Handling in Java
Java provides similar error handling capabilities as Python using the try-catch-finally block. This allows you to catch exceptions and provide alternative paths for program execution. The finally block is also useful for releasing resources that were used in the try block. The try-catch-finally block is structured as follows:
try { // code that might throw an exception} catch (ExceptionType e) { // code to handle the exception} finally { // code that will always execute}
The code inside the try block is executed first. If an exception occurs, the code inside the catch block is executed instead. The finally block is always executed, regardless of whether an exception was thrown or not.
Like Python, Java also allows you to create custom exception classes. This can be useful when you want to provide more detailed error messages or create more specific error handling logic. Custom exceptions are created by defining a new class that inherits from the built-in Exception class:
public class CustomException extends Exception { public CustomException(String message) { super(message); }}
You can then throw the custom exception using the throw keyword:
if (someCondition) { throw new CustomException("Something went wrong.");}
Error Handling in JavaScript
JavaScript also provides runtime error handling using the try-catch-finally statement. This allows you to catch exceptions and provide alternative paths for program execution. The finally statement is also useful for releasing resources that were used in the try statement. The try-catch-finally statement is structured as follows:
try { // code that might throw an exception} catch (exception) { // code to handle the exception} finally { // code that will always execute}
The code inside the try block is executed first. If an exception occurs, the code inside the catch block is executed instead. The finally block is always executed, regardless of whether an exception was thrown or not.
JavaScript also allows you to throw custom errors using the throw statement. This can be useful when you want to provide more detailed error messages or create more specific error handling logic. Custom errors are created by defining a new class that inherits from the built-in Error class:
class CustomError extends Error { constructor(message) { super(message); this.name = "CustomError"; }}
You can then throw the custom error using the throw keyword:
if (someCondition) { throw new CustomError("Something went wrong.");}
Conclusion
Handling runtime errors is an important skill for any programmer. By understanding different strategies for handling runtime errors and the statements to use, you can write more robust and error-free code. Whether you are programming in Python, Java, or JavaScript, there are a variety of tools and techniques available to make the error handling process easier and more effective.