Coding is a world of endless possibilities, where programmers strive to solve complex problems with elegant solutions. In Python, a versatile and widely-used programming language, the append() function plays a vital role in manipulating lists. Whether you’re a beginner or an experienced developer, understanding what append() means in coding is crucial to harnessing its potential. In this comprehensive guide, we’ll dive into the append() function in Python, exploring its syntax, practical examples, and frequently asked questions. By the end, you’ll be well-equipped to master this essential function and ace your next coding interview.
The append() Function in Python: Overview and Purpose
The append() function in Python serves as a fundamental tool for list manipulation. Lists, as one of the most versatile data structures in Python, allow you to store and organize collections of items. However, the real power of lists lies in their ability to grow dynamically, and that’s where the append() function comes into play.
The primary purpose of the append() function is to add elements to the end of an existing list. This functionality provides immense flexibility, as you can incrementally build lists, update them with new data, or even create lists from scratch. The append() function allows your code to adapt to changing requirements by accommodating additional items seamlessly.
By utilizing the append() function, you can avoid the limitations of fixed-size arrays and enjoy the benefits of dynamic data structures. It eliminates the need to manually manage indices or resize arrays, simplifying the process of working with collections of varying sizes. Whether you’re building a shopping cart for an e-commerce website, logging user activities, or processing large datasets, the append() function is a powerful asset in your Python toolkit.
Additionally, the append() function enhances code readability. By explicitly stating your intent to add an element to the end of a list, you make your code more expressive and self-explanatory. This clarity aids collaboration and makes it easier for others to understand and maintain your codebase.
In summary, the append() function in Python empowers you to expand and modify lists effortlessly. Its purpose lies in adding elements to the end of a list, enabling dynamic growth and manipulation. By embracing this function, you gain the ability to create adaptable, scalable, and readable code that can handle a wide range of data-driven scenarios.
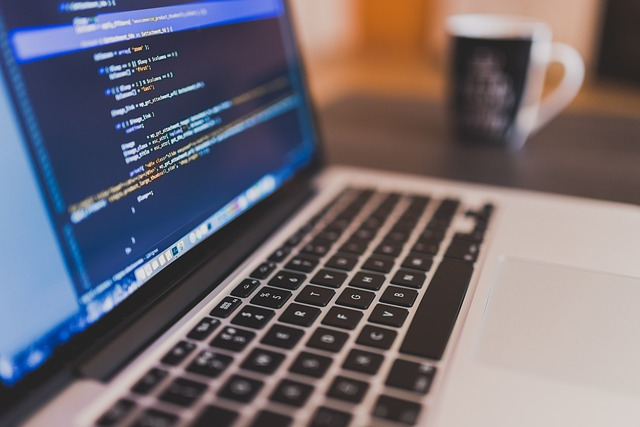
What Is the append() Function in Python and What Does It Do?
The append() function in Python is a built-in method specifically designed for list manipulation. Its purpose is to add a new element to the end of an existing list. When you invoke the append() function, it takes a single argument, which can be of any data type, and appends it to the end of the list. This function operates in-place, meaning it modifies the original list directly without creating a new list.
The append() function provides a convenient way to extend lists dynamically as your program runs. It allows you to handle situations where you don’t know the exact number of elements in advance or when the list size can vary over time. Instead of manually resizing the list or maintaining counters and indices, you can rely on append() to handle the expansion and adjustment of the list for you.
One important aspect to note is that append() adds the element as a single item at the end of the list. It does not attempt to merge lists or flatten nested lists. If you want to add the elements of another list to the end of the current list, you can use the extend() function instead.
The append() function is not limited to a specific data type. You can append elements of various types such as integers, strings, floats, booleans, or even other lists. This flexibility allows you to construct lists that contain heterogeneous data, enabling you to represent complex structures or collections of different entities within a single list.
By utilizing the append() function, you can build and update lists incrementally, one element at a time. This makes it ideal for scenarios where you receive data in a sequential manner or need to process items individually before incorporating them into the list. The append() function ensures that your code remains modular and adaptable, as you can add elements at any point during program execution without disrupting the existing list structure.
In summary, the append() function in Python is a powerful tool that adds an element to the end of a list. It modifies the list in-place, allowing for dynamic growth and manipulation. With its versatility and simplicity, append() empowers you to construct lists of varying sizes and types, adapting to the evolving needs of your program.
The append() Function in Python: Syntax
The syntax of the append() function in Python is straightforward and easy to grasp. To append an element to a list, you use the following syntax:

Here, list_name refers to the name of the list to which you want to add an element, while element represents the value or item you want to append. It’s important to note that element can be of any valid data type, including integers, strings, floats, or even other lists. The append() function seamlessly integrates with different data types, making it a versatile tool in your Python coding arsenal.
The append() Function in Python: Example
Let’s explore a practical example to illustrate how the append() function works. Consider the following snippet:
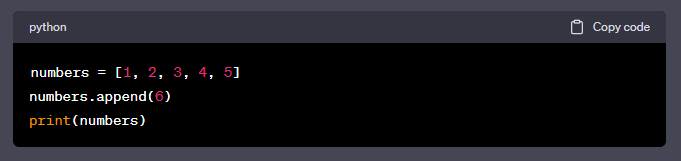
In this example, we have a list called numbers containing integers from 1 to 5. By invoking numbers.append(6), we add the number 6 to the end of the list. When we print the updated numbers list, the output will be: [1, 2, 3, 4, 5, 6]. As you can see, append() successfully appends the element to the existing list, expanding its size and preserving the order of the elements.
FAQs on the append Function in Python
Can append() add multiple elements to a list at once?
No, the append() function can only add one element at a time. If you want to add multiple elements simultaneously, you can use the extend() function. The extend() function takes an iterable as an argument, such as another list or a tuple, and appends each element individually to the end of the target list.
Does append() return a value?
No, the append() function in Python doesn’t return any value. It modifies the list in-place and doesn’t produce a new list. It’s important to remember this distinction when working with the append() function, as it means you cannot assign the result of append() to a variable or use it in expressions.
Can I append a list to another list using append()?
Yes, you can append a list to another list using the append() function. However, it’s essential to understand that when you append a list using append(), the entire list becomes a single element within the target list. In other words, the nested list retains its structure and is treated as a single item in the appended list. If you want to merge the elements of one list with another, you should use the extend() function instead.
Can append() add elements at a specific position within a list?
No, the append() function always adds elements to the end of a list. If you need to insert an element at a specific position, you can use the insert() function. The insert() function takes two arguments: the index at which you want to insert the element and the element itself. It shifts the existing elements to accommodate the new item.
Is append() exclusive to lists? Can I use it with other data structures?
The append() function is specific to lists in Python. It is not available for other data structures such as tuples, sets, or dictionaries. Each data structure has its own set of methods tailored to its specific behavior and requirements.
Are there any performance considerations when using append()?
While the append() function is efficient for adding elements to the end of a list, it’s important to note that inserting elements at the beginning or middle of a list using append() can be time-consuming. This is because every element after the insertion point needs to be shifted. If you frequently need to insert elements at arbitrary positions, you may consider using alternative data structures such as linked lists or deque, which offer more efficient insertions.
By addressing these frequently asked questions, you have gained a deeper understanding of the append() function in Python. These insights will enable you to leverage append() effectively in your code and make informed decisions about when and how to utilize this function.
Ready to Nail Your Next Coding Interview?
Mastering the append() function in Python is an essential step towards becoming a proficient programmer. Whether you’re preparing for a coding interview or seeking to improve your coding skills, understanding the intricacies of append() can give you a competitive edge. Here are some additional tips and recommendations to help you excel in your next coding interview:
- Practice with Real-World Examples: To solidify your understanding of append(), practice implementing it in various real-world scenarios. For example, simulate a shopping cart where you append items selected by a user, or create a program that builds a dynamic playlist by appending songs as they are selected. By applying append() to practical situations, you’ll gain hands-on experience and a deeper appreciation for its versatility;
- Understand Time and Space Complexity: While append() is a convenient function, it’s important to be aware of its time and space complexity. The append() function has an amortized time complexity of O(1), which means it is highly efficient for most cases. However, if you frequently insert elements at the beginning or middle of a list, the time complexity can increase to O(n) as all subsequent elements need to be shifted. Consider the requirements of your specific use case and choose the appropriate data structure accordingly;
- Explore Related List Operations: Append() is just one of many list operations available in Python. Take the time to explore and familiarize yourself with other useful list methods such as insert(), remove(), pop(), and index(). Understanding the full range of list manipulation functions will broaden your programming capabilities and enable you to choose the most suitable method for each task;
- Leverage Python’s List Comprehension: Python offers a powerful feature called list comprehension, which allows you to create new lists based on existing lists in a concise and efficient manner. By combining list comprehension with append(), you can perform complex transformations and filtering operations on lists. Practice using list comprehension alongside append() to enhance your ability to manipulate and transform lists effectively;
- Seek Feedback and Collaborate: Collaboration and feedback are invaluable in honing your coding skills. Engage in coding communities, participate in coding challenges, and seek feedback from experienced programmers. By exposing yourself to diverse perspectives and constructive criticism, you can refine your understanding of append() and improve your overall programming proficiency.
Remember, mastering append() is just one aspect of becoming a well-rounded programmer. Keep exploring other fundamental concepts and algorithms, and continuously challenge yourself to solve coding problems. By combining your knowledge of append() with a solid foundation in data structures and algorithms, you’ll be well-equipped to tackle any coding interview or project with confidence.
In conclusion, the append() function in Python is a powerful tool that allows you to add elements to lists dynamically. By practicing and understanding its nuances, you can leverage append() effectively in your code and demonstrate your proficiency in Python programming. Stay curious, keep learning, and embrace the endless possibilities that append() and Python offer. Good luck with your coding endeavors!
Conclusion
Age should never be a barrier to learning coding or pursuing a career in the tech industry. While it’s true that younger individuals may have a head start, adults can bring unique perspectives and strengths to the table. By embracing a growth mindset, setting clear goals, and following effective learning strategies, anyone can embark on a successful coding journey after the age of 30. Remember, the most important factor is your passion and determination to learn and grow. So, if you’ve ever dreamt of diving into the world of coding, don’t hesitate—start today and unlock your potential in the limitless realm of technology.