A loop functions by repetitively executing a set of instructions. However, there are instances where we need to halt the loop prematurely based on certain conditions. In C#, there exist multiple techniques to cease the loop ahead of schedule. Let’s delve into these strategies and explore their implementations.
Mastering Loop Control: Leveraging Break for Early Exit
In the realm of programming, especially in languages like C#, understanding how to wield loop control statements efficiently can significantly enhance the elegance and functionality of your code. One such powerful tool in your arsenal is the ‘break’ statement. When judiciously deployed within a loop structure, it acts as a swift terminator, abruptly halting the loop’s execution, and propelling the program flow forward.
Unveiling the Break Statement’s Dynamics
The ‘break’ statement serves as an escape hatch from the clutches of a loop, emancipating the program when certain conditions are met. Here’s a closer look at its mechanics:
- Instantaneous Termination: Upon encountering the ‘break’ statement, the loop in which it resides promptly ceases execution, sparing the program from further iterations;
- Single Loop Interruption: It’s crucial to note that ‘break’ exerts its influence solely on the loop enclosing it. Nested loops remain unaffected, necessitating alternative strategies for their premature termination.
Strategic Employment of Break
While ‘break’ is a versatile tool, its optimal usage demands a nuanced approach. Consider the following scenarios:
- Early Exit Strategies: When specific conditions warrant an immediate exit from a loop, ‘break’ proves invaluable. Whether it’s encountering an invalid input or reaching a desired outcome, ‘break’ can swiftly steer the program towards the next course of action;
- Avoidance of Redundant Execution: Employing ‘break’ strategically can preempt redundant executions of loop bodies, optimizing runtime efficiency.
Navigating the Labyrinth: Break and Nested Loops
Navigating the terrain of nested loops requires finesse. While ‘break’ is a potent instrument for single loops, its efficacy diminishes within nested constructs. In such scenarios, alternative constructs like ‘goto’ or judicious loop restructuring often offer a more elegant solution.
Unlocking the Power of Early Exit: Practical Example
Let’s dissect a practical example showcasing the judicious use of ‘break’ within a do-while loop:
using System;
class Kodify_Example
{
static void Main()
{
string name = "", password = "";
do
{
Console.Write("What's your name? (Press Enter to stop) ");
name = Console.ReadLine().ToLower();
if (name == "")
{
break; // Exiting loop when name is empty
}
if (name != "jos")
{
continue; // Skipping iteration if name is not 'jos'
}
Console.Write("What's the secret password? ");
password = Console.ReadLine();
} while (password != "abc123");
if (password == "abc123")
{
Console.WriteLine("Welcome and thanks for logging in!");
}
}
}
In this example, the ‘break’ statement facilitates an early exit from the loop when the user opts to terminate the interaction by not providing a name.
Building Secure Login Console Application in C#
In this console application, we ensure secure user authentication by implementing a robust login system. The process involves creating two essential string variables: name and password. These variables serve as the foundation for user input and validation.
Establishing Input Validation
We initiate the authentication process within a do-while loop, ensuring that our code executes at least once. This loop structure guarantees that the program interacts with the user, soliciting necessary information and validating it effectively.
User Interaction and Input Handling
- Prompting for Name: The program utilizes Console.Write() method to request the user’s name, ensuring a personalized interaction. Upon receiving the input, we convert it to lowercase using ToLower() method for consistent handling;
- Handling Empty Input: An if statement verifies if the user submits an empty string, indicating pressing Enter without providing a name. In such cases, the loop is terminated early using the break statement, enabling intuitive program flow based on user actions;
- Validating User Name: Another if statement evaluates whether the provided name matches the expected value. If not, denoted by != “jos”, the program prompts the user to retry, utilizing the continue statement to initiate a new loop cycle. This meticulous validation enhances security by ensuring only authorized users proceed.
Password Verification and Access Control
- Password Entry: Upon successful name input, the program prompts the user for the password using Console.Write(). The entered password is stored securely within the password variable;
- Loop-based Password Validation: The do-while loop condition ensures continuous validation of the password. As long as the entered password differs from the expected value, abc123, the loop persists. This iterative approach fortifies the authentication process, preventing unauthorized access.
User Welcome Message
Upon successful authentication, the program concludes by displaying a warm welcome message to the user, acknowledging their successful login and expressing gratitude for their interaction.
Sample Output:
- Input: What’s your name? (Press Enter to stop) Sarah;
- Input: What’s your name? (Press Enter to stop) Andy;
- Input: What’s your name? (Press Enter to stop) Jos;
- Input: What’s the secret password? 123abc;
- Input: What’s your name? (Press Enter to stop) Jos;
- Input: What’s the secret password? abc123;
- Output: Welcome and thanks for logging in!
Harnessing the Power of Goto Statements to Optimize Loops in C# Programming
The goto statement in programming provides a means to direct the flow of code execution to a specific labeled statement within the program. This mechanism essentially allows the program to ‘jump’ to the designated label, bypassing intermediate code blocks. While goto can be used to exit loops, it’s essential to ensure that the target label is positioned outside the loop structure.
Understanding the Functionality of goto:
The goto statement serves as a powerful tool in certain scenarios, particularly for terminating loops prematurely. However, its usage warrants caution due to its potential to introduce complexity and decrease code readability.
Advantages of goto:
- Exiting Nested Loops: One of the notable strengths of goto is its capability to exit multiple nested loops simultaneously, providing a convenient escape mechanism;
- Flexibility in Control Flow: It offers a flexible control flow, enabling developers to navigate through the codebase with precision.
Considerations and Best Practices:
- Code Readability: While goto can offer solutions in specific contexts, its excessive use can lead to convoluted and hard-to-understand code. Hence, it’s generally recommended to minimize its usage;
- Alternative Constructs: In most cases, employing structured control flow constructs like break, continue, or redesigning the loop conditions is preferable over resorting to goto.
Application in Early Loop Termination:
Let’s delve into an example to illustrate how the goto statement can facilitate the premature termination of a for loop:
using System;
class Example
{
static void Main()
{
string[] names = new string[6]{
"Michelle", "Theresa", "Dyna",
"Scott", "Rajeesh", "Wouter"
};
int lettersSum = 0;
for (int i = 0; i < names.Length; i++)
{
Console.WriteLine("{0} - {1} letters", names[i], names[i].Length);
lettersSum += names[i].Length;
// Jump out of loop to the specified label
if (lettersSum > 18)
{
goto AfterLoop;
}
}
Console.WriteLine("Number of names collected: {0}", names.Length);
AfterLoop:
Console.WriteLine("\nLoop terminated early!");
}
}
In this code snippet, the loop iterates through an array of names, calculating the sum of the lengths of each name. The loop is designed to terminate prematurely if the accumulated sum exceeds a certain threshold (in this case, 18 characters). Upon encountering the termination condition, the goto statement redirects the program flow to the labeled statement AfterLoop, effectively bypassing any subsequent iterations.
Understanding the Power of C#’s Return Statement
In the world of C# programming, the return statement holds a remarkable power—it serves as the key to exiting methods effectively and elegantly. While it might seem like a small piece of syntax, mastering its usage can greatly enhance your code’s readability and efficiency.
The Essence of Return
At its core, the return statement simply transfers control out of a block of code. In simpler terms, it allows you to gracefully exit a method. Typically, you’ll find the return statement residing as the final line within a method. However, its utility extends beyond just the method’s end.
- Early Exit: By strategically placing return statements within a method, you can exit the method from any point, not just at its conclusion. This capability is particularly handy when dealing with loops, enabling you to break out of them swiftly;
- Nested Loop Handling: Imagine scenarios where you have nested loops within your method. With return, you gain the power to break out of multiple nested loops simultaneously, streamlining your code’s control flow.
Types of Returns
In C#, there are two primary ways to employ the return statement:
- Void Methods: For methods that don’t return any value (void methods), a simple return; suffices to exit the method;
- Non-Void Methods: In methods where you expect to return a value, you utilize return followed by an expression of the same type as the method’s return type.
Points to Remember
It’s crucial to bear in mind a few key points regarding the behavior of return statements:
- Method Termination: When a return statement is encountered, it effectively terminates the entire method’s execution;
- Skipping Subsequent Code: Any code following the return statement within the same method will be skipped. This implies that careful consideration must be given to the placement of return statements to ensure desired program behavior.
Applying Return in Practice: A Real-World Example
Let’s delve into a practical demonstration to solidify our understanding of how return statements work in action. Consider the following program snippet:
using System;
using System.IO;
class ExampleProgram
{
static void Main()
{
int count = CountSubstrings(@"C:\Temp\Example.txt", "e");
Console.WriteLine("The file has {0} lines with an 'e'.", count);
}
static int CountSubstrings(string fileName, string substring)
{
int count = 0;
string currentLine = "";
using (StreamReader reader = new StreamReader(fileName))
{
while ((currentLine = reader.ReadLine()) != null)
{
if (currentLine.Contains(substring))
{
count++;
}
// Stop counting when reaching the '@' character
if (currentLine.Contains("@"))
{
return count; // Ends the loop and method early
}
}
return count;
}
}
}
In this example:
- The CountSubstrings method takes a file name and a substring as input parameters;
- It reads lines from the specified file, counting occurrences of the given substring;
- If a line contains a special character ‘@’, the method promptly exits, returning the current count.
Exiting Loops Prematurely Using Exception Handling
When coding in C#, encountering errors during program execution is inevitable. However, with the power of exception handling, we can gracefully handle such errors and even control the flow of our loops. One remarkable feature of C# is the ‘throw’ statement, which allows us to generate exceptions explicitly, signaling to other parts of our code that something has gone awry.
Why Use ‘throw’ to Exit Loops?
While loops are essential constructs in programming, there are times when we need to exit them prematurely, especially upon encountering errors or certain conditions. The ‘throw’ statement provides a neat solution to this dilemma, enabling us to halt the execution of loops immediately, bypassing any remaining iterations. This is particularly useful when:
- A critical error occurs within the loop;
- It’s necessary to terminate the loop early due to specific conditions.
Best Practices for Utilizing ‘throw’ in Loop Control
Implementing ‘throw’ within loops requires careful consideration to ensure the robustness and stability of our code. Here are some best practices to keep in mind:
- Precise Exception Type: Be specific about the type of exception you throw. This aids in clarity and helps in handling exceptions more effectively downstream;
- Targeted Error Handling: Use ‘throw’ strategically where you want to handle errors promptly without propagating them further up the call stack unnecessarily;
- Encapsulate with Try-Catch: Always encapsulate your loop with a try-catch block to catch the thrown exceptions. Failing to do so might lead to unhandled exceptions, which could crash your program.
Early Termination in a Foreach Loop
Let’s delve into a practical example to illustrate how we can employ ‘throw’ to exit a foreach loop prematurely. Consider the following scenario:
using System;
using System.Collections.Generic;
using System.Linq;
class Program
{
static void Main()
{
List<List<int>> testScores = new List<List<int>>();
// Populate testScores with nested lists of test scores
try
{
foreach (List<int> scores in testScores)
{
double classAverage = scores.Average();
Console.WriteLine("Average test score: {0:0.00}", classAverage);
if (classAverage <= 50)
{
throw new Exception("Class' average is way too low!");
}
}
}
catch
{
Console.WriteLine("Ouch! Class scored too low.");
}
}
}
Understanding the Code
- We initialize a nested list named ‘testScores’ to store various sets of test scores;
- Each list within ‘testScores’ represents the scores of individual classes or groups;
- The foreach loop iterates over each set of scores, calculating the average and displaying it;
- If the average score falls below 50, an exception is thrown, indicating a critical issue with the class’ performance.
Understanding Jump Statements and Try/Finally Blocks
In the world of programming, particularly in C#, there exists a fascinating phenomenon when it comes to jump statements and try/finally blocks. These elements add layers of complexity and control to the flow of code execution, often surprising programmers with their behavior.
The Intriguing Interaction: Jump Statements and Try/Finally Blocks
Ordinarily, when a jump statement like break, goto, return, or throw is encountered within a code block, the program swiftly shifts its focus to the designated target, leaving the current context behind. However, this routine alters when a try/finally code block intervenes.
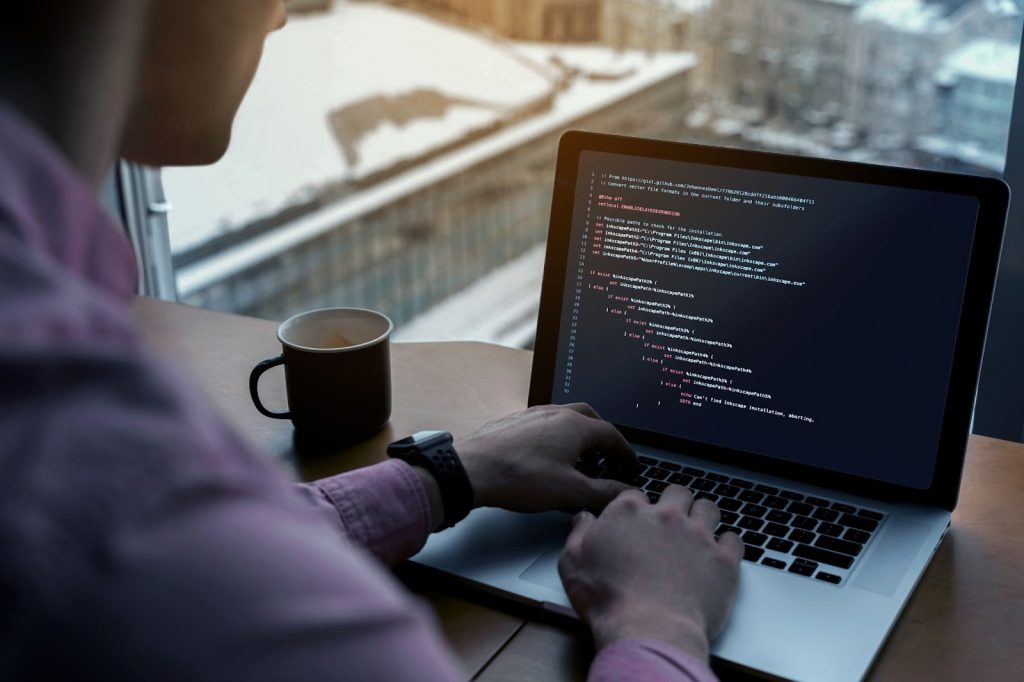
The Unveiling Mechanism: Try/Finally Block’s Impact
In C#, when a jump statement leads out of one or more try code blocks that are accompanied by a finally block, an interesting detour occurs. Instead of immediately heeding the call of the jump statement, the program diverts its attention to execute the finally blocks first. Only after this meticulous execution does it proceed to the destination dictated by the jump statement.
Visualizing the Phenomenon: An Example
Consider a scenario where a loop encapsulated within a try/finally block encounters an error condition and employs a jump statement like return to break out of the loop prematurely. Contrary to expectations, the program doesn’t make an abrupt exit. Instead, it gracefully traverses through the finally blocks before concluding the loop.
Example Program Walkthrough
Let’s delve into a practical illustration to grasp this behavior more vividly:
using System;
class Example
{
static void Main()
{
int[] values = new int[10]{
-10, -3, 0, 3, 5, 20, 50, 70, 90, 100
};
Console.WriteLine("Dividing values..");
DivideValues(values);
}
static void DivideValues(int[] values)
{
for (int i = 1; i < values.Length; i++)
{
try
{
try
{
Console.WriteLine("{0} / {1} = {2}",
values[i],
values[i - 1],
values[i] / values[i - 1]);
}
catch (DivideByZeroException)
{
Console.WriteLine("Oops! Divided by zero.");
Console.WriteLine("\tExiting loop with 'return'..");
return; // Break out of loop and method
}
finally
{
Console.WriteLine("\tInner finally block.");
}
}
finally
{
Console.WriteLine("\tOuter finally block.");
}
}
Console.WriteLine("Regular method exit point");
return;
}
}
Executing Custom Methods with Array Processing
In the Main() function, the initial step involves the declaration of an integer array, which serves as the input data for subsequent operations. Following this, the custom method DivideValues() is invoked to process the array effectively. However, the intricacies lie within this method, where a series of operations unfold to handle various scenarios seamlessly.
Processing Array Elements
The DivideValues() method employs a meticulous approach to iterate through each value within the array. This iteration is facilitated by a for loop, which meticulously navigates through the array elements to perform designated operations. Within this loop structure, the code encapsulates critical error handling mechanisms to ensure robustness and graceful handling of exceptions.
Handling Exceptions Gracefully
Within the loop structure, a try-catch-finally paradigm encapsulates the essential logic. Here’s how it unfolds:
- Try Block: This segment of code attempts the division operation, aiming to compute the result of dividing the current array value by the previous one. The Console.WriteLine() function plays a pivotal role here, rendering the computed values and pertinent information to the console output;
- Catch Block: In the event of encountering a divide-by-zero scenario, a DivideByZeroException is triggered, directing the program flow into the catch block. Within this block, a tailored error message is displayed via Console.WriteLine(), notifying users of the exceptional condition;
- Finally Block: Regardless of the outcome, the finally block ensures the execution of essential cleanup tasks. It encapsulates both inner and outer segments, offering a comprehensive approach to resource management within the loop.
Behavior Upon Exception
Upon encountering a divide-by-zero error, the program exhibits intriguing behavior. Despite the early termination of the loop initiated by the return statement within the catch block, the finally code blocks persist in execution. This behavior is highlighted in the program’s output, showcasing the continued execution of both inner and outer finally blocks.
Insightful Observations
- Execution Persistence: The program’s behavior underscores the persistent execution of finally code blocks, even in scenarios where explicit program flow alterations, such as return statements, are employed;
- Graceful Termination: Despite encountering exceptions and subsequent early exits from the loop, the program maintains integrity through the execution of essential cleanup operations encapsulated within finally blocks.
Implications in Control Flow
The program’s behavior underlines significant insights into control flow mechanisms within C#. Particularly, the interaction between try, catch, and finally blocks elucidates the nuanced handling of exceptions and resource management, offering valuable lessons for developers navigating complex code structures.
Exploring C#’s Break Statement
While this discourse primarily delves into the dynamics of exception handling, it also piques curiosity about other control flow constructs, such as the break statement. Understanding the interplay between break and exception handling mechanisms further enriches one’s comprehension of program control flow in C#.
Conclusion
In conclusion, mastering the art of loop termination in C# empowers programmers to wield greater control over their code’s execution flow. By understanding and utilizing the various methods available for stopping loops early, developers can enhance the efficiency and flexibility of their applications. Whether employing break statements, conditional checks, or other techniques, the ability to gracefully exit loops when necessary is a valuable skill in software development.
Add comment