Frequently, prior to integrating strings from external origins, our C# application necessitates a process of refinement. An effective technique for achieving this is by eliminating any excess whitespace. Now, let’s delve into the methodology of accomplishing this task within the realm of C#.
Aggressively Removing Whitespace Characters
Removing whitespace characters from a string is a common task in programming, and there’s a straightforward method to accomplish this. By filtering every character in the string and retaining only non-whitespace characters, we achieve a clean and concise output.
How it Works:
- Character Filtering: Using the LINQ method Where() on the source string, we iterate through each character;
- Whitespace Check: Inside the Where() method, we specify that each character c should not be a whitespace character, leveraging !Char.IsWhiteSpace(c);
- Result Concatenation: The filtered characters are then joined together into a new string using String.Concat().
Example Code:
using System;
using System.Linq;
class ExampleProgram
{
static void Main()
{
string example = " Hi there! ";
string trimmed = String.Concat(example.Where(c => !Char.IsWhiteSpace(c)));
Console.WriteLine($"Original string: {example}.");
Console.WriteLine($"Trimmed string: {trimmed}.");
}
}
Benefits:
- Efficiency: Removes unnecessary whitespace characters swiftly;
- Readability: Concise and easy-to-understand code;
- Customizable: Easily adaptable to specific whitespace removal needs;
- Compatibility: Applicable across various programming languages with similar syntax.
Mastering String Manipulation in C#: Trimming Whitespace and Filtering with LINQ
In the Main() method of our code, we embark on a journey of string manipulation, exploring the depths of C# functionalities. Here’s a detailed breakdown of our journey:
- Creating a Multi-line String: We kick off by crafting a multi-line string using the magical @ character, which allows us to preserve formatting and escape sequences within the string literal. This string, a tapestry of characters, serves as our playground for the upcoming transformations. We assign this rich tapestry to the variable example, ready to be unraveled and redefined;
- Trimming Whitespace: With our multi-line string captured, our next move is to trim away any trailing, leading, or excess whitespace. This ensures our subsequent operations are crisp and focused. Employing the trusty Trim() method, we meticulously groom the string, leaving no whitespace unscathed;
- Filtering with LINQ’s Where() Method: As adventurers in the realm of LINQ, we invoke the powerful Where() method on our example string. Within its parentheses lies our filtering condition, a quest to exclude every character that masquerades as whitespace. Through the arcane Char.IsWhiteSpace() method, we discern the true nature of each character, separating the wheat from the chaff;
- Concatenating Filtered Characters: The Where() method bestows upon us a sequence of individual characters, each a potential protagonist in our narrative. To reunite these characters into a cohesive tale, we enlist the aid of String.Concat(), weaving them together into a new string, free from the shackles of whitespace. This amalgamation, dubbed exampleTrimmed, stands as a testament to our mastery over strings and sequences;
- Revelation Through Console.WriteLine(): With our journey nearing its conclusion, we unveil the fruits of our labor through the venerable Console.WriteLine() method. Two invocations grace the screen, one showcasing the original unadulterated string, a relic of the past, while the other presents its trimmed counterpart, streamlined and refined. Behold the transformation:
Original string:
Hello,
World
.
Trimmed string:
Hello,World.
Reflecting on the Whitespace Exodus: Let us pause to appreciate the significance of our endeavor. Not only have we liberated our string from the clutches of extraneous whitespace, but we have also cleansed it of ambiguity and disorder. From the cryptic depths of multi-line obscurity emerges a string, bold and unyielding, ready to face the challenges that lie ahead.
Utilizing C#’s Trim() Method: Removing Whitespace Creatively
Whitespace, the silent occupant of strings, often hides within the text, making it appear untidy or causing unexpected behavior in programs. Fear not, for C# provides a simple yet powerful solution – the Trim() method. Let’s embark on a journey to explore its wonders and unveil its magic.
Understanding Trim() Method
The Trim() method in C# is akin to a master cleaner, adept at removing unwanted spaces, tabs, line breaks, or any other whitespace characters from both ends of a string. Here’s a closer look at its functionality:
- Whitespace Removal: Without arguments, Trim() meticulously purges all whitespace characters from the beginning and end of a string;
- Return Value: After its cleansing ritual, Trim() graciously presents a modified version of the original string, now devoid of unnecessary spaces.
Example Program Unveiled
To witness Trim() in action and grasp its efficacy, let’s delve into a practical example program:
using System;
class Whitespace_Eradicator
{
static void Main()
{
string example = @"
Hello,
World
";
string exampleTrimmed = example.Trim();
Console.WriteLine($"Original string: {example}.");
Console.WriteLine($"Trimmed string: {exampleTrimmed}.");
}
}
In this enlightening snippet, we unleash the power of Trim() to tame the unruly whitespace within the string example. Brace yourselves as we witness the metamorphosis from chaos to clarity.
Exploring the Results
Behold the transformation brought forth by the Trim() method:
- Original String: The unrefined example string, riddled with excessive whitespace, stands before us in all its disorderly glory;
- Trimmed String: Witness the marvel as Trim() works its magic, presenting the pristine version of the string, devoid of any extraneous spaces or clutter.
Example String Manipulation in C#
Creating the Example String: Let’s start by creating an example string with multiple lines and various whitespace characters. We’ll store this string in a variable named example.
string example = @"Hello, World.";
This string contains spaces, tabs, and newlines, providing a realistic scenario for string manipulation.
Trimming the String: The objective here is to remove any leading or trailing whitespace characters from the string. To achieve this, we’ll utilize the Trim() method in C#.
string exampleTrimmed = example.Trim();
The Trim() method efficiently removes whitespace characters from both the beginning and end of the string.
Displaying Original and Modified Strings:
Now, let’s output both the original and modified strings using the Console.WriteLine() method to observe the changes.
Console.WriteLine("Original string:\n" + example);
Console.WriteLine("Trimmed string: " + exampleTrimmed);
This allows us to compare the original string with the trimmed version, highlighting the impact of the Trim() method on whitespace removal.
Utilizing C#’s TrimStart() Method
In C#, handling strings often involves managing whitespace, which can clutter and affect the readability of text. Fortunately, C# offers a method called TrimStart() to alleviate this issue.
What is TrimStart()?
TrimStart() is a method in C# that eliminates leading whitespace characters from the beginning of a string, leaving the rest of the string intact. This method doesn’t alter the original string; instead, it returns a modified copy with the leading whitespace removed.
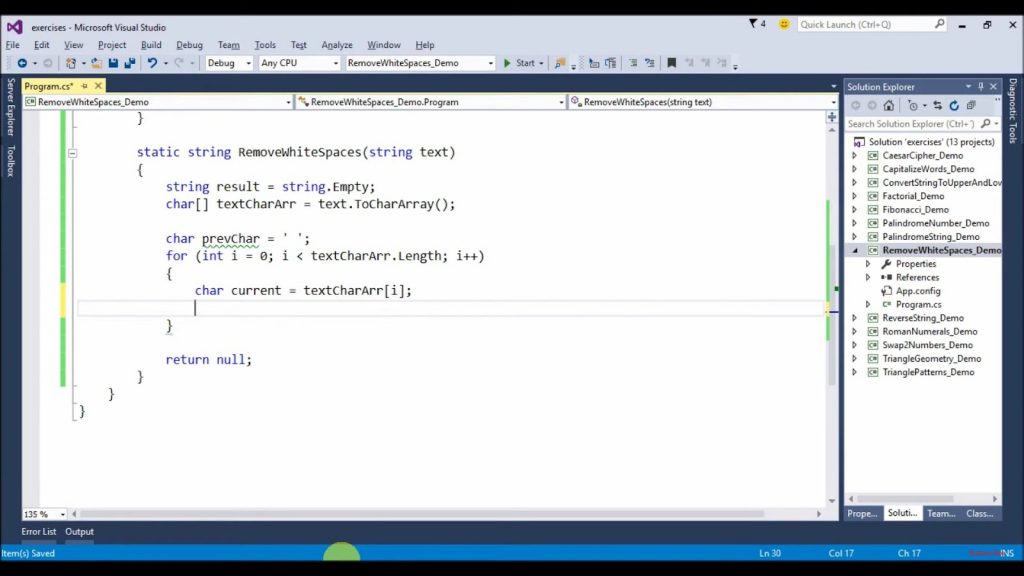
Example Usage: Let’s delve into a practical example to understand how TrimStart() works:
using System;
class ExampleProgram
{
static void Main()
{
string example = @"
Hello,
World
";
string exampleTrimmed = example.TrimStart();
Console.WriteLine($"Original string: {example}.");
Console.WriteLine($"Trimmed string: {exampleTrimmed}.");
}
}
Explanation of the Program:
In the provided code, we have a multi-line string stored in the example variable, encapsulated within @””. This string contains leading whitespace, making it less readable.
Next, we invoke the TrimStart() method on the example string. This action removes any leading whitespace characters, producing a cleaned version of the string stored in the exampleTrimmed variable.
Output: Upon executing the program, the output demonstrates the transformation:
Original string:
Hello,
World
.
Trimmed string: Hello,
World
.
Unveiling the Fourth Dimension of String Manipulation: Stripping Trailing Whitespace
In the realm of string manipulation, one often encounters the need to refine text by removing superfluous whitespace. Embracing the elegance of C#, the TrimEnd() method emerges as a stalwart companion, facilitating the seamless elimination of trailing spaces. Let’s embark on an enlightening journey to delve deeper into its nuances and practical applications.
The TrimEnd() Method: A Closer Encounter
The TrimEnd() method stands poised, ready to embark on its mission of whitespace eradication. Here’s a glance at its syntax:
string example = " Hi there! ";
string trimmed = example.TrimEnd();
- Invocation: Without any arguments, TrimEnd() graciously extends its services to excise trailing whitespace, leaving the core essence of the string intact;
- Return Value: A pristine rendition of the original string, liberated from the shackles of trailing spaces, is ushered forth.
Unveiling the Mechanics: A Programmatic Exploration
Let’s embark on a journey through a console application to witness TrimEnd() in action:
using System;
class StringManipulationDemo
{
static void Main()
{
string example = @"
Hello,
World
";
string exampleTrimmed = example.TrimEnd();
Console.WriteLine($"Original string: {example}.");
Console.WriteLine($"Trimmed string: {exampleTrimmed}.");
}
}
- String Initialization: Behold, a multi-line string adorned with whitespace, awaiting its transformation;
- Invocation of TrimEnd(): With a simple invocation, we bid farewell to trailing spaces, invoking the method upon our string instance;
- Observation of Transformation: Witness the metamorphosis as we compare the original string with its refined counterpart.
Deciphering the Console Output: A Tale of Transformation
Behold the narrative woven by the console application:
Original string:
Hello,
World
.
Trimmed string:
Hello,
World.
- A Symphony of Whitespace: The original string, resplendent with spaces, beckons for refinement;
- Trimmed Brilliance: Through the deft touch of TrimEnd(), trailing whitespace is gracefully excised, preserving the essence of the text.
Conclusion
In conclusion, ensuring the cleanliness and integrity of strings sourced externally is a crucial aspect of programming in C#. By adeptly removing whitespace, we enhance the reliability and efficiency of our programs. This process not only streamlines data handling but also contributes to the overall robustness of the application. As developers, mastering such techniques empowers us to create more resilient and efficient software solutions in the C# ecosystem.
Add comment