The foreach loop stands as a fundamental tool in the toolkit of every C# programmer, providing an easy and intuitive method for traversing collections. Yet, as the landscape of software development advances, the methodologies and instruments available to us also progress. LINQ (Language Integrated Query) extension methods introduce robust querying features to C#, enabling developers to work with collections using expressive and succinct language. In this discussion, we will delve into how LINQ extension methods can transform the functionality of foreach loops, thereby improving the clarity and upkeep of code.
Bypassing the First Item in a Foreach Loop
A common requirement while dealing with loops in programming is skipping certain values, typically the first few. Standard loops like ‘for’ and ‘while’ allow easy implementation of this requirement by simply commencing the index count at a value other than zero. However, the foreach loop doesn’t offer such straightforward functionality. So how can we work our way around this?
C#’s rich set of LINQ methods comes to the rescue here. The ‘Skip()’ LINQ method leaves out a specified number of elements from the beginning of a collection. Incorporating this method prior to starting the loop enables us to exclude the first or more elements from the collection being processed.
Let’s illustrate this with an example:
using System;
using System.Collections.Generic;
using System.Linq;
// ...
List<int> values = new List<int>()
{
9, 26, 77, 75, 73, 77,
59, 93, 9, 13, 64, 50
};
// Bypass the first element during iteration
foreach (int value in values.Skip(1))
{
Console.Write(value + " ");
}
In the above code, a list holding a sequence of integers is declared. The foreach loop is then utilized to iterate over the list. However, before the iteration commences, the ‘Skip(1)’ method is applied to the list. This tells the loop to exclude the first integer value during the iteration.
Inside the loop, the ‘Console.Write()’ method prints each value. Thanks to the use of the ‘Skip()’ LINQ method, the loop output starts from the second value in the list, successfully bypassing the very first entry.
While this example illustrates skipping just the first element in a collection, note that the ‘Skip()’ method can be used with any number as argument to exclude an arbitrary number of elements from the start.
Enhancing Foreach Loop Functionality with Skip()
Often, when working with loops, there might be a need to exclude certain elements. While conventional loops like ‘for’ or ‘while’ offer the convenience of simply initiating the index from a non-zero value to accomplish this, the foreach loop doesn’t extend the same functionality. Can one still skip certain elements using the foreach loop?
Yes, with the use of LINQ’s versatile ‘Skip()’ method, it’s possible to exclude any number of elements from the beginning of a collection while utilizing a foreach loop. This method, when invoked before the commencement of the loop, ensures the initial elements are bypassed.
Consider the following example:
using System;
using System.Collections.Generic;
using System.Linq;
List<int> values = new List<int>()
{
9, 26, 77, 75, 73, 77,
59, 93, 9, 13, 64, 50
};
// Exclude the initial two elements from the loop
foreach (int value in values.Skip(2))
{
Console.Write(value + " ");
}
In this code snippet, we first create a list comprising several integers. Next, a foreach loop is constructed to traverse the elements of the ‘values’ collection. However, before the loop starts running, the ‘Skip()’ method is applied to the list with argument ‘2’, implying the initial two elements are to be left out during the iteration.
The ‘Console.Write()’ command within the loop prints the elements iterated over, confirming that the first two elements from the original list have indeed been passed over.
It’s noteworthy that the ‘Skip()’ method does not modify the original list but instead generates a new enumerable that begins after the specified number of elements. As such, you can use it to skip any number of elements from the start of a collection simply by adjusting the argument value.
While the ‘continue’ statement could also be used within the loop to skip over certain elements, it incurs the overhead of the loop running over those items before skipping them, making it less efficient. In contrast, the ‘Skip()’ method effectively streamlines the code while achieving increased efficiency.
Utilizing Foreach Loop to Exclude the Last Element
Just as skipping the initial elements of a foreach loop is possible, bypassing the final element or set of elements is an equally viable operation. Traditional loops, such as for and while, have a straightforward way of achieving this by simply iterating until they reach the ‘length – 1’ index. The foreach loop, however, isn’t as straightforward in this regard and necessitates a different approach.
The LINQ extension method, ‘Take()’, provides a feasible solution to this problem. The ‘Take()’ method retrieves a specified quantity of elements from a collection. So, when the requirement is to skip the last entity, ‘Take()’ can be utilized to fetch all elements except the last one. Moreover, the number of elements in a collection can be ascertained using the ‘Count()’ LINQ method.
Here’s an example of utilizing these two extension methods within a foreach loop:
using System;
using System.Collections.Generic;
using System.Linq;
List<int> values = new List<int>()
{
9, 26, 77, 75, 73, 77,
59, 93, 9, 13, 64, 50
};
foreach (int value in values.Take(values.Count() - 1))
{
Console.Write(value + " ");
}
In this code illustration, a list of integers is first declared. Then, a foreach loop is coded to traverse the ‘values’ collection. Before the loop is initiated, the ‘Take()’ method is invoked on the list. By passing ‘values.Count() – 1’ as the argument to ‘Take()’, the method is directed to fetch all elements except the last one.
As the loop iterates over the list, the ‘Console.Write()’ method outputs each value, confirming that the last element is effectively passed over.
In this manner, using ‘Take()’ with ‘Count()’ converts a simple foreach loop into a dynamic construct that can capably bypass specific elements based on your requirements. However, remember that ‘Take()’ generates a new enumerable without altering the original list, so the original data is still intact for any further operations you might want to perform.
Excluding Specified Number of Final Elements with Foreach Loop
Just like it’s possible to bypass the first element(s) in a foreach loop, the loop is equally capable of selectively excluding a chosen number of values from the end. Despite being natural in a for or while loop, similar behavior in a foreach loop necessitates an alternative solution.
The LINQ extension method, ‘Take()’, offers an elegant workaround in this context. This method returns a specific number of elements from a collection. It facilitates the skipping of the final item by taking all elements, excluding the last one. The ‘Count()’ LINQ method can be exploited to determine the number of elements in the collection.
Here is a demonstration of combining these two methods within a foreach loop:
using System;
using System.Collections.Generic;
using System.Linq;
List<int> values = new List<int>()
{
9, 26, 77, 75, 73, 77,
59, 93, 9, 13, 64, 50
};
// Exclude the last two elements from the loop
foreach (int value in values.Take(values.Count() - 2))
{
Console.Write(value + " ");
}
This code initiates with the declaration of a list of integers. Subsequently, a foreach loop is coded, intended to traverse the ‘values’ collection. However, before the loop execution starts, the ‘Take()’ method is invoked on the list, along with the ‘Count()’ method to determine the number of elements. The ‘Take()’ method is passed an argument of ‘values.Count() – 2’, implying it will include all elements except the last two in the iteration.
The ‘Console.Write()’ command prints the iterated values, thereby confirming that the last two elements of the list have been successfully excluded.
Do note that, much like the ‘Skip()’ method, ‘Take()’ also generates a new enumerable from the collection without altering the original list, hence preserving the original data. Therefore, using ‘Take()’ in conjunction with ‘Count()’ allows a foreach loop to skip a specified number of elements from the end, offering a flexible and efficient solution.
Alternate Approach for Skipping Odd Indexes
In programming, skipping odd indexes is a common task, especially when dealing with loops. While using a for loop to accomplish this is straightforward by adjusting the index increment, employing a foreach loop requires a different strategy due to its lack of direct control over the iteration index. However, fear not, as there’s a clever alternative using LINQ’s Where() method.
The LINQ Where() Method
The Where() method in LINQ is a powerful tool for filtering elements based on specific criteria. In the context of skipping odd indexes, its overloaded version allows filtering elements by their index, enabling us to precisely control which elements get processed.
- List Initialization: Begin by initializing a list with the desired elements;
- Filtering with Where(): Utilize the Where() method, passing a lambda expression that includes both the value and index of each element;
- Modulus Operation: Within the lambda expression, use the modulus operator % to determine if the index is even;
- Iteration: Iterate over the filtered collection, processing only the elements with even indexes.
Example Implementation
using System;
using System.Collections.Generic;
using System.Linq;
// List initialization
List<int> values = new List<int>()
{
9, 26, 77, 75, 73, 77,
59, 93, 9, 13, 64, 50
};
// Filtering with Where() method
foreach (int value in values.Where((number, index) => index % 2 == 0))
{
Console.Write(value + " ");
}
Breakdown of the Example
- List Initialization: Create a list named values containing twelve integer values;
- Filtering with Where(): Use the Where() method to filter the collection based on the condition index % 2 == 0, ensuring only elements with even indexes are retained;
- Iteration: The foreach loop iterates over the filtered collection, printing out values with even indexes.
Benefits and Applications
- Simplicity: This approach provides a concise and readable solution for skipping odd-indexed elements;
- Flexibility: The flexibility of LINQ allows for versatile filtering criteria beyond simple index-based skipping;
- Performance: While LINQ introduces some overhead, for most scenarios, the performance impact is negligible compared to the clarity and maintainability gained.
Exploring the Power of C# Foreach Loop with Odd Index Selection
Diving into the realm of C# programming, developers often encounter scenarios where they need to manipulate collections in specific ways. One such common task involves iterating through a collection and selecting elements based on their index properties. Fortunately, C# offers a versatile solution through the foreach loop, coupled with LINQ methods like Where(). In this guide, we’ll uncover how to leverage the foreach loop to selectively include elements with odd indexes, excluding the even ones.
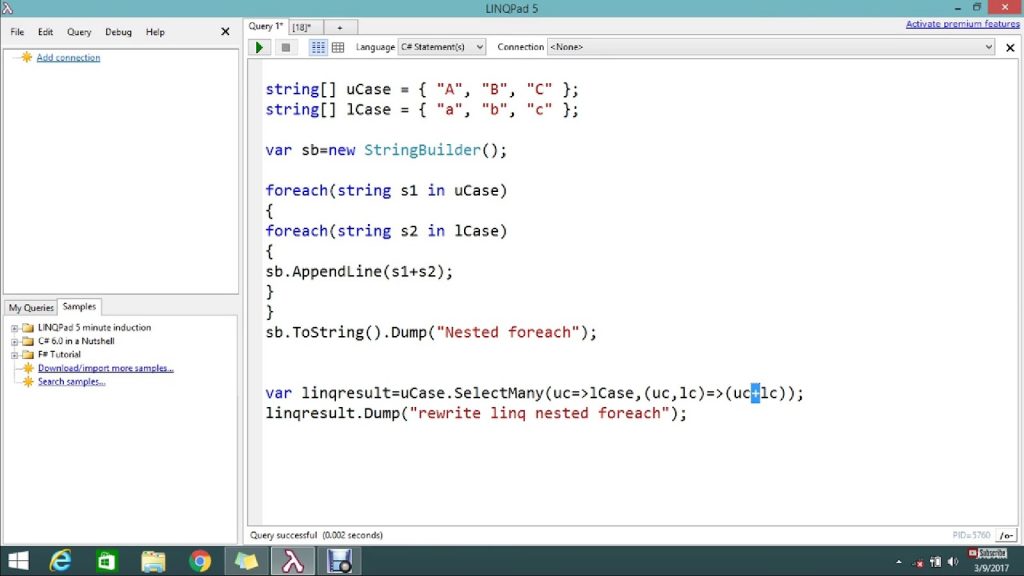
Understanding the Concept
Before we delve into coding intricacies, let’s grasp the core concept. When we talk about odd indexes in a collection, we’re referring to positions that aren’t multiples of 2. For instance, in a collection of integers [9, 26, 77, 75, 73, 77, 59, 93, 9, 13, 64, 50], elements at positions 1, 3, 5, 7, 9, and 11 represent odd indexes.
Implementing the Solution
Now, let’s translate this concept into code using C#. Here’s a breakdown of the implementation:
- Building the Collection: First, we create a list of integers containing various numerical values. This list serves as our data source for the demonstration;
- Utilizing the foreach Loop: We employ a foreach loop to traverse through each element in the collection. Within this loop, we specify a local variable, ‘value,’ to represent each item during iteration;
- Selective Inclusion with Where() Method: To filter out elements with odd indexes, we utilize the Where() LINQ method. Within its parentheses, we create a tuple (number, index) to access both the value and its index in the collection. By applying the modulus operator (%) to the index, we discern whether it’s an odd or even position. In this case, an index is considered odd if index % 2 != 0;
- Printing Selected Values: Inside the loop, we utilize Console.Write() to output each selected value. Since we’ve filtered out elements with even indexes, only those with odd indexes will be printed.
Example Demonstration
Let’s put theory into practice with a snippet:
using System;
using System.Collections.Generic;
using System.Linq;
// ...
List<int> values = new List<int>()
{
9, 26, 77, 75, 73, 77,
59, 93, 9, 13, 64, 50
};
foreach (int value in values.Where((number, index) => index % 2 != 0))
{
Console.Write(value + " ");
}
Outcome Analysis: Upon executing the code, the output will showcase only the values corresponding to odd indexes (1, 3, 5, 7, 9, and 11) from the original collection. This selective inclusion demonstrates the practicality and efficiency of leveraging C# features for tailored data manipulation.
Conclusion
In conclusion, while the foreach loop in C# provides a convenient means of iterating through collections, its limitations in terms of directional looping and subset iteration can be overcome with the utilization of specialized methods available within the language. By leveraging these methods, developers can enhance the flexibility and efficiency of their code, thereby optimizing the looping process in C#.
Add comment