Coding can be a complex field, full of technical jargon and intricate processes. One such concept that programmers must understand is parameters – a key component of programming languages. In this article, we will explore what parameters are and how they are used in coding. We will also delve into the different types of parameters, working with parameters in different programming languages, and best practices for using parameters in code.
Defining Parameters in Programming
Before we dive deep into parameters, let’s start with the basics. Simply put, a parameter is a value that is passed into a function or method in programming. A function or method is a block of code that performs a specific task, and parameters allow for flexibility and customization by allowing developers to pass in different values of data to achieve different outcomes.
When a function or method is called, it expects certain values to be passed in as parameters. These values can be of different data types, such as integers, strings, booleans, floats, or arrays. By passing in different values of data as parameters, developers can achieve different outcomes from the same function or method. This is what makes parameters so powerful and essential in programming.
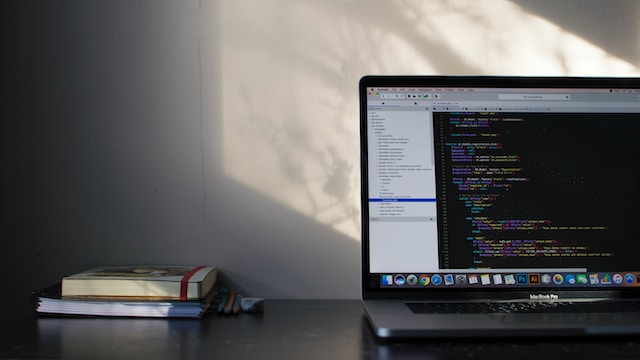
The Role of Parameters in Code
Parameters play a significant role in programming, enabling developers to create more efficient and effective code. By passing in parameters to functions and methods, coding can become more modular and reusable. With the right data types and values, parameters can make our code sharper, faster, and more precise.
For example, imagine you are creating a program that calculates the area of a circle. You could write a function that takes the radius of the circle as a parameter, and returns the area of the circle. By passing in different values for the radius, you can calculate the area of circles of different sizes, without having to write a separate function for each size.
Types of Parameters
There are several types of parameters that programmers use. The most common ones are:
- Integer Parameters: These are parameters that expect integer values, such as 1, 2, 3, etc;
- String Parameters: These are parameters that expect string values, such as “hello”, “world”, etc;
- Boolean Parameters: These are parameters that expect boolean values, such as true or false;
- Float Parameters: These are parameters that expect floating-point values, such as 1.2, 3.14, etc;
- Array Parameters: These are parameters that expect arrays, which are collections of data, such as [1, 2, 3], [“apple”, “banana”, “orange”], etc.
Parameter vs. Argument: What’s the Difference?
It is important to understand the difference between a parameter and an argument. A parameter is the value that is expected when a function or method is defined, while an argument is the actual value that is passed to the function or method. They are not the same, but they are often used interchangeably.
For example, if you have a function that calculates the sum of two numbers, the function might look like this:
function calculateSum(num1, num2) { return num1 + num2;}
In this function, num1 and num2 are the parameters. When you call the function and pass in actual values for num1 and num2, such as:
calculateSum(2, 3);
2 and 3 are the arguments that are passed to the function. The function then returns the sum of 2 and 3, which is 5.
Understanding the difference between parameters and arguments is essential for writing effective and efficient code.
Importance of Parameters in Coding
Now that we have covered the basics of parameters let’s move on to their importance in programming.
Enhancing Code Reusability
By using parameters, developers can write more modular and reusable code. Rather than creating separate functions for every possible use case, we can create one function and pass different parameters to achieve different results. This makes the code more efficient, easily maintainable, and saves time in development.
For example, let’s say we want to create a function that calculates the area of a rectangle. Instead of creating a separate function for every possible rectangle, we can create one function and pass in the length and width as parameters. This way, we can reuse the same function for any rectangle we want to calculate the area of.
Similarly, we can create a function that sorts an array of numbers. Instead of creating separate functions for sorting ascending and descending, we can create one function and pass in a parameter that specifies the sorting order.
Improving Code Readability
Well-defined parameters make code easier to read and understand. When a function or method is called with named parameters, it becomes much clearer what each parameter represents and what the code is supposed to do. By using descriptive parameter names, code is easier to comprehend, and developers can write more efficient code with less overall effort.
For example, let’s say we have a function that calculates the distance between two points. By using descriptive parameter names such as “x1”, “y1”, “x2”, and “y2”, it becomes much easier to understand what the function is doing and what each parameter represents.
Facilitating Code Maintenance
With the use of parameters, debugging and maintaining code becomes much easier. When errors occur, valuable information can be gathered from the parameters to determine which code block or function is causing the problem. This process also makes it easier to update code or add new features without interfering with the existing functions or methods.
For example, let’s say we have a function that calculates the average of an array of numbers. If we later decide to add a feature that calculates the median, we can simply add a new parameter to the existing function instead of creating a new function from scratch. This saves time and effort and makes the code more maintainable.
In conclusion, the use of parameters in coding is essential for creating efficient, maintainable, and readable code. By using parameters, developers can write more modular and reusable code, improve code readability, and facilitate code maintenance.
Working with Parameters in Different Programming Languages
When it comes to programming, parameters are an essential component of functions. They allow you to pass in data to a function and manipulate it as needed. Understanding how parameters work in different programming languages is crucial for any programmer.
Let’s explore how parameters work in some of the most popular programming languages:
Parameters in Python
Python is a popular programming language that is known for its simplicity and ease of use. In Python, parameters are defined using the def statement to define functions. The parameters are listed inside the parenthesis following the function name. They can be passed in as required, with the syntax of the function name followed by parentheses containing the parameters, separated by commas.
Python also allows for default parameter values, which means that if a parameter is not passed in, it will use the default value. This can be useful for setting up optional parameters.
Parameters in Java
Java is a widely-used programming language that is known for its robustness and reliability. In Java, parameters are passed in as input to a method using the method’s parenthesis. Similar to Python, they are listed inside the parenthesis and separated by commas.
Java also allows for method overloading, which means that you can have multiple methods with the same name but different parameters. This can be useful for creating more flexible code.
Parameters in JavaScript
JavaScript is a programming language that is used primarily for web development. It uses functions to perform tasks, and parameters are passed in as input to those functions. They are defined in the same way as Java and Python, within the parentheses of the function’s definition.
JavaScript also allows for anonymous functions, which means that you can define a function without giving it a name. This can be useful for creating more concise code.
Parameters in C++
C++ is a powerful programming language that is used for a wide range of applications, including operating systems, game development, and more. Like many other programming languages, C++ defines parameters using the function’s name and its parameters inside the parenthesis. Parameters in C++ can also include references or pointers as a way to manipulate the original data.
C++ also allows for function templates, which means that you can create a generic function that can work with multiple data types. This can be useful for creating more flexible code that can be used in a variety of situations.
Best Practices for Using Parameters in Code
Finally, let’s explore some best practices when it comes to using parameters in code.
Choosing Descriptive Parameter Names
When defining parameters, it is important to use descriptive and meaningful names. Doing so will make the code easier to read and follow, and it will also help avoid any confusion when working with fellow developers or updating the code.
Limiting the Number of Parameters
It is good practice to limit the number of parameters used in a function or method. Too many parameters can lead to confusion and make the code harder to understand. Instead, try to think of ways to simplify and optimize functions, keeping them organized and efficient.
Using Default Parameter Values
Default parameter values provide a way to offer an optional parameter that can be set when a value is not provided. This technique can be useful when there is a common or typical usage, such as setting a default value of zero or an empty string.
Conclusion
Parameters are a critical part of programming languages that enable developers to write efficient, maintainable, and reusable code. In this article, we have explored what parameters are, different types of parameters, their importance, how to work with them in different programming languages, and best practices to keep in mind when using them. By understanding parameters’ concepts, we can write more refined and effective code that fulfills the needs of our projects. With this knowledge, you are ready to create functional and efficient code that meets your needs.