Are you intrigued by the world of Arduino and its endless possibilities for building and tinkering with electronics? An open-source electronics platform, has gained immense popularity among hobbyists, educators, and professionals alike. While the hardware itself is a crucial component, the language plays an equally significant role in bringing your ideas to life. In this comprehensive guide, we will delve into the language, its unique characteristics, and its relationship with other programming languages. Whether you are a beginner or an experienced coder, this article will equip you with the knowledge you need to confidently write code for Arduino projects.
What is the Arduino Language?
The language is specifically designed to simplify the process of programming microcontrollers and make it more accessible to beginners and non-experts in the field of software development. It is a high-level programming language based on C++ with some modifications and simplifications to accommodate the unique requirements of the Arduino platform.
One of the primary goals of the language is to shield users from the complexities of low-level programming, allowing them to focus on the core functionality of their projects. It provides a straightforward syntax that is easy to read and understand, even for those who have little to no prior coding experience.
Arduino’s language abstraction simplifies tasks such as reading sensor values, controlling actuators, and communicating with other devices. This abstraction layer eliminates the need for intricate knowledge of the underlying hardware, making it easier to prototype and develop projects quickly.
The language provides a set of built-in functions and libraries that allow users to interact with the various components and peripherals of an Arduino board. These functions handle low-level operations, such as configuring pins, generating PWM signals, and communicating with serial devices, thus saving users from writing complex code from scratch.
By adopting the language, users can focus on the logic and behavior of their projects, rather than spending excessive time on low-level hardware details. This approach promotes rapid prototyping, iteration, and experimentation, enabling users to bring their ideas to life quickly and easily.
It’s important to note that although the language simplifies certain aspects of programming, it still retains the core concepts and syntax of C++. This means that if you are familiar with C++ programming, you can leverage your existing knowledge and skills to write code. Additionally, Arduino’s compatibility with C++ allows users to incorporate more advanced programming techniques and libraries when necessary, giving them the flexibility to tackle complex projects as their skills progress.
In the next sections, we will explore some of the key aspects of the coding, including its limitations, support for object-oriented programming, utilization of the Arduino.h library, setup and loop functions, variable initialization, automatic prototype generation, specific types, folder organization, and alternative IDE options.
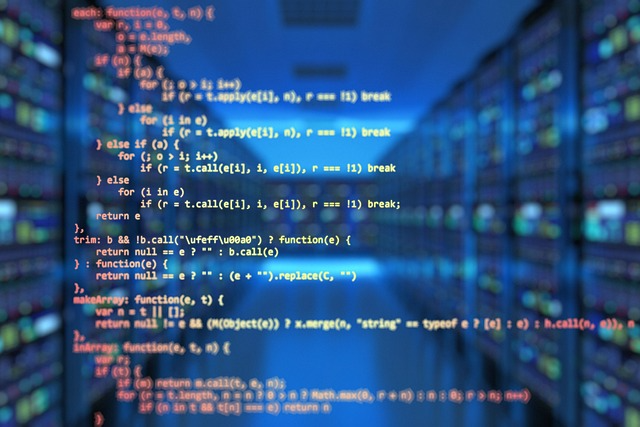
C++ Limitations
While the based on C++, it does have certain limitations compared to the full-fledged C++ language. These limitations are intentional and aimed at maintaining a user-friendly and simplified programming experience for users.
Here are some of the notable limitations:
Memory Constraints: Arduino boards typically have limited amounts of memory, both in terms of RAM and program storage (flash memory). This means that you need to be mindful of memory usage when writing code. The limited memory capacity restricts the use of large data structures or extensive dynamic memory allocation.
Lack of Standard C++ Libraries: This does not include the full set of standard C++ libraries that you would find in traditional C++ development environments. This omission is again to keep the code size and memory usage minimal. However, many commonly used functions and libraries are available through the Arduino Standard Library and other third-party libraries specifically developed.
No Exception Handling: Exception handling, a powerful feature in C++, is not available in the code. This means that you cannot use try-catch blocks to handle and recover from runtime errors or exceptions. Instead, you must rely on error checking and conditionals to handle potential issues in your code.
Limited Language Features: The Arduino language is stripped down to include only the most essential features and syntax elements required for programming microcontrollers. Certain advanced C++ language features, such as function overloading or templates, are not available in. However, these limitations are typically not a hindrance for most projects, as the simplified language covers the majority of use cases.
It’s important to note that despite these limitations, they remain powerful and capable of handling a wide range of projects. The focus on simplicity and accessibility makes it an ideal choice for beginners and hobbyists, allowing them to quickly grasp the fundamentals of programming and start building their own electronic creations.
In the following sections, we will explore how the supports object-oriented programming, the role of the Arduino.h library, the structure and functions of the setup and loop, variable initialization, automatic prototype generation, specific types, folder organization, and alternative IDE options to write code with the language. These aspects will further enhance your understanding of the Arduino language and its capabilities.
Object-Oriented Programming with Arduino Language
Arduino supports object-oriented programming (OOP) principles, allowing you to create reusable and modular code structures for your projects. While the Arduino language simplifies certain aspects of OOP compared to traditional C++, you can still apply fundamental concepts to enhance your code organization and readability.
Here’s what you need to know about object-oriented programming with the Arduino language:
- Classes and Objects: In the Arduino language, you can define your own classes to encapsulate data and behavior into objects. A class serves as a blueprint for creating objects with shared characteristics and functionality. By defining classes, you can organize your code into logical units and create multiple instances (objects) of those classes to represent different components or entities in your project;
- Encapsulation: Encapsulation is a key principle of OOP, and Arduino facilitates encapsulation by allowing you to define private and public members within a class. Private members are accessible only within the class itself, while public members can be accessed from outside the class. This helps in hiding implementation details and exposing only the necessary functionality, improving code maintainability and reusability;
- Abstraction: Abstraction allows you to create simplified interfaces for interacting with complex functionalities. In Arduino, you can define public methods within a class that provide a high-level interface to interact with the underlying hardware or perform specific tasks. This abstraction layer shields the user from complex low-level operations, making the code more readable and modular;
- Inheritance: Inheritance is the mechanism through which one class can inherit properties and behaviors from another class. While Arduino’s language simplifications do not support full-fledged inheritance as in C++, you can still achieve code reuse and modularity through code organization. By defining base classes with shared functionalities, you can create derived classes that inherit and extend those functionalities for specific purposes;
- Polymorphism: Polymorphism allows objects of different classes to be treated as instances of a common base class. In Arduino, while the language simplifications do not support polymorphism in the traditional sense, you can still achieve polymorphic behavior by using function overloading and function overriding. This allows you to write functions with the same name but different parameters or behaviors, providing flexibility in how you interact with objects.
By utilizing the principles of object-oriented programming, you can create well-structured, modular, and reusable code for your Arduino projects. This approach enhances code readability, simplifies maintenance and updates, and promotes efficient development practices. Embrace the power of object-oriented programming with the Arduino language to take your projects to the next level.
The Arduino.h Library
The Arduino.h library plays a crucial role in Arduino programming, providing a set of functions and definitions that facilitate interaction with the hardware components of an Arduino board. When you write Arduino code, including the Arduino.h library in your sketch grants you access to a wide range of pre-defined functions and constants.
Here’s what you need to know about the Arduino.h library:
Pin Modes and Operations: The Arduino.h library defines constants such as INPUT, OUTPUT, and INPUT_PULLUP, which are used to set the mode of digital pins. These constants make it easy to configure pins for reading inputs or driving outputs. The library also provides functions like pinMode(), digitalWrite(), and digitalRead() to control the state of digital pins.
Analog Input and Output: Arduino boards often have analog input and output capabilities. The Arduino.h library includes functions such as analogRead() and analogWrite() to read analog input values and generate analog output signals using pulse width modulation (PWM).
Serial Communication: The Arduino.h library supports serial communication, allowing you to send and receive data between an Arduino board and other devices. It provides functions like Serial.begin(), Serial.print(), and Serial.read() for configuring the serial communication settings and transmitting and receiving data over the serial port.
Timing and Delays: The Arduino.h library includes functions such as delay(), delayMicroseconds(), and millis() for controlling timing and creating delays in your code. These functions are useful for tasks like creating precise timing intervals, implementing time-based behaviors, and synchronizing actions with external events.
Math and Utility Functions: The Arduino.h library offers various math and utility functions that simplify common operations. Functions like min(), max(), constrain(), map(), and random() help you manipulate and process data efficiently.
Interrupts: Arduino boards support interrupts, which allow you to respond to external events in a timely manner. The Arduino.h library provides functions and macros for configuring and handling interrupts, enabling you to create responsive and event-driven code.
Advanced Features: The Arduino.h library also includes advanced features like EEPROM handling functions for reading and writing data to the board’s non-volatile memory, tone() and noTone() functions for generating audio tones, and more.
By including the Arduino.h library in your code, you can leverage its rich set of functions and definitions to interact with the hardware components of an Arduino board. It simplifies the process of controlling pins, reading sensors, communicating with other devices, and performing various tasks essential to your project. Familiarize yourself with the functions provided by the Arduino.h library to make the most out of the Arduino platform’s capabilities.
In the following sections, we will explore the structure and functions of the setup and loop, variable initialization, automatic prototype generation, specific Arduino types, folder organization, and alternative IDE options to write code with the Arduino language. These aspects will further enhance your understanding of Arduino programming and empower you to create even more complex and sophisticated projects.
Setup and Loop Functions
In Arduino programming, the setup() and loop() functions play vital roles in the execution of your code. Understanding how these functions work is essential for developing Arduino projects.
Here’s an overview of the setup and loop functions:
setup(): The setup() function is called only once when the Arduino board is powered on or reset. It is typically used to initialize variables, configure pin modes, and perform any necessary setup tasks. This function sets the initial conditions for your project before the main loop starts executing.
loop(): The loop() function is where the main execution of your code takes place. Once the setup() function completes, the Arduino enters an infinite loop, repeatedly executing the code within the loop() function. It is here that you define the core logic and behavior of your project. The loop() function continues running until the Arduino board is powered off or reset.
By dividing your code into the setup() and loop() functions, you can separate the initialization tasks from the main execution flow. This modular structure simplifies code organization and allows for easier modification and debugging. The setup() function ensures that your project starts in a known state, while the loop() function provides a continuous cycle for your code to run.
Variable Initialization with the Arduino Language
In Arduino programming, proper variable initialization is essential for maintaining predictable and reliable behavior. When declaring variables in Arduino, it’s good practice to assign initial values to them. Here’s why variable initialization is important:
Avoiding Garbage Values: When you declare a variable without initializing it, it can contain random values present in the memory at that time. These random values are often referred to as “garbage values” and can lead to unexpected behavior and bugs in your code. By initializing variables, you ensure that they start with known and intended values.
Consistent Behavior: Initializing variables to specific values helps ensure that your code behaves consistently across different runs and environments. It eliminates ambiguity and provides a reliable starting point for your calculations and comparisons.
Clarity and Readability: Initializing variables with meaningful values makes your code more readable and self-explanatory. It communicates the purpose and intended usage of the variable, making it easier for others (including your future self) to understand the code.
To initialize variables in the Arduino language, you can assign them values at the point of declaration or use the assignment operator (=) in the setup() function. By establishing initial values for your variables, you promote code reliability, clarity, and maintainability.
Automatic Prototype Generation
One of the convenient features of the Arduino language is the automatic prototype generation for functions. In Arduino, you can define function prototypes, which provide a forward declaration of a function’s name, return type, and parameters. The Arduino compiler automatically generates function prototypes for all functions defined in your code, allowing you to call functions before their actual definition. Here are the benefits of automatic prototype generation:
Flexible Function Order: With automatic prototype generation, you can define functions in any order in your code. This flexibility allows you to structure your code logically, placing functions based on their intended usage or dependencies.
Function Call Flexibility: The automatic prototypes enable you to call functions from anywhere in your code, even before their actual definition. This can be helpful when you have functions that call each other or have interdependencies.
Simplified Code Structure: Automatic prototype generation eliminates the need to manually declare function prototypes, reducing the amount of code you have to write and maintain. It streamlines the overall code structure and makes it easier to manage and update.
Boolean, Byte, and Other Specific Arduino Types
The Arduino language introduces specific data types that are commonly used in electronics projects. These types provide a way to represent and manipulate specific values efficiently.
Here are some of the specific data types available in the Arduino language:
Boolean: The boolean data type can have two possible values: true or false. Booleans are often used to represent logical conditions or flags in Arduino programming, allowing you to control the flow of your code based on certain conditions.
Byte: The byte data type represents an 8-bit value, ranging from 0 to 255. Bytes are frequently used for storing or transmitting data in its raw binary form. They are particularly useful when working with low-level operations or when dealing with serial communication and I2C protocols.
Unsigned Integers: Arduino supports various unsigned integer types, such as uint8_t, uint16_t, and uint32_t, representing 8-bit, 16-bit, and 32-bit unsigned integers, respectively. These types allow you to work with positive numbers only, effectively doubling the range of values you can represent compared to signed integers.
Arrays: Arrays are a collection of values of the same type, grouped together under a single variable name. They allow you to store and manipulate multiple values efficiently. Arrays in Arduino can be used to store sensor readings, LED patterns, or any other set of related data.
Strings: The Arduino String data type allows you to work with text and manipulate strings of characters. Strings provide a convenient way to store and manipulate textual information in your Arduino projects.
By leveraging these specific data types, you can optimize memory usage, improve code readability, and work with different types of data efficiently in your Arduino projects.
Folder Organization
Organizing your Arduino project files into folders can greatly enhance code management and project structure. While Arduino IDE does not enforce a specific folder structure, creating a logical organization can make your code easier to navigate and maintain. Here are some suggested folders you can create in your Arduino project:
Source Code: The source code folder is the main directory where you store your Arduino sketch (.ino) files. This folder should contain the primary code files for your project.
Libraries: If you are using external libraries in your project, it’s beneficial to create a separate folder to store those libraries. This ensures that your project remains organized and allows for easy library management.
Documentation: Keeping a documentation folder can be helpful for storing any relevant project documentation, including circuit diagrams, datasheets, project notes, or README files. This folder acts as a centralized repository for all project-related information.
Examples: If you have different variations or examples of your project code, consider creating an “examples” folder. This allows you to keep multiple versions or variations of your code for reference or testing purposes.
Resources: In the resources folder, you can store any additional resources needed for your project, such as images, sound files, or configuration files.
Remember, folder organization is subjective and can vary based on the complexity and requirements of your project. The key is to establish a structure that makes sense to you and facilitates easy navigation and maintenance of your codebase.
Using Alternative IDEs to Write Code with the Arduino Language
While the Arduino IDE (Integrated Development Environment) is the official and widely used platform for Arduino programming, there are alternative IDEs available that offer additional features and enhancements. These alternative IDEs can provide a more robust and personalized coding experience. Here are a few notable alternative IDEs for Arduino:
Visual Studio Code (VS Code) with PlatformIO: VS Code is a popular code editor known for its versatility and extensive plugin ecosystem. When combined with the PlatformIO plugin, it becomes a powerful development environment for Arduino and other microcontrollers. PlatformIO offers advanced features like code autocompletion, integrated library management, and debugging capabilities.
Eclipse Arduino IDE: Eclipse is a well-established and highly customizable IDE widely used in the software development community. The Eclipse Arduino IDE plugin extends the Eclipse environment to support Arduino development. It provides features like code navigation, project management, and a rich set of debugging tools.
Atom with Arduino IDE: Atom is a lightweight, open-source text editor that can be customized to suit your preferences. With the Arduino IDE plugin, you can transform Atom into a capable Arduino development environment. The plugin offers features like automatic code completion, built-in serial monitor, and easy integration with the Arduino ecosystem.
Visual Micro for Visual Studio: Visual Micro is a plugin that integrates Arduino development into Microsoft Visual Studio. It provides a familiar development environment for those already comfortable with Visual Studio. The plugin supports code highlighting, IntelliSense, and advanced debugging features.
By exploring alternative IDEs, you can find the one that aligns best with your workflow and offers features that enhance your Arduino programming experience. While the Arduino IDE remains a reliable choice, these alternatives provide additional functionalities and customization options to suit your specific needs.
Conclusion
In conclusion, the Arduino language, based on a simplified version of C++, offers a versatile and accessible platform for coding Arduino projects. Understanding the Arduino language allows you to utilize the vast array of libraries, functions, and data types available to interact with hardware components effectively.
Object-oriented programming principles can be applied in Arduino, enabling modular and reusable code structures. The Arduino.h library provides a comprehensive set of functions for interacting with the Arduino board’s hardware, simplifying tasks such as pin control, analog input/output, serial communication, timing, and more.
Proper usage of the setup() and loop() functions ensures the initialization and continuous execution of your code. Variable initialization, automatic prototype generation, specific Arduino types, folder organization, and alternative IDEs further enhance your Arduino programming experience.
By mastering the Arduino language and employing best practices, you can unlock the full potential of the Arduino platform, creating innovative projects and bringing your ideas to life.