In the realm of C# programming, when dealing with textual data within our applications, it’s not uncommon to encounter the need for data preprocessing. A common task involves refining the integrity of our data by removing unwanted characters from strings. Exploring one avenue to accomplish this within the C# framework is our objective.
Dive Deeper into C# Trim(): Exploring its Key Features and Usage Tips
The C# Trim() string method brings unique capabilities for string manipulation at your fingertips. Here is a detailed look into some distinctive features of Trim() and how you can utilize them in your programming:
The Core Aspects of Trim()
- Non-destructive Process: The Trim() method provides you a revised version of the original string and does not modify the source string;
- Intelligent Character Trimming: The power of Trim() lies in its intelligent trimming mechanism. The method starts eradicating characters from the edges until it encounters a character outside its defined cutting set. For instance, the invocation of Trim(‘a’, ‘z’) will cease its action once it comes across a character other than ‘a’ or ‘b’;
- Return of Original String: If no character qualifies for trimming, Trim() returns the original, unaltered string;
- Whitespace Removal: By default, Trim() without any argument expunges whitespaces from both ends of the string;
- Enduring Feature of C#: Trim() is a long-standing feature in C#, making its first appearance in .NET Framework 1.1, .NET Core 1.0, and .NET Standard 1.0.
Key Considerations When Using Trim()
While using the Trim() method, consider the following points to ensure accuracy:
- Case Sensitivity: The characters you specify are case-sensitive. For example, Trim(‘h’) will not function similarly to Trim(‘H’);
- Order-Independent Character Removal: The method eliminates characters regardless of their order. So, using Trim(‘x’, ‘y’) will have the same effect as Trim(‘y’, ‘x’). If you need to eliminate specific character patterns, consider employing C#’s Replace() string method;
- Independent Trimming: Trim() operates independently on each side of the string. It halts when it encounters a character at one end of the string that is not part of the cut set. Hence, the process is not symmetric: it could potentially eliminate 3 characters from the start of the string but 475 from the end;
- Handling of Empty and Null Strings: When executing Trim() on an empty string (“”), it returns an empty string. However, applying Trim() on a null string results in a NullReferenceException exception.
By understanding these intricacies, you will be better equipped to utilize the full potential of C# Trim() and ensure clean, clear, and effective string manipulations in your programming endeavours.
Unleashing Differentiating Features of C# TrimStart()
The power of C# TrimStart() lies in its precise string manipulation functions. It offers two distinguished methods to remove characters, specifically from the beginning of any string.
Tackling Extra Whitespaces with TrimStart()
Firstly, the conventional TrimStart() method targets the elimination of excessive whitespaces, which are often overlooked during data input but can hamper the readability of the code. Here’s an example:
string phrase = " Let's Kodify!";
string trimmedPhrase = phrase.TrimStart();
// Outcome: "Let's Kodify!"
The TrimStart() method accurately identifies and removes all leading whitespace characters, resulting in a neatly formatted string.
Fine-Tuning Character Omission with TrimStart(char[])
The second usage of TrimStart() takes a more customized approach, allowing developers to specify certain Unicode characters to be removed from the beginning of a string.
string phrase = " Let's Kodify!";
string trimmedPhrase = phrase.TrimStart(' ', 'L');
// Outcome: "et's Kodify!"
In the above example, TrimStart(’ ‘, ‘L’) is applied to eliminate leading spaces and the letter ‘L’. The result is a modified string that begins directly with “et’s Kodify!”
Key Pointers for Utilizing TrimStart()
While using the TrimStart() method, consider the following aspects:
- It is case-sensitive, which means that arguments within TrimStart() need to match the exact format of the string characters to be removed;
- The execution sequence of characters in TrimStart() is irrelevant. Whether you write TrimStart(‘x’, ‘y’) or TrimStart(‘y’, ‘x’), the output remains the same;
- TrimStart() only removes characters from the start of a string, irrespective of their occurrence in the rest of the string;
- If no argument is provided, TrimStart() focuses on removing leading whitespace characters.
With these insights into C# TrimStart(), you can enhance your code’s readability and clarity, ultimately leading to better software quality and maintainability.
Exploring the Aptitude of C# TrimEnd() and Its Varied Usage
Diving further into the world of C# string manipulations, we encounter the precise and effective TrimEnd() function. Like its siblings, Trim() and TrimStart(), the application of the C# TrimEnd() method can enhance your strings and the overall clarity of your code.
Using TrimEnd() to Eliminate Trailing Whitespaces
Firstly, the TrimEnd() method is superbly equipped to strip unnecessary whitespace characters from the end of a string. Let’s understand this with a coding snippet:
string phrase = "Let's Kodify! ";
string trimmedPhrase = phrase.TrimEnd();
// Result: "Let's Kodify!"
In this instance, invoking TrimEnd() on the string ‘phrase’ wipes out all trailing whitespace characters, leaving us with a clean, legible string.
Tailoring Character Removal with TrimEnd(char[])
Besides the conventional function, TrimEnd() also offers a more personalized mechanism for precise character removal. The method allows an array of specified characters to be trimmed just from the string’s end.
string phrase = "Let's Kodify!...";
string trimmedPhrase = phrase.TrimEnd('.', ' ');
// Result: "Let's Kodify!"
Here, TrimEnd(‘.’, ’ ‘) is used to remove trailing periods and spaces. The end product is a more refined and uncluttered string.
Valuable Insights while Using TrimEnd()
When implementing the TrimEnd() method, the following considerations might enhance your coding precision:
- Remember that the method is case-sensitive, ensuring the accurate match of characters;
- The sequence of characters in TrimEnd() doesn’t influence the outcome. The functions TrimEnd(‘a’, ‘b’) and TrimEnd(‘b’, ‘a’) hold the same effect;
- TrimEnd() specifically works on the string’s end, leaving the rest of the string intact;
- Without any argument, it eliminates trailing whitespaces.
By harnessing these key qualities of C# TrimEnd(), developers can elevate their control over string manipulations, streamline their code, and foster software of exceptional quality.
Enhancing Text Trimming with the TrimEnd() Method
When it comes to refining strings in your code, the TrimEnd() method stands out as a versatile tool. It’s like giving your strings a well-deserved makeover, removing any excess characters that might be hanging around at the end. Let’s delve deeper into the nuances of this method and explore its two distinct modes of operation:
1. Trimming Whitespace:
Whitespace characters like spaces, tabs, and newlines often lurk at the end of strings, cluttering your data and causing potential issues. The TrimEnd() method comes to the rescue by effortlessly whisking away these unwanted characters, leaving your string looking pristine.
Example:
string original = "Hello, World! ";
string trimmed = original.TrimEnd();
// Result: "Hello, World!"
Tips for Trimming Whitespace:
- Always trim user input before processing to ensure data integrity;
- Use TrimEnd() in conjunction with other string manipulation methods for comprehensive data cleaning;
- Remember that whitespace includes more than just spaces; be mindful of tabs, newlines, and other non-printable characters.
2. Custom Trimming with Character Arrays:
But wait, there’s more! The TrimEnd() method goes beyond basic whitespace removal. With a simple tweak, you can customize it to trim specific characters, catering to your unique trimming needs. Simply provide an array of characters you want to bid farewell, and TrimEnd() will handle the rest.
Example:
string original = "Hello, World! ";
string trimmed = original.TrimEnd(' ', '!');
// Result: "Hello, World"
Exploring the Versatility of TrimEnd() Method
TrimEnd(), a method entrenched in the arsenal of C# programmers, offers a spectrum of features that render string manipulation a breeze. Delve into its nuances and unleash its potential to optimize your code.
- String Transformation without Alteration: The hallmark trait of TrimEnd() lies in its non-destructive nature. It leaves the original string untouched while furnishing a modified rendition. This ensures data integrity and preserves the integrity of the source string;
- Dynamic Character Trimming: Embark on a journey of dynamic string tailoring with TrimEnd(). By specifying a set of characters, this method adeptly prunes the string until encountering a character beyond the defined set. For instance, TrimEnd(‘o’, ‘p’) meticulously shears characters until diverging from ‘o’ or ‘p’, offering precision in string refinement;
- Intelligent Handling of Empty Strings: TrimEnd() is astute in its treatment of empty strings. When the string is devoid of trailing characters for removal, it dutifully returns the source string unaltered. This pragmatic approach streamlines code execution and eliminates redundant operations;
- Whitespace Removal: A quintessential utility of TrimEnd() emerges when dealing with whitespace. Without specifying any arguments, it proficiently excises all trailing whitespace from the string. This simplifies data processing and enhances readability by eliminating extraneous spaces;
- Widely Compatible Across C# Ecosystem: TrimEnd() stands as a stalwart companion for C# programmers across various frameworks. From its inception in .NET Framework 1.1 to its ubiquity in .NET Core 1.0 and .NET Standard 1.0, its seamless integration empowers developers to harness its capabilities across diverse projects.
Exploring the Functionality of the TrimEnd() Method
Let’s delve into the practical application of the TrimEnd() method through an illustrative example program. This console application demonstrates how this string method effectively cleans the trailing characters from the right side of a string.
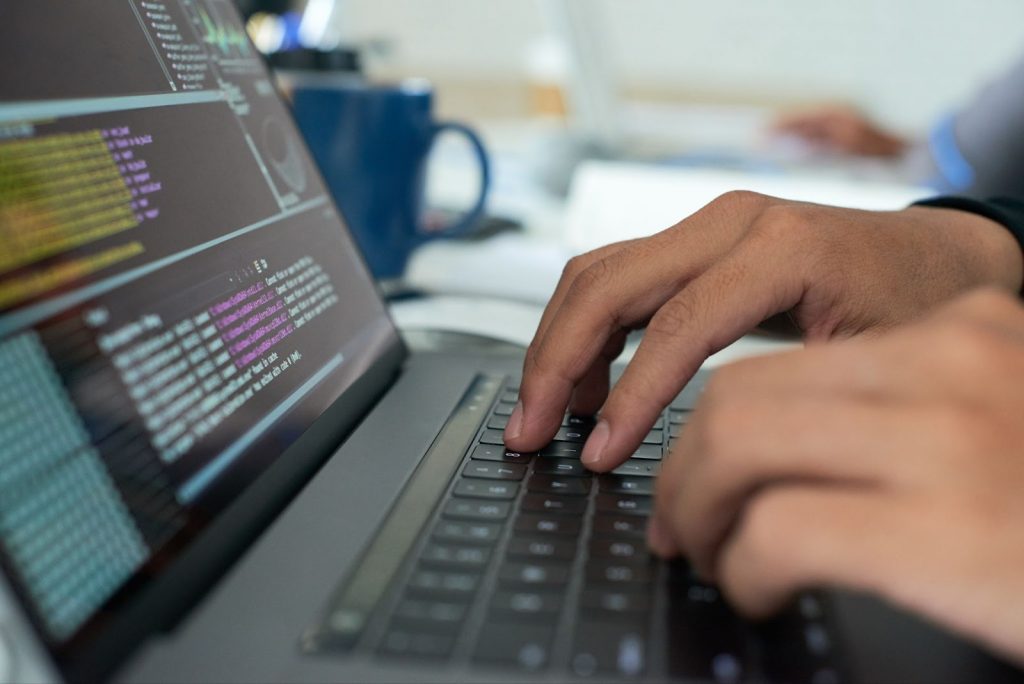
using System;
class TrimEndExample
{
static void Main()
{
// Define the example string with trailing characters to be trimmed
string example = ">> Hello, World! <<";
// Utilize TrimEnd() to remove specific characters from the end of the string
string exampleTrimmed = example.TrimEnd(' ', '!', '<');
// Display the original and trimmed strings
Console.WriteLine($"Original string: {example}");
Console.WriteLine($"Trimmed string: {exampleTrimmed}");
}
}
Understanding the Program Flow
In this program, we initiate by creating a string variable named “example,” which encapsulates the phrase “Hello, World!” enclosed within the symbols ‘>>’ and ‘<<‘. The aim is to eliminate trailing characters from the right end of this string.
Applying the TrimEnd() Method
The TrimEnd() method is invoked on the string instance “example” to facilitate the removal of specific characters from its trailing end. Within the parentheses of TrimEnd(), we specify three characters to be eliminated: a space (‘ ‘), an exclamation mark (‘!’), and a left angle bracket (‘<‘). Consequently, TrimEnd() systematically eliminates all occurrences of these characters from the end of the string. If the method encounters a character differing from those specified, it ceases trimming and returns the modified string.
Visualizing the Output
The program concludes with two Console.WriteLine() statements that portray the original string and the resultant trimmed string. Upon execution, the C# output provides a clear depiction:
Original string: >> Hello, World! <<
Trimmed string: >> Hello, World
Recommendations and Best Practices
- Parameter Selection: Choose the characters to trim wisely based on the specific requirements of your string manipulation task;
- Efficiency Consideration: Avoid unnecessary trimming by precisely identifying the characters needing removal;
- Testing and Validation: Verify the output against expected results to ensure the TrimEnd() method behaves as intended;
- Error Handling: Implement robust error handling mechanisms to address scenarios where unexpected characters are encountered.
Conclusion
In conclusion, mastering the art of data cleansing is pivotal for ensuring the reliability and efficiency of our C# programs. By understanding techniques such as trimming specific characters from strings, we enhance the robustness of our applications and streamline data processing workflows. With this knowledge at hand, we’re better equipped to navigate the intricacies of text manipulation in C#, empowering us to build more resilient and effective software solutions.
Add comment