Conditional statements are an essential part of programming languages, allowing developers to create different paths for the code to follow based on specific criteria. In C#, if-else statements are commonly used for decision making and branching. However, C# also offers a shorthand version of the if-else statement, known as the ternary operator. In this article, we will explore the C# if-else shorthand, also known as the ternary operator, its syntax, advantages, and use cases.
Introduction to C# Conditional Statements
C# is a popular programming language used to develop a wide range of applications, including desktop applications, video games, and web applications. One of the most essential features of any programming language is the ability to make decisions based on specific conditions. In C#, we use conditional statements to control the flow of the program, allowing the computer to make decisions about the execution of code based on specific criteria.
The Importance of Conditional Statements in Programming
Conditional statements are essential in programming because they help us create programs that can make decisions and respond to different scenarios. For example, if we were building a program that calculates the average grade of a student, we would need to use conditional statements to determine if the grade is passing or failing. This process allows us to create programs that can respond to different input values and provide appropriate output.
Basic Syntax of C# If Else Statements
The basic syntax of the C# if-else statement is as follows:
- if ( condition )
- {
- // Code to be executed if the condition is true
- }
- else
- {
- // Code to be executed if the condition is false
- }
In this syntax, the condition is a Boolean expression that evaluates to either true or false. If the condition is true, the code within the first set of curly braces is executed. If the condition is false, the code within the second set of curly braces is executed.
Conditional statements can be nested, allowing for more complex decision-making processes. For example, we can use nested if-else statements to determine if a student has passed a class based on their average grade and attendance record. This allows us to create programs that can handle a wide range of scenarios and provide appropriate output based on specific conditions.
Another important type of conditional statement in C# is the switch statement. The switch statement allows us to evaluate a single variable or expression and execute different blocks of code based on its value. This is useful when we have a large number of possible values and want to avoid writing multiple if-else statements.
Overall, conditional statements are a crucial part of programming in C# and other languages. They allow us to create programs that can make decisions and respond to different scenarios, making our applications more robust and versatile.
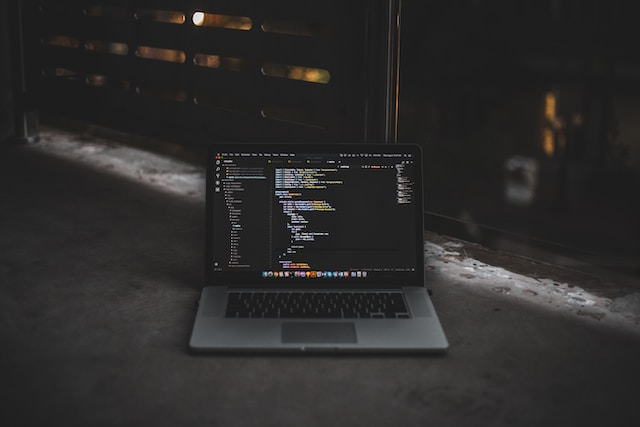
The Ternary Operator: C#’s If Else Shorthand
The ternary operator is a shorthand version of the if-else statement in C#. It allows us to write conditional expressions in a more concise way, reducing the amount of code we need to write. The ternary operator is particularly useful when we need to assign a value to a variable based on a condition.
However, it is important to use the ternary operator judiciously. Overuse of the ternary operator can make code harder to read and understand, especially for those who are new to the language. It is important to balance the benefits of concise code with the need for code that is easy to read and maintain.
Syntax of the Ternary Operator
The syntax of the ternary operator is as follows:
condition ? value_if_true : value_if_false;
In this syntax, the condition evaluates to a Boolean expression. If the condition is true, the value_if_true expression is returned. If the condition is false, the value_if_false expression is returned.
For example, consider the following code:
int x = 10;
string message = x > 5 ? "x is greater than 5" : "x is less than or equal to 5";
In this code, the condition x > 5 is evaluated. If it is true, the string “x is greater than 5” is assigned to the message variable. If it is false, the string “x is less than or equal to 5” is assigned to the message variable.
Advantages of Using the Ternary Operator
There are several advantages to using the ternary operator over traditional if-else statements:
- The ternary operator is more concise, reducing the amount of code we need to write.
- The ternary operator can make code easier to read, especially when used in small expressions.
- The ternary operator can be faster than if-else statements in some cases, as it requires fewer CPU cycles to execute.
For example, consider the following code:
int x = 10;
int y = 5;
int z = x > y ? x - y : y - x;
In this code, the condition x > y is evaluated. If it is true, the value of x – y is assigned to the z variable. If it is false, the value of y – x is assigned to the z variable.
Common Use Cases for the Ternary Operator
The ternary operator is commonly used in the following scenarios:
- Assigning a value to a variable based on a condition;
- Using the result of a condition to determine the return value of a method or function;
- Performing basic calculations based on conditions.
For example, consider the following code:
int x = 10;
int y = 5;
int z = x > y ? x - y : y - x;
Console.WriteLine("The absolute difference between {0} and {1} is {2}", x, y, z);
In this code, the absolute difference between x and y is calculated using the ternary operator and then printed to the console.
Comparing the Ternary Operator and Traditional If Else Statements
The ternary operator and traditional if-else statements are both conditional statements used in programming. They both serve the same purpose of controlling the flow of execution based on a condition. However, they differ in syntax, readability, and performance.
Readability and Clarity
One of the main advantages of traditional if-else statements is that they are often more explicit and easier to read and understand. The syntax of the ternary operator can become difficult to read and understand when used in complex expressions. This is because the ternary operator condenses the if-else statement into a single line of code, making it harder to follow.
For instance, consider the following code:
result = (condition) ? value1 : value2;
This code can be difficult to understand, especially if the condition, value1, and value2 are complex expressions. In contrast, the same code written using a traditional if-else statement would be more explicit:
if (condition) { result = value1;} else { result = value2;}
Using traditional if-else statements can make the code more readable and easier to understand, especially for developers who are not familiar with the ternary operator.
Performance Considerations
Another advantage of the ternary operator is that it can be faster than traditional if-else statements, especially when used in small expressions. This is because the ternary operator is a single expression, whereas a traditional if-else statement is a block of code that requires branching.
However, if the expression is complex or the condition is evaluated repeatedly, the difference in performance between the ternary operator and traditional if-else statements may become negligible. In such cases, it is essential to consider the readability and maintainability of the code as well.
Situations Where One Method is Preferred Over the Other
When deciding between the ternary operator and traditional if-else statements, it is essential to consider the specific requirements of the program and the context in which the expression will be used. In general, the ternary operator is better suited for simple, concise expressions, while traditional if-else statements may be more appropriate for more complex scenarios.
For instance, when checking if a value is null, the ternary operator can be used to assign a default value:
name = (name != null) ? name : "John Doe";
On the other hand, when there are multiple conditions to check or complex logic to implement, traditional if-else statements may be more appropriate:
if (condition1) { // code block} else if (condition2) { // code block} else { // code block}
Overall, both the ternary operator and traditional if-else statements have their advantages and disadvantages. It is up to the developer to decide which method is more appropriate for a given scenario based on the specific requirements of the program.
Tips for Using the Ternary Operator Effectively
When using the ternary operator, there are a few best practices to keep in mind:
The ternary operator is a powerful tool in the C# language that allows you to write concise and readable code. It’s a shorthand way of writing an if-else statement, and it can save you a lot of time and effort when used correctly.
Avoiding Nested Ternary Operators
While nesting ternary operators can save space, it can also make the expression difficult to read and understand. Whenever possible, it’s best to use traditional if-else statements for complex expressions. This can help to improve the readability and maintainability of your code in the long run.
For example, instead of writing:
int result = (a > b) ? ((a > c) ? a : c) : ((b > c) ? b : c);
You can write:
int result;if (a > b){ if (a > c) { result = a; } else { result = c; }}else{ if (b > c) { result = b; } else { result = c; }}
Ensuring Proper Parentheses Usage
When using the ternary operator, it’s important to use parentheses to ensure that the expression is evaluated correctly. This can help to prevent bugs and improve code clarity. For example:
int x = (a > b) ? (c + d) : (e + f);
Without the parentheses, the expression would be evaluated as:
int x = a > (b ? c + d : e) + f;
Which is not what we intended.
Combining Ternary Operators with Other C# Features
The ternary operator can be combined with other C# features, such as lambda expressions and null coalescing operators, to create more powerful and concise expressions. Understanding these features and how they interact with the ternary operator can help you write more efficient and effective code.
For example, you can use a lambda expression with the ternary operator to create a concise and readable code:
List<int> numbers = new List<int> { 1, 2, 3, 4, 5 };int sum = numbers.Aggregate((a, b) => a + b);
Here, the lambda expression (a, b) => a + b is used with the Aggregate method to calculate the sum of all the numbers in the list. This is much more concise than writing a loop to iterate over the list and calculate the sum.
Similarly, you can use the null coalescing operator with the ternary operator to handle null values:
string name = person?.Name ?? "Unknown";
Here, the null coalescing operator ?? is used with the ternary operator to assign the value of person.Name to name if it’s not null, or the string “Unknown” if it is null.
Conclusion
The C# ternary operator, or shorthand if-else statement, is a powerful tool that can help developers write more concise and efficient code. While it has several advantages over traditional if-else statements, it may not be the best choice for every scenario. By understanding the syntax, advantages, and use cases of the ternary operator, developers can make informed decisions about when and how to use this feature in their code.