Java, as a popular programming language, offers developers a powerful and flexible platform for building robust applications. However, understanding the concepts of compile time and runtime, as well as the errors associated with each, is crucial for writing efficient and bug-free code. In this article, we delve into the world of Java’s compile time and runtime errors, exploring their definitions, differences, and examples. By gaining a comprehensive understanding of these key concepts, you’ll be equipped to write code that performs optimally, detects errors early, and delivers a smooth user experience.
What is Compile Time in Java?
During the development of a Java program, the concept of compile time plays a vital role in the overall execution process. The compile time, also known as the compilation phase, is the initial stage where the Java source code is transformed into bytecode by the Java compiler.
At compile time, the Java compiler analyzes the source code, checking it for syntax errors and ensuring that it adheres to the rules of the Java programming language. The compiler performs various essential tasks, such as lexical analysis, syntax analysis, semantic analysis, and code generation.
Let’s take a closer look at each of these tasks:
- Lexical Analysis: This is the process of breaking down the source code into a series of tokens. Tokens can be keywords, identifiers, operators, literals, or separators. The lexer (part of the compiler) scans the source code character by character and generates a sequence of tokens;
- Syntax Analysis: Once the tokens are generated, the syntax analysis phase, also known as parsing, comes into play. This phase ensures that the sequence of tokens conforms to the grammatical rules defined by the Java programming language. If the code violates any grammar rules, syntax errors are reported;
- Semantic Analysis: In this phase, the compiler performs a deeper analysis of the code to ensure that the semantics, or meaning, of the program are correct. It checks for type compatibility, variable declarations, method invocations, and other language-specific rules. If any inconsistencies are detected, the compiler generates compile time errors;
- Code Generation: After successfully passing the previous stages, the compiler generates bytecode, which is a platform-independent representation of the program. Bytecode is stored in .class files and can be executed by any JVM-compatible environment.
During the compilation phase, the compiler provides detailed error messages and line numbers to help developers identify and rectify issues. It is essential to address all compile time errors before proceeding to the next phase, as unresolved errors will prevent the program from being executed.
By catching errors early in the development cycle, the compile time phase ensures that the program’s syntax, structure, and adherence to language rules are correct, leading to more reliable and efficient Java applications.
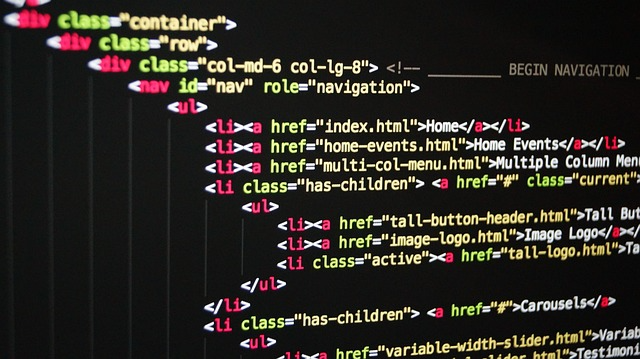
Understanding Compile Time Errors in Java
Compile time errors, also referred to as syntax errors, occur when the compiler encounters issues while translating the Java source code into bytecode. These errors prevent the successful compilation of the program, and as a result, the code cannot be executed until all compile time errors are resolved.
Let’s explore some common examples of compile time errors that developers often encounter:
- Missing Semicolon: One of the most basic and common compile time errors is forgetting to include a semicolon at the end of a statement. Java uses semicolons to separate statements, and omitting them leads to a syntax error;
- Undefined Variables: If a variable is used in the code without being declared or initialized, a compile time error occurs. The compiler cannot recognize the variable, resulting in an “undeclared variable” error;
- Type Mismatches: Java is a strongly-typed language, meaning variables must be assigned values of the correct data type. Assigning a value of one type to a variable of another incompatible type results in a compile time error. For example, assigning a string value to an integer variable will cause a type mismatch error;
- Incorrect Method Signatures: In Java, methods are defined by their signatures, which include the method name and the parameters it accepts. If a method is called with incorrect arguments or the wrong number of arguments, a compile time error occurs;
- Unreachable Code: This error occurs when there are code segments that can never be reached during program execution. It often happens when there is an unconditional return statement or an infinite loop before the code that follows;
- Improper Use of Reserved Keywords: Java has a set of reserved keywords that have predefined meanings within the language. If these keywords are used inappropriately, such as using “class” as a variable name, a compile time error will occur.
When a compile time error is encountered, the compiler provides detailed error messages that indicate the nature of the error, the line number where it occurred, and often suggestions for resolving the issue. These error messages act as valuable guidance for developers to identify and fix errors before executing the program.
It is important to note that the presence of compile time errors prevents the code from being compiled into bytecode and executed. Therefore, addressing these errors is crucial for the successful execution of a Java program. By carefully reviewing and rectifying compile time errors, developers can ensure that their code meets the language’s syntax and semantic requirements, resulting in a functional and error-free application.
What is Runtime in Java?
Once the Java source code has successfully compiled into bytecode, the runtime phase begins. During this phase, the Java Virtual Machine (JVM) executes the bytecode and manages the program’s execution. The runtime phase is where the program interacts with the user and performs its intended functionality.
Here are some key aspects of the runtime phase in Java:
- Java Virtual Machine (JVM): The JVM is a crucial component of the Java platform. It is responsible for executing the bytecode generated during the compilation phase. The JVM provides an environment in which Java programs can run independently of the underlying operating system and hardware. It performs several tasks during runtime, including memory management, garbage collection, and exception handling;
- Memory Management: One of the key responsibilities of the JVM during runtime is managing the program’s memory. It dynamically allocates and deallocates memory resources for objects created during program execution. The JVM uses various memory areas, such as the heap, stack, and method area, to store different types of data and ensure efficient memory utilization;
- Garbage Collection: Java incorporates automatic memory management through a process called garbage collection. The JVM periodically identifies and reclaims memory that is no longer in use by the program. It frees up memory occupied by objects that are no longer referenced, reducing memory leaks and manual memory management overhead;
- Exception Handling: During runtime, the JVM handles exceptions that occur within the program. Exceptions are unexpected events or error conditions that disrupt the normal flow of execution. The JVM employs a robust exception handling mechanism, allowing developers to catch and handle exceptions gracefully. Uncaught exceptions that are not handled by the program will cause the program to terminate with an error message;
- Program Execution: The runtime phase is where the program’s instructions are executed sequentially. The JVM interprets the bytecode or, in some cases, just-in-time (JIT) compiles it into machine code for faster execution. The program interacts with users, processes input and output operations, performs calculations, and executes algorithms as defined by the logic written in the source code.
Throughout the runtime phase, the JVM manages the underlying complexities of executing Java programs, providing an abstract and consistent execution environment across different platforms. It ensures that the program runs efficiently, handles resources appropriately, and responds to user interactions effectively.
Understanding the runtime phase is essential for diagnosing and resolving runtime errors, optimizing performance, and ensuring the smooth operation of Java applications. By leveraging the capabilities of the JVM and writing code that adheres to best practices, developers can create robust and reliable software solutions.
The Difference Between Runtime and Compile Time
The primary distinction between runtime and compile time lies in their respective phases and responsibilities within the Java execution process. Compile time refers to the initial translation of the source code into bytecode, which is carried out by the compiler. On the other hand, runtime encompasses the execution of the bytecode by the JVM, handling memory management, and executing the program’s instructions. It’s important to understand this difference to identify when and where errors occur in the development cycle.
Differentiating Compile Time Errors and Runtime Errors
Compile time errors and runtime errors differ not only in their occurrence during the development cycle but also in their impact on the program’s execution. As mentioned earlier, compile time errors prevent the program from successfully compiling, halting its execution until all errors are resolved. In contrast, runtime errors occur during the execution of the program when the JVM encounters an unexpected situation or error condition that it cannot handle. These errors are not caught by the compiler and may lead to program crashes, unexpected behavior, or incorrect results.
Conclusion:
In Java development, understanding the differences between compile time and runtime, as well as the errors associated with each, is crucial for producing reliable and efficient code. Compile time errors, occurring during the compilation phase, are detected and reported by the compiler, while runtime errors arise during the execution phase when the JVM encounters unforeseen issues. By thoroughly comprehending these concepts and their examples, developers can proactively identify and resolve errors, leading to more stable and robust Java applications. Remember, a thorough understanding of compile time and runtime intricacies can significantly enhance your programming skills and contribute to the overall quality of your Java projects.
Add comment