Python is a popular programming language used in a wide range of industries, including software development, data science, and web development. One of the many useful features that Python offers is the ability to truncate values. In this article, we will discuss what truncation is, why it is useful, and how to truncate in Python with step-by-step instructions.
Understanding Truncation in Python
Before we dive into the specifics of truncating in Python, let’s define what truncation is. Truncation is the process of removing the decimal portion of a number or cutting a string or list to a specified length. In Python, there are several ways to truncate values, including using the math.trunc() function, converting the value to an integer with the int() function, and implementing string slicing.
What is Truncation?
Truncation is a process of removing the decimal portion of a number or cutting a string or list to a specified length. It is a common operation in programming and is used to simplify data and make it easier to work with. For example, if you are working with financial data, you might want to remove the decimal portion of a number to perform integer division. This can help you avoid rounding errors and ensure that your calculations are accurate.
Truncation can also be used to cut a string or list to a specific length. This is useful when you are working with data that has a maximum length requirement, such as a password or username. By truncating the string or list to the required length, you can ensure that it meets the necessary criteria.
Why Truncate in Python?
There are several reasons why you might want to truncate values in Python. For example, you might want to remove the decimal portion of a number to perform integer division or to display the value as a whole number. This can be useful when working with financial data or when you need to display data in a specific format.
Truncation can also be used to cut a string or list to a specified length. This can make it easier to work with the data and ensure that it meets specific requirements. For example, if you are working with a password field, you might want to truncate the input to a specific length to ensure that it is not too long or too short.
In addition to these practical applications, truncation can also be used as a tool for data analysis and manipulation. By truncating data to specific lengths or removing decimal portions, you can simplify the data and make it easier to analyze. This can be particularly useful when working with large datasets or complex data structures.
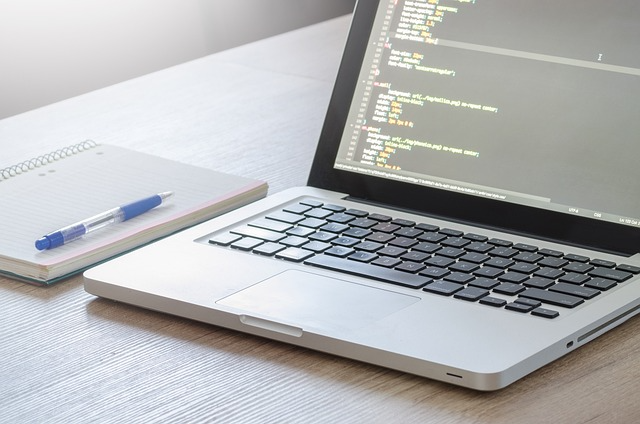
Setting Up Your Python Environment
Before we can start truncating in Python, we need to set up our Python environment. This involves installing Python and choosing a code editor. Setting up your Python environment is crucial for any Python project, as it ensures that you have all the necessary tools and software to work with Python efficiently.
Installing Python
If you don’t already have Python installed, you can download it from the official Python website at python.org/downloads/. Python is available for all major operating systems, including Windows, macOS, and Linux. Once you have downloaded the appropriate installer for your operating system, follow the installation instructions to install Python on your computer.
Python is a popular programming language that is widely used for web development, data analysis, machine learning, and more. Python has a large and active community of developers, who contribute to the language by creating libraries, frameworks, and tools that make Python even more powerful.
Choosing a Code Editor
There are many code editors to choose from, each with its own set of features and benefits. Some popular code editors for Python include Visual Studio Code, PyCharm, and Sublime Text. When choosing a code editor, consider factors such as ease of use, customization options, and support for Python-specific features.
Visual Studio Code is a free and open-source code editor that is developed by Microsoft. It has a wide range of features, including syntax highlighting, code completion, and debugging tools. Visual Studio Code also has a large and active community of developers, who create extensions and plugins to add even more functionality to the editor.
PyCharm is a popular code editor for Python that is developed by JetBrains. It is a full-featured IDE that includes features such as code analysis, debugging, and version control integration. PyCharm also has a range of plugins and extensions that can be used to customize the editor to your specific needs.
Sublime Text is a lightweight and fast code editor that is popular among Python developers. It has a simple and intuitive interface, and supports a wide range of programming languages, including Python. Sublime Text also has a range of plugins and extensions that can be used to add new features and functionality to the editor.
Ultimately, the choice of code editor comes down to personal preference. Try out a few different editors to see which one you like best, and don’t be afraid to experiment with different features and settings to find the setup that works best for you.
Python Truncation Methods
When working with data in Python, it is often necessary to truncate values to a certain number of decimal places or to a specific length. In this tutorial, we will explore three different methods for truncating values in Python.
Using the math.trunc() Function
The math.trunc() function is a built-in function in Python that returns the integer part of a number, discarding the decimal portion. This function can be useful when you need to remove the decimal portion of a number without rounding the value. Here’s an example:
import mathx = 3.14159truncated = math.trunc(x)print(truncated)
This code will output:
3
Note that the math.trunc() function will always return an integer, even if the input is negative. For example, if we pass in -3.14159, the function will return -3.
In addition to truncating values, the math module in Python provides a variety of other mathematical functions, such as square roots, logarithms, and trigonometric functions. These functions can be useful in a wide range of applications, from scientific computing to financial modeling.
Truncating with the int() Function
Another way to truncate values in Python is to convert the value to an integer using the int() function. This function will round the value towards zero, so if the input is positive, it will behave the same as the math.trunc() function. However, if the input is negative, the int() function will round the value towards negative infinity. Here’s an example:
x = 3.14159truncated = int(x)print(truncated)
This code will output:
3
Like the math.trunc() function, the int() function will return an integer, even if the input is negative. However, unlike math.trunc(), int() will round the value towards zero.
It is important to note that if you pass a string to the int() function, it will attempt to convert the string to an integer. If the string contains non-numeric characters, this will result in a ValueError. To avoid this, you can use the isnumeric() method to check if a string is numeric before attempting to convert it to an integer.
Implementing String Slicing for Truncation
You can also use string slicing to truncate a string or list to a specified length. This can be useful when you need to limit the amount of text displayed in a user interface or when working with large datasets. Here’s an example:
s = "Hello, world!"truncated = s[:5]print(truncated)
This code will output:
Hello
The syntax for string slicing is string[start:end], where start is the index of the first character you want to include and end is the index of the first character you want to exclude. In this example, we are slicing the string s to include the first five characters.
String slicing can also be used to extract specific portions of a string, such as the first word or the last name in a list of names. It is a powerful tool for working with text data in Python.
Truncating Floating-Point Numbers
Truncating floating-point numbers in Python can be a bit more complicated than truncating integers or strings. This is because floating-point numbers are inherently imprecise, and rounding errors can occur. However, there are several ways to truncate floating-point numbers in Python.
One way to truncate a floating-point number is to use the int() function. This function takes a floating-point number as an argument and returns the integer value of that number. However, this method simply drops the decimal portion of the number, rather than rounding it.
Another way to truncate a floating-point number is to use the math.trunc() function. This function takes a floating-point number as an argument and returns the integer value of the number, truncated towards zero. For example, math.trunc(3.2) would return 3, while math.trunc(-3.2) would return -3.
Truncating to a Specific Decimal Place
If you want to truncate a floating-point number to a specific decimal place, you can use the string formatting syntax. Here’s an example:
x = 3.14159
truncated = "%0.2f" % x
print(truncated)
This will output:
3.14
The string formatting syntax “%0.2f” tells Python to format the value as a float with two decimal places. This method simply drops any digits beyond the specified decimal place, rather than rounding the number.
If you want to round the number to a specific decimal place before truncating it, you can use the round() function before applying the string formatting syntax. For example:
x = 3.14159
rounded = round(x, 2)
truncated = "%0.2f" % rounded
print(truncated)
This will output:
3.14
Rounding Floating-Point Numbers
If you want to round a floating-point number to the nearest integer, you can use the round() function. Here’s an example:
x = 3.14159
rounded = round(x)
print(rounded)
This will output:
3
If you want to round a floating-point number to a specific decimal place, you can use the round() function with a second argument specifying the number of decimal places to round to. Here’s an example:
x = 3.14159
rounded = round(x, 2)
print(rounded)
This will output:
3.14
It’s important to note that rounding floating-point numbers can also introduce rounding errors, especially if you’re working with very large or very small numbers. In these cases, it’s often better to use other methods of truncation, such as math.trunc() or the string formatting syntax.
Truncating Lists and Arrays
Truncating lists and arrays is a common operation in Python, especially when dealing with large datasets. In this article, we will explore different methods to truncate lists and arrays in Python.
Truncating Lists with Slicing
Slicing is a powerful feature in Python that allows you to extract a portion of a list or array. You can use slicing to truncate a list to a specified length. Here’s an example:
myList = [1, 2, 3, 4, 5]
truncated = myList[:3]
print(truncated)
This will output:
[1, 2, 3]In the above example, we used slicing to extract the first three elements of the list myList. The colon (:) in the slicing notation indicates that we want to extract all the elements from the beginning of the list up to (but not including) the element at index 3.
You can also use slicing to truncate a list from the end. Here’s an example:
myList = [1, 2, 3, 4, 5]
truncated = myList[:-2]
print(truncated)
This will output:
[1, 2, 3]In the above example, we used slicing to extract all the elements from the beginning of the list up to (but not including) the second last element.
Truncating NumPy Arrays
If you are working with NumPy arrays, you can use the numpy.trunc() function to truncate values. Here’s an example:
import numpy as np
myArray = np.array([1.1, 2.2, 3.3, 4.4, 5.5])
truncated = np.trunc(myArray)
print(truncated)
This will output:
[1. 2. 3. 4. 5.]In the above example, we used the numpy.trunc() function to truncate all the decimal values in the array myArray to integers. The function returns an array of the same shape as the input array, with each value truncated to an integer.
You can also use slicing with NumPy arrays to truncate them. Here’s an example:
import numpy as np
myArray = np.array([1, 2, 3, 4, 5])
truncated = myArray[:3]
print(truncated)
This will output:
[1 2 3]In the above example, we used slicing to extract the first three elements of the array myArray.
Truncating lists and arrays is a simple and efficient way to reduce the size of your data and make it more manageable. Whether you’re working with lists or NumPy arrays, Python provides several ways to truncate them.
Conclusion
In this article, we’ve covered what truncation is, why it is useful, and several ways to truncate values in Python, including using the math.trunc() function, converting values to integers with the int() function, and implementing string slicing. We’ve also looked at how to truncate floating-point numbers and truncate lists and NumPy arrays. Armed with this knowledge, you can now truncate values in Python with ease.