Python is a high-level programming language that is commonly used in various industries such as web development, data science, and artificial intelligence. Understanding programming concepts in Python is essential for any developer looking to create efficient, robust code. In this article, we will explore the basics of the if and or operators in Python and how they can be used to create decision-making programs.
The Basics of if and or in Python
Before we dive into how to use if and or in Python, it’s important to understand what these operators are and how they work.
What are if and or?
The if operator is used in programming to execute a specific block of code when a certain condition is met. The condition is evaluated as either true or false, and if it’s true, the block of code is executed. On the other hand, the or operator is used to combine multiple conditions together. If any of the conditions are true, the entire expression will evaluate to true.
How if and or Work in Python
Certainly! In Python, the if and or statements are powerful tools for controlling the flow of your program and making decisions based on certain conditions. Let’s take a closer look at how they work.
if statement:
- The if statement allows you to execute a block of code only if a certain condition is met. Here’s the basic syntax:
if condition:
# code to execute if condition is True
The condition can be any expression that evaluates to either True or False. If the condition is True, the indented block of code following the if statement will be executed. If the condition is False, the block of code will be skipped.
You can also include an else clause to specify a block of code to execute when the condition is False.
Here’s an example:
x = 10 if x > 5: print("x is greater than 5") else: print("x is less than or equal to 5")
In this example, since the condition x > 5 is True, the code inside the first block will be executed, and it will print “x is greater than 5”.
or operator:
- The or operator allows you to combine multiple conditions and check if at least one of them is True. Here’s an example:
x = 10 if x < 5 or x > 15: print("x is either less than 5 or greater than 15") else: print("x is between 5 and 15 (inclusive)")
In this case, the condition x < 5 or x > 15 evaluates to False because neither of the conditions is True. Therefore, the code inside the else block will be executed, and it will print “x is between 5 and 15 (inclusive)”.
You can also use the or operator in more complex conditions. Here’s an example:
x = 10 if (x > 5 and x < 15) or x == 20: print("x is between 5 and 15 (exclusive), or it is equal to 20") else: print("x is not in the specified range")
In this case, the condition (x > 5 and x < 15) or x == 20 evaluates to True because the first part (x > 5 and x < 15) is True. Therefore, the code inside the first block will be executed, and it will print “x is between 5 and 15 (exclusive), or it is equal to 20”.
You can combine multiple if statements and or operators to create more complex decision-making logic in your Python programs.
The Importance of Indentation
Python relies heavily on indentation to define code blocks. Therefore, it’s important to ensure that your code is properly indented, or else it may not work as intended. The standard indentation level in Python is four spaces.
Now, let’s take a closer look at the if operator. In Python, the if statement can be followed by an optional else statement. The else statement is executed if the condition in the if statement is false. Additionally, Python also has an elif statement, which is short for “else if”. The elif statement allows you to check multiple conditions in a single if statement. If the first condition is false, the elif statement will be evaluated, and so on.
Let’s say you’re writing a program that checks whether a number is positive, negative, or zero. You could use an if statement with elif and else statements to accomplish this:
num = float(input(“Enter a number: “))if num > 0: print(“Positive number”)elif num == 0: print(“Zero”)else: print(“Negative number”)
When you run this program and enter a number, it will check whether the number is greater than zero, equal to zero, or less than zero, and print the appropriate message.
Now, let’s take a look at the or operator. The or operator can be used to check multiple conditions at once. For example, let’s say you want to check whether a person is eligible to vote in the United States. In order to be eligible to vote, a person must be a U.S. citizen and at least 18 years old. You could use the or operator to check both of these conditions:
age = int(input(“Enter your age: “))citizen = input(“Are you a U.S. citizen? (y/n) “)if age >= 18 and (citizen == “y” or citizen == “Y”): print(“You are eligible to vote.”)else: print(“You are not eligible to vote.”)
When you run this program, it will ask for your age and whether you are a U.S. citizen. If you are at least 18 years old and a U.S. citizen, it will print “You are eligible to vote.” Otherwise, it will print “You are not eligible to vote.”
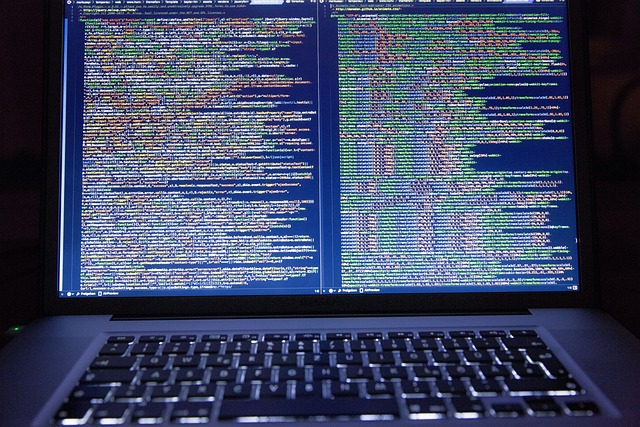
Conclusion
In conclusion, the if and or operators are powerful tools in Python programming. By using these operators, you can create complex programs that can make decisions based on multiple conditions. It’s important to remember to properly indent your code and use the correct syntax when using these operators. With a little practice, you’ll be able to use if and or like a pro in no time!
Using if Statements in Python
Now that we understand the basics of the if operator, let’s explore how to use it in Python.
Simple if Statements
The simplest form of an if statement is shown below:
if condition: # code block
The if statement is followed by a condition, which is evaluated as either true or false. If the condition is true, the code block that follows the if statement will be executed. Note that the code block is indented four spaces from the if statement.
if-else Statements
Sometimes you may want to execute one block of code if a condition is true and another block of code if the condition is false. This can be achieved with an if-else statement:
if condition: # code block for true conditionelse: # code block for false condition
If the condition is true, the code block following the if statement will be executed. If the condition is false, the code block following the else statement will be executed.
if-elif-else Statements
Sometimes you may have multiple conditions that you want to evaluate. These conditions can be evaluated with an if-elif-else statement:
if condition1: # code block for condition1 being trueelif condition2: # code block for condition2 being trueelse: # code block for both conditions being false
The elif statement is short for “else if.” If condition1 is true, Python will execute the code block following the first if statement. If condition1 is false and condition2 is true, Python will execute the code block following the elif statement. If both condition1 and condition2 are false, Python will execute the code block following the else statement.
Nested if Statements
You may also have situations where you need to evaluate multiple conditions within a code block. This can be achieved by nested if statements:
if condition1: if condition2: # code block for both conditions being true else: # code block for condition1 being true and condition2 being falseelse: #code block for condition1 being false
If condition1 is true, Python will evaluate condition2. If condition2 is true, Python will execute the code block following the second if statement. If condition2 is false, Python will execute the code block following the else statement of the first if statement. If condition1 is false, Python will execute the code block following the else statement of the first if statement.
Understanding the or Operator
The or operator is a logical operator that combines two or more conditions and evaluates to True if at least one of the conditions is True. It follows the following rules:
If the first condition is True, the entire expression is considered True, and the subsequent conditions are not evaluated. This is known as short-circuit evaluation.
If the first condition is False, the or operator evaluates the second condition, and so on. It stops evaluating as soon as it encounters a True condition. If none of the conditions evaluate to True, the expression as a whole evaluates to False.
Here’s an example to illustrate these rules:
x = 5
y = 10
if x > 10 or y < 15:
print("At least one condition is True.")
else:
print("No conditions are True.")
In this example, the first condition x > 10 is False, but the second condition y < 15 is True. Since at least one condition is True, the code inside the if block will be executed, and it will print “At least one condition is True.”
It’s important to note that the or operator returns the value of the first True condition it encounters, or the value of the last False condition if none of the conditions are True.
For example:
x = 10
y = 20
result = x < 5 or y > 15
print(result) # Output: True
In this example, the condition x < 5 is False, but the condition y > 15 is True. Since the or operator encounters a True condition, it returns True as the final result.
You can also use parentheses to group conditions and control the order of evaluation. For example:
x = 5
y = 10
z = 15
if (x < y) or (y < z and x > z):
print("At least one condition is True.")
else:
print("No conditions are True.")
In this case, the first condition (x < y) is True, so the entire expression is considered True, and the code inside the if block will be executed.
Understanding how the or operator works is essential for creating complex conditional logic in your Python programs. It allows you to test multiple conditions and execute code based on the evaluation of those conditions.
Combining Conditions with or
The or operator can be used to combine multiple conditions together:
if condition1 or condition2 or condition3: # code block for any of the conditions being true
In this example, the code block will be executed if any of the three conditions are true.
Short-circuit Evaluation with or
The or operator can be used to implement short-circuit evaluation, which can improve code efficiency. Short-circuit evaluation means that if the left-hand side of the expression is true, the right-hand side of the expression will not be evaluated. For example:
x = 0if x == 0 or 1/x == 1: # code block
In this example, Python will not evaluate 1/x == 1 because x == 0 is true. If x were any other value except 0, the expression 1/x == 1 would have to be evaluated.
Practical Examples of if and or in Python
Now that we understand how to use if and or in Python, let’s explore some practical examples of how they can be used in real-world programs.
Filtering Data Based on Multiple Conditions
Suppose you have a list of integers and you want to filter out any values less than 5 or greater than 10:
data = [2, 5, 8, 11]filtered_data = []for value in data: if value < 5 or value > 10: continue filtered_data.append(value)print(filtered_data) # [5, 8]
In this example, the if statement is used to filter out any values less than 5 or greater than 10. The continue statement is used to skip over any values that do not meet the condition. The resulting list contains only the values that meet the condition.
Creating a Simple Decision-Making Program
Suppose you want to create a program that will prompt the user for their age and tell them if they are old enough to vote:
age = int(input("Enter your age: "))if age >= 18: print("You are old enough to vote!")else: print("You are not old enough to vote.")
In this example, the input function is used to prompt the user for their age. The if statement is used to determine if the user is old enough to vote. If the user is 18 or older, the program will display a message indicating that they are old enough to vote. If the user is younger than 18, the program will display a message indicating that they are not old enough to vote.
Validating User Input with if and or
Suppose you want to create a program that will prompt the user for a positive integer:
while True: value = int(input("Enter a positive integer: ")) if value > 0: break print("Invalid input. Please enter a positive integer.")print("You entered", value)
In this example, a while loop is used to repeatedly prompt the user for input until they enter a positive integer. The if statement is used to validate the user’s input. If the user enters a positive integer, the break statement is used to exit the loop. If the user enters a non-positive integer, the program will display a message indicating that the input is invalid and prompt the user for input again.
In conclusion, the if and or operators are powerful tools in Python that allow you to control the flow of your program and make decisions based on conditions.
Here are the key points to remember:
- The if statement is used to execute a block of code only if a specific condition is True;
- You can use the else clause with the if statement to specify a block of code to execute when the condition is False;
- The or operator allows you to combine multiple conditions and evaluates to True if at least one of the conditions is True;
- Short-circuit evaluation occurs when the or operator stops evaluating conditions as soon as it encounters a True condition;
- The or operator returns the value of the first True condition encountered, or the value of the last False condition if none of the conditions are True;
- Parentheses can be used to group conditions and control the order of evaluation.
By mastering the usage of the if and or operators, you can create more dynamic and flexible programs. These operators are essential building blocks for implementing decision-making logic and filtering data based on complex conditions. Keep in mind the proper indentation and logical combination of these operators to create clear and efficient code.
Conclusion
If and or operators are fundamental concepts in Python programming. They can be used to create decision-making programs and filter data based on complex conditions. Understanding how to use these operators can help you create efficient and robust code. Remember to properly indent your code and combine the if and or operators effectively to create effective programs.