In programming, making decisions based on certain conditions is an essential aspect of the process. Python, being a popular language for data analytics and web development, offers various conditional statements, including the ‘if equals’ statement. In this article, we will explore the basics of the Python ‘if equals’ statement, its syntax, comparison operators used for comparisons, and some common use cases of the statement. Additionally, we will discuss how to combine ‘if equals’ with other operators and best practices for using it.
The Basics of Python ‘if equals’ Statement
The ‘if equals’ statement in Python is a conditional statement that tests the equality of two variables. A boolean true or false value is returned based on whether these variables are equal or not. The statement compares the variables on the left and right side of the ‘==’ operator.
When using the ‘if equals’ statement, it is important to note that the two variables being compared must be of the same data type. For example, comparing a string to an integer will always return false, even if the values are the same.
Syntax of the ‘if equals’ Statement
Generally speaking, the syntax for an ‘if equals’ statement in Python is:
if x == y: # the code block to execute goes here if the condition is TRUEelse: # the code block to execute goes here if the condition is FALSE
The code block after the colon is only executed if the condition is true. If the condition is false, it is skipped, and the next block of code is executed. It is also possible to chain multiple ‘if equals’ statements together using the ‘elif’ keyword. This allows for more complex conditional logic in your code.
Comparison Operators in Python
Python has several comparison operators, including:
- ‘==’ for equality comparison;
- ‘!=’ for non-equality comparison;
- ‘<‘ and ‘>’ for less than and greater than comparison, respectively;
- ‘<=’ and ‘>=’ for less than or equal to and greater than or equal to comparison, respectively.
These comparison operators can be used in conjunction with the ‘if equals’ statement to create more complex conditional logic in your code. For example, you could use the greater than operator to check if a variable is greater than a certain value, and then use the ‘if equals’ statement to check if it is also equal to another value.
Common Use Cases for ‘if equals’
The ‘if equals’ statement can be used in various scenarios, including:
- Validating user input: When taking input from a user, it is important to validate that the input is of the correct data type and within a certain range. The ‘if equals’ statement can be used to check if the input matches the expected value;
- Checking whether an element exists in a list: When working with lists, it is often necessary to check whether a certain element exists in the list. The ‘if equals’ statement can be used to compare the value of each element in the list to the desired value;
- Comparing the values of two variables: When working with multiple variables in your code, it may be necessary to compare the values of two variables. The ‘if equals’ statement can be used to check if the values are equal.
Overall, the ‘if equals’ statement is a powerful tool in Python that allows for complex conditional logic in your code.
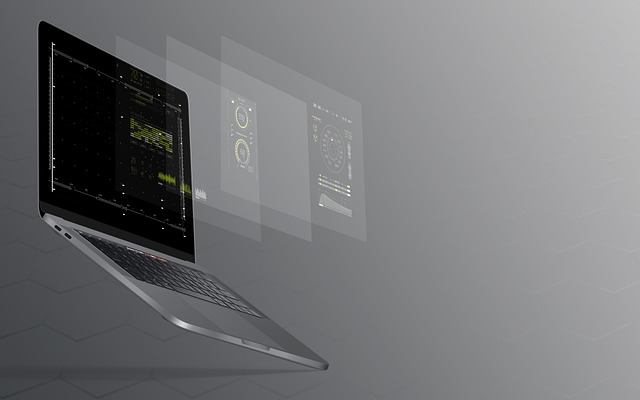
Conditional Statements in Python
Conditional Statements are a fundamental aspect of programming languages, particularly for languages like Python and Java. They allow programmers to create logic in their code that can make decisions based on certain conditions. In Python, there are three primary conditional statements: ‘if’, ‘elif’, and ‘else’.
The ‘if’ Statement
The ‘if’ statement is the basic conditional statement in Python. Its syntax is straightforward and easy to understand. The ‘if’ statement is used to check whether the condition provided is true or false. If the condition is true, then the code block inside the ‘if’ statement will be executed. If the condition is false, the code block inside the ‘if’ statement will be skipped.
For example, suppose we want to check whether a number is greater than 5 or not. We can use the ‘if’ statement to do this:
num = 7if num > 5: print("The number is greater than 5")
In this example, the condition ‘num > 5’ is true, so the code block inside the ‘if’ statement will be executed, and the output will be ‘The number is greater than 5’.
The ‘elif’ Statement
The ‘elif’ statement is used to check several conditions if the first condition is not met. It stands for “else if”. The ‘elif’ statement is useful when we want to check multiple conditions and execute different code blocks based on those conditions.
For example, suppose we want to check whether a number is negative, positive, or zero. We can use the ‘elif’ statement to do this:
num = 0if num > 0: print("The number is positive")elif num < 0: print("The number is negative")else: print("The number is zero")
In this example, if the number is greater than 0, the code block inside the first ‘if’ statement will be executed, and the output will be ‘The number is positive’. If the number is less than 0, the code block inside the ‘elif’ statement will be executed, and the output will be ‘The number is negative’. If the number is 0, the code block inside the ‘else’ statement will be executed, and the output will be ‘The number is zero’.
The ‘else’ Statement
The ‘else’ statement only executes when all preceding conditions are false. Its syntax is straightforward and easy to understand. The ‘else’ statement is used to provide a default code block to execute when none of the preceding conditions are true.
For example, suppose we want to check whether a number is even or odd. We can use the ‘if’, ‘elif’, and ‘else’ statements to do this:
num = 4if num % 2 == 0: print("The number is even")else: print("The number is odd")
In this example, if the number is divisible by 2, the code block inside the ‘if’ statement will be executed, and the output will be ‘The number is even’. If the number is not divisible by 2, the code block inside the ‘else’ statement will be executed, and the output will be ‘The number is odd’.
Combining ‘if equals’ with Other Operators
The ‘if equals’ statement is a powerful tool in programming, but it becomes even more useful when combined with other operators to create more complex conditions.
Let’s take a closer look at how the ‘and’ and ‘or’ operators can be used in conjunction with ‘if equals’.
Using ‘and’ and ‘or’ Operators
The ‘and’ operator is used to check if both conditions are true, while the ‘or’ operator is used to check if either condition is true. This can be incredibly useful in situations where you need to check multiple conditions before executing a block of code.
For example:
if x == y and z == 5: # the code block to execute goes here if both conditions are TRUEelif x == y or z == 5: # the code block to execute goes here if one of the conditions are TRUE
In the above code, if both x and y are equal AND z is equal to 5, then the code block will be executed. If only one of the conditions is true, then the second code block will be executed.
Nesting ‘if equals’ Statements
The ‘if equals’ statement can also be nested within another ‘if equals’ statement to create even more complex expressions. The inner statement is evaluated first before the outer, and all conditions must be true for the code block to execute.
For example:
if x == y: if z == 5: # the code block to execute goes here if both conditions are TRUE
In the above code, if x is equal to y, then the inner ‘if equals’ statement will be evaluated. If z is also equal to 5, then the code block will be executed. If either condition is false, then the code block will not be executed.
By using ‘if equals’ statements in combination with other operators, you can create incredibly powerful expressions that allow you to control the flow of your code in a precise and efficient manner.
Python ‘if equals’ Statement Best Practices
The ‘if equals’ statement is a fundamental tool in Python that enables developers to make decisions based on certain conditions. It evaluates a condition and executes a block of code if the condition is true. In this article, we will explore the best practices for using the ‘if equals’ statement in Python.
Proper Indentation
Proper indentation is critical in Python because it determines which code blocks are executed based on the condition. Therefore, it is essential to ensure accurate tabs and spaces as required by the Python syntax. Improper indentation can lead to syntax errors and unexpected program behavior.
For example, consider the following code:
if x == 5:print("x is equal to 5")
The code above will result in a syntax error because the print statement is not indented correctly. To fix the error, we need to indent the print statement:
if x == 5: print("x is equal to 5")
Keeping Code Readable
Cluttered code can be challenging to read, especially when there are numerous if statements. To increase readability, use proper spacing, and avoid unnecessary nested conditions.
For example, consider the following code:
if x == 5 and y == 10 and z == 15: print("x is 5, y is 10, and z is 15")
The code above is difficult to read and understand. We can improve its readability by breaking it down into multiple lines:
if x == 5 and y == 10 and z == 15: print("x is 5, y is 10, and z is 15")
Avoiding Unnecessary Complexity
Try to keep the code simple and clear to avoid unnecessary complexity that contributes to debugging hassles. Use meaningful variable names, comments, and descriptive code blocks to make it easy to understand the code’s functionality.
For example, consider the following code:
if len(my_list) > 0: print("The list is not empty")else: print("The list is empty")
We can simplify the code above by using the Boolean value of the list:
if my_list: print("The list is not empty")else: print("The list is empty")
By simplifying the code, we make it easier to understand and maintain.
In summary, the ‘if equals’ statement is a powerful tool in Python that enables developers to make decisions based on certain conditions. By following these best practices, you can write efficient and readable code that is easy to maintain and debug.