Working with strings is a fundamental aspect of programming. In C#, strings are a sequence of characters, usually used to store text. However, they can present some challenges when it comes to removing spaces. If you’re struggling with whitespace in your strings, this guide will help you understand the different types of spaces and the various methods for removing them from a C#.
Understanding Strings in C#
Before we dive into removing spaces, let’s review some basics about strings in C#. In C#, strings are represented by the keyword. They are immutable, meaning once created, they cannot be modified. Instead, any operation on a returns a new as a result. This feature is important to keep in mind when working with strings in C#.
What is a String?
Simply put, is a sequence of characters. These can include letters, numbers, special characters. Spaces are common in text, but may be undesired in certain circumstances, such as when searching for a specific phrase.
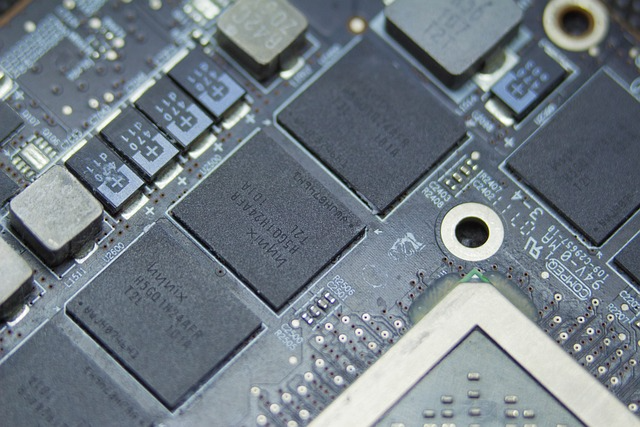
Different Types of Spaces in Strings
When it comes to working with strings, one of the common tasks is to manipulate within them. Before we can dive into methods for removing spaces, it’s important to understand the different types of spaces that can occur in strings.
The most common type of space in text is the regular space. These spaces, also called “soft spaces,” are used to separate words or characters within a sentence. In C#, regular spaces are represented by the character.
Another type of space is the non-breaking space. These spaces are similar to regular spaces, but they are designed to prevent line breaks or word wrapping. In C#, non-breaking spaces are represented by the &;nbsp; HTML entity. Non-breaking spaces are often used in web development to ensure that certain elements, such as buttons or links, remain on the same line.
The third type of space is the tab space. These spaces are used to horizontally indent text. In C#, tab spaces are represented by the \t character. Tab spaces are often used to align columns of text, such as in a table or code block.
It’s important to keep in mind the different types of spaces when working with strings, as they can affect the appearance and functionality of your text. Understanding how to manipulate these spaces can help you format your text in a way that is visually appealing and easy to read.
Methods to Remove Spaces from a String
Spaces in a string can be a nuisance when trying to manipulate or analyze the data. Fortunately, there are several methods available in C# to remove spaces from a string. In this article, we will explore some of the most commonly used methods for removing spaces from a string.
Using the Replace Method
The simplest method for removing spaces from a string in C# is to use the Replace method. This method searches for the specified string value and replaces it with the specified replacement string value.
For instance, if you want to remove all spaces in a string, you can use the following code snippet:
string str = "This is a string with spaces.";string newStr = str.Replace(" ", "");Console.WriteLine(newStr);
The output of this code will be: Thisisastringwithspaces.
This code replaces all regular spaces with an empty string, effectively removing them from the original string.
Using the Trim Method
The Trim method removes all leading and trailing whitespace characters from a string. This method is particularly useful for removing any unwanted white spaces from the beginning or end of a string.
Here’s an example:
string str = " This is a string with spaces. ";string newStr = str.Trim();Console.WriteLine(newStr);
The output of this code will be: This is a string with spaces.
The Trim method removed the leading and trailing spaces in the original string and returned a new string with the content we desired.
Using Regular Expressions
Regular expressions are a powerful tool for string manipulation. They allow you to define patterns that match specific sets of characters. In the context of removing spaces from a string, regular expressions can be used to match all whitespace characters and remove them.
Here’s an example:
string str = "This is a string with spaces.";string newStr = Regex.Replace(str, @"\s+", "");Console.WriteLine(newStr);
The output of this code will be: Thisisastringwithspaces.
This code uses the Regex.Replace method to match any instance of one or more whitespace characters in the string and replaces them with an empty string.
Using StringBuilder and Loops
The StringBuilder class is a mutable string class that can be used to build and manipulate strings. It provides methods for inserting, appending, and removing characters from the string. In the context of removing spaces from a string, the StringBuilder class can be used in combination with loops to iteratively remove spaces.
Here’s an example:
string str = "This is a string with spaces.";StringBuilder newStrBuilder = new StringBuilder(str.Length);foreach (char c in str){ if (!Char.IsWhiteSpace(c)) { newStrBuilder.Append(c); }}string newStr = newStrBuilder.ToString();Console.WriteLine(newStr);
The output of this code will be: Thisisastringwithspaces.
This code uses a foreach loop to iterate through each character in the original string. It checks whether the character is whitespace and, if it is not, appends it to a new StringBuilder instance. Finally, the resulting string is returned using the ToString method of the StringBuilder instance.
Overall, these methods provide efficient and effective ways to remove spaces from a string in C#. Depending on your specific needs, one method may be more suitable than the others. By understanding the different methods available, you can choose the best approach for your particular situation.
Removing Spaces at Specific Positions
When working with strings, it is common to encounter situations where you need to remove spaces from specific positions within a string. Fortunately, there are several methods available in C# to accomplish this task.
Removing Leading Spaces
Leading spaces are spaces that occur at the beginning of a string. These spaces can be removed using the TrimStart method. This method removes any whitespace characters from the beginning of a string.
Here is an example:
string str = " This is a string with leading spaces.";string newStr = str.TrimStart();Console.WriteLine(newStr);
The output of this code will be: This is a string with leading spaces.
As you can see, the TrimStart method removed the leading spaces from the original string.
Removing Trailing Spaces
Trailing spaces are spaces that occur at the end of a string. These spaces can be removed using the TrimEnd method. This method removes any whitespace characters from the end of a string.
Here is an example:
string str = "This is a string with trailing spaces. ";string newStr = str.TrimEnd();Console.WriteLine(newStr);
The output of this code will be: This is a string with trailing spaces.
As you can see, the TrimEnd method removed the trailing spaces from the original string.
Removing Spaces Between Words
Sometimes, you may need to remove spaces between words in a string, while leaving any leading or trailing spaces intact. One way to accomplish this is to use a regular expression pattern that matches spaces occurring between two word characters.
Here is an example:
string str = "This is a string with spaces between words.";string newStr = Regex.Replace(str, @"\b\s+\b", " ");Console.WriteLine(newStr);
The output of this code will be: This is a string with spaces between words.
In this example, the regular expression pattern \b\s+\b matches any instance of one or more whitespace characters between two word boundaries (represented by the \b metacharacter).
Overall, these methods provide a flexible and powerful way to remove spaces from specific positions within a string.
Conclusion
Removing spaces from a string in C# can be accomplished using various built-in methods, regular expressions, and loops. Different methods may be more appropriate depending on the specific use case and the types of spaces that need to be removed. Hopefully, this guide has provided you with a better understanding of how to manipulate strings in C# and the available options for removing spaces.