In today’s fast-paced digital world, building efficient and high-performance web servers is crucial for delivering seamless user experiences. One language that has gained significant popularity for server-side development is Go, also known as Golang. With its simple syntax, powerful standard library, and inherent concurrency support, Go provides a solid foundation for creating robust and performant web servers.
In this article, we will dive into the world of Go’s HTTP server capabilities, exploring routes, Go HTTP router, and demonstrating how to write efficient server code. Whether you’re a seasoned Go developer or new to the language, this article will equip you with the knowledge and tools to build scalable and high-performance web servers.
Understanding Routes in Go
Routes are essential components of any web server as they define the endpoints and actions associated with specific URLs. In Go, routes are managed using the “net/http” package. By leveraging this package, you can define multiple routes and map them to appropriate handler functions. These functions handle incoming HTTP requests, process them, and generate corresponding responses.
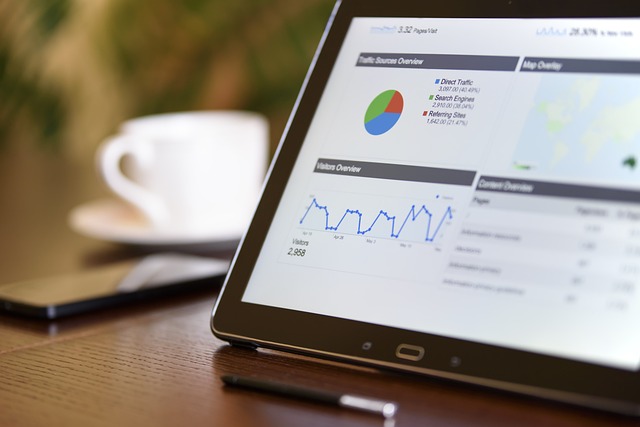
Exploring Go HTTP Router
Go offers several third-party HTTP routers that enhance the routing capabilities of the standard library. One popular router is the “gorilla/mux” package, known for its flexibility and simplicity. It allows you to define complex routes, handle route parameters, and even integrate middleware for advanced functionality such as authentication and request logging.
To demonstrate the usage of the Go HTTP router, consider the following example code:
```go
package main
import (
"fmt"
"log"
"net/http"
"github.com/gorilla/mux"
)
func main() {
router := mux.NewRouter()
// Define routes
router.HandleFunc("/", homeHandler)
router.HandleFunc("/users/{id}", userHandler)
// Start the server
fmt.Println("Server started on port 8080")
log.Fatal(http.ListenAndServe(":8080", router))
}
func homeHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Welcome to the homepage!")
}
func userHandler(w http.ResponseWriter, r *http.Request) {
vars := mux.Vars(r)
userID := vars["id"]
fmt.Fprintf(w, "User ID: %s", userID)
}
```
In the above code snippet, we import the “gorilla/mux” package and create a new router. We define two routes: one for the homepage (“/”) and another for retrieving user information (“/users/{id}”). The handler functions, `homeHandler` and `userHandler`, are responsible for generating the appropriate responses for each route.
Creating an Example Table
To provide a comprehensive overview of Go’s HTTP server capabilities, let’s create a table outlining various HTTP methods, their corresponding routes, and the associated handler functions. This table will serve as a handy reference guide when building your own web servers:
Method | Route | Handler Function |
---|---|---|
GET | /users | getUsersHandler |
POST | /users | createUserHandler |
GET | /users/{id} | getUserHandler |
PUT | /users/{id} | updateUserHandler |
DELETE | /users/{id} | deleteUserHandler |
This table showcases common HTTP methods such as GET, POST, PUT, and DELETE, along with their respective routes and handler functions. You can extend this table based on your specific server requirements.
Conclusion:
In this article, we delved into the world of Go’s HTTP server capabilities, exploring routes, the Go HTTP router, and demonstrating how to write efficient server code. By leveraging Go’s simplicity and powerful libraries like “gorilla/mux,” you can build scalable and high-performance web servers.
Remember to optimize your server code by implementing best practices, such as minimizing allocations, utilizing connection pooling, and applying proper error handling. With Go’s concurrency support, you can take full advantage of its inherent parallelism to handle multiple requests concurrently.
Armed with the knowledge gained from this article, you are now well-equipped to embark on your journey of building blazing-fast and reliable web servers using Go. Happy coding!
Add comment