While loops are essential constructs in any programming language as they allow us to execute a block of code repeatedly until a specific condition is met. In Go, the while is implemented using the `for` keyword, which might seem a bit confusing for newcomers. However, once you grasp the concept and understand the syntax, while in Go become powerful tools for solving various programming challenges.
This article aims to provide a comprehensive guide on how to program while in Go effectively. We will explore different examples, demonstrate idiomatic techniques, and share best practices to help you optimize your code and enhance your programming skills. Whether you’re a beginner or an experienced developer, this guide will equip you with the knowledge needed to master.
How to Program a While Loop in Go?
To begin our journey into mastering, let’s first understand how to program them. In Go, we use the `for` statement to create while loops. The basic syntax looks like this:
```go
for condition {
// Code to be executed
}
```
Here, `condition` represents a boolean expression that determines whether the should continue executing or not. As long as the condition evaluates to true, the will keep iterating. Once the condition becomes false, the will exit, and the program will continue executing the code following the loop.
The condition can be any expression that evaluates to a boolean value, such as a comparison (`<`, `>`, `<=`, `>=`, `==`, `!=`), a logical operation (`&&`, `||`), or a function call that returns a boolean value.
It’s important to note that unlike some other programming languages, Go does not have a specific `while` keyword. Instead, the `for` keyword is used in a flexible manner to create. This design choice allows for consistency and simplicity in Go’s syntax.
Let’s explore some variations and common patterns for using while loops in Go.
Basic While Loop
The basic structure of a while is straightforward. Let’s take a look at a simple example:
```go
package main
import "fmt"
func main() {
i := 0
for i < 5 {
fmt.Println(i)
i++
}
}
```
In this example, we initialize a variable `i` to 0. The continues executing as long as the condition `i < 5` is true. We print the current value of `i` and then increment it using the `i++` statement. This will output the numbers 0 to 4.
Infinite Loop
In some cases, you might want to create an infinite where the condition is always true. This is useful when you want a certain block of code to repeat indefinitely until a specific break condition is met. Here’s an example:
```go
package main
import "fmt"
func main() {
for {
fmt.Println("This is an infinite loop!")
}
}
```
In this example, we omit the condition part of the `for` statement, creating. They will keep printing the specified message indefinitely. Be cautious when using ensure you have a proper exit condition to prevent your program from running indefinitely.
Condition with Break
While can also incorporate a `break` statement to exit the prematurely. This is useful when you want to stop the execution based on a specific condition within the body. Consider the following example:
```go
package main
import "fmt"
func main() {
i := 0
for {
if i == 5 {
break
}
fmt.Println(i)
i++
}
fmt.Println("Loop exited!")
}
```
In this example, we create. However, we include an `if` statement inside the body that checks if `i` is equal to 5. If the condition is true, the `break` statement is executed, and exited. The program then continues to execute the code following.
This pattern allows you to control the loop’s behavior and exit it based on certain conditions.
Now that we have a solid understanding of how to program while loops in Go, let’s explore various examples and scenarios where while loops can be utilized effectively.
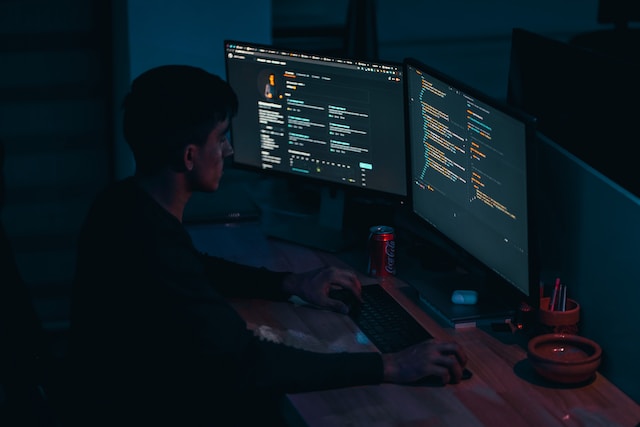
Code a Go While Loop with For
In Go, the `for` statement is versatile and can be used to create while loops. We can omit the initialization and post-loop statements, effectively creating a while loop. Let’s see an example:
```go
package main
import "fmt"
func main() {
i := 0
for i < 5 {
fmt.Println(i)
i++
}
}
```
In this example, we initialize a variable `i` to 0 before the loop. The condition `i < 5` determines whether the loop should continue executing. Inside the loop, we print the current value of `i` and then increment it using the `i++` statement.
The output of this program will be:
```
0
1
2
3
4
```
Let’s break down the steps of this while loop:
- We initialize `i` to 0 before the loop;
- The loop checks if `i` is less than 5. Since the initial value of `i` is 0, the condition is true, and the loop is entered;
- Inside the loop, we print the value of `i`, which is 0, and then increment `i` by 1 using the `i++` statement;
- The loop condition is checked again. Now `i` is 1, which is still less than 5, so the loop continues to execute;
- This process repeats until `i` becomes 5. At that point, the condition `i < 5` evaluates to false, and the loop is exited;
- The program continues to execute the code following the loop, if any.
Using the `for` statement to create while loops in Go provides flexibility and clarity. By omitting the initialization and post-loop statements, we can focus solely on the loop condition, making the code concise and easy to understand.
Now that we’ve seen how to code a while loop in Go, let’s dive into more examples and explore different scenarios where while loops can be applied effectively.
Examples of While Loops in Go
Now that we understand the basic syntax and structure of while loops in Go, let’s explore some practical examples to gain a deeper understanding of their usage.
Example: Count with a Basic While Loop
Suppose we want to count from 1 to 10 using a while loop in Go. We can achieve this by modifying the previous example as follows:
```go
package main
import "fmt"
func main() {
count := 1
for count <= 10 {
fmt.Println(count)
count++
}
}
```
In this example, we initialize a variable `count` to 1 before the loop. The loop continues executing as long as the condition `count <= 10` is true. Inside the loop, we print the current value of `count` and then increment it using the `count++` statement.
The output of this program will be:
```
1
2
3
4
5
6
7
8
9
10
```
By modifying the initialization and condition of the while loop, we can achieve different counting patterns or iterate over a specific range of values.
Example: Scan for User Input with a While Loop
Another common scenario is reading user input until a specific condition is met. Let’s consider an example where we ask the user to enter a positive number, and we keep asking until a valid input is provided:
```go
package main
import (
"fmt"
"strconv"
)
func main() {
var input string
validInput := false
for !validInput {
fmt.Print("Enter a positive number: ")
fmt.Scanln(&input)
if num, err := strconv.Atoi(input); err == nil && num > 0 {
validInput = true
}
}
fmt.Println("Valid input received:", input)
}
```
In this example, we declare a variable `input` to store the user’s input as a string. We also introduce a boolean variable `validInput` to track if the input is valid or not. The while loop continues executing as long as the condition `!validInput` (not validInput) is true.
Inside the loop, we prompt the user to enter a positive number and scan the input using `fmt.Scanln`. We then use `strconv.Atoi` to convert the input from a string to an integer (`num`). If the conversion is successful and `num` is greater than 0, we set `validInput` to true, indicating that a valid input has been received, and the loop will exit.
This example demonstrates how a while loop can be used to repeatedly ask for input until a valid condition is met, ensuring the user provides the expected type of input.
Example: Empty a Collection with the While Loop
While loops can also be useful for emptying a collection, such as a slice, by removing elements until the collection becomes empty. Consider the following example:
```go
package main
import "fmt"
func main() {
numbers := []int{1, 2, 3, 4, 5}
for len(numbers) > 0 {
fmt.Println("Removing element:", numbers[0])
numbers = numbers[1:]
}
fmt.Println("Collection is now empty")
}
```
In this example, we initialize a slice called `numbers` with some values. The while loop continues executing as long as the condition `len(numbers) > 0` is true. Inside the loop, we print and remove the first element of the slice using slicing (`numbers = numbers[1:]`). This effectively reduces the length of the slice by one in each iteration.
The output of this program
will be:
```
Removing element: 1
Removing element: 2
Removing element: 3
Removing element: 4
Removing element: 5
Collection is now empty
```
By utilizing the while loop, we can iterate over the collection and remove elements until it becomes empty, achieving the desired result.
These examples demonstrate the versatility of while loops in Go and their applicability in various scenarios. Whether it’s counting, validating user input, or manipulating collections, while loops provide a powerful mechanism for repetitive execution and flow control.
Now that we’ve explored practical examples of while loops, let’s move on to the next section where we’ll delve into idiomatic approaches to using while loops in Go.
Idiomatic Go: Using the While Loop without Semicolons
While Go allows the use of semicolons to omit loop components, it is considered more idiomatic to omit the semicolons altogether. This approach aligns with Go’s philosophy of simplicity and readability. By omitting the semicolons, the code becomes cleaner and easier to understand. Let’s rewrite the previous examples using the idiomatic approach.
Example: Count with a Basic While Loop (Idiomatic Go)
```go
package main
import "fmt"
func main() {
count := 1
for count <= 10 {
fmt.Println(count)
count++
}
}
```
In this idiomatic Go version, we remove the semicolons from the loop components. The loop initializes `count` to 1, checks the condition `count <= 10`, and increments `count` by 1 inside the loop body. This style adheres to Go’s preferred coding conventions and enhances code readability.
Example: Scan for User Input with a While Loop (Idiomatic Go)
```go
package main
import (
"fmt"
"strconv"
)
func main() {
var input string
validInput := false
for !validInput {
fmt.Print("Enter a positive number: ")
fmt.Scanln(&input)
if num, err := strconv.Atoi(input); err == nil && num > 0 {
validInput = true
}
}
fmt.Println("Valid input received:", input)
}
```
In this idiomatic Go version, we remove the semicolons from the loop components as well. The loop continues as long as the condition `!validInput` is true. Inside the loop, we prompt the user for input, scan it, and validate it. If the input is valid, we set `validInput` to true, and the loop exits. The use of while loop without semicolons aligns with Go’s recommended coding style.
By adhering to the idiomatic approach of omitting semicolons in the while loop, we make our Go code more readable, maintainable, and in line with the Go community’s coding standards.
Now that we’ve covered the idiomatic usage of while loops in Go, let’s move on to the conclusion where we summarize our learnings and wrap up the article.
Tables of While Loop Examples
To provide a comprehensive reference, let’s summarize the while loop examples we’ve discussed in a table format. This table will serve as a quick guide for understanding and implementing while loops in Go.
Example | Description |
---|---|
Count with a Basic While Loop | Increment a variable and print its value until a specific condition is met. |
Scan for User Input with a While Loop | Prompt the user for input until a valid condition is met. |
Empty a Collection with the While Loop | Remove elements from a collection until it becomes empty. |
Idiomatic Go: Using the While Loop | Code while loops in Go without semicolons, following Go’s coding convention. |
This table provides an overview of the examples covered, highlighting their purpose and usage. It serves as a handy reference when you need to implement while loops in different scenarios.
Feel free to refer to this table whenever you encounter a situation where while loops can be beneficial in your Go programming projects.
Remember that while loops offer flexibility and control over repetitive execution, and by understanding their implementation and best practices, you can utilize them effectively in your code.
By combining the knowledge gained from this article, the additional tips, and the table of examples, you have a solid foundation for mastering while loops in Go.
Keep exploring and experimenting with different applications of while loops to further enhance your programming skills and develop efficient, reliable Go programs.
Additional Tips and Best Practices
As you continue to work with while loops in Go, here are some additional tips and best practices to keep in mind:
- Keep the loop condition simple and readable: While loop conditions should be concise and easy to understand. Avoid complex expressions that may make the code confusing or error-prone;
- Ensure loop termination: Always ensure that your while loops have a clear termination condition. Without a proper termination condition, your loop may run indefinitely, causing your program to hang or crash;
- Use meaningful variable names: Choose meaningful names for your loop variables to enhance code readability. A well-named variable can provide insights into the purpose of the loop and improve overall code comprehension;
- Consider the scope of loop variables: Be mindful of the scope of variables used within the while loop. If you need to access loop variables outside of the loop, declare them before the loop;
- Break versus continue: Understand the difference between the `break` and `continue` statements. The `break` statement exits the loop entirely, while the `continue` statement skips the current iteration and proceeds to the next iteration of the loop;
- Use constants for loop conditions: When possible, consider using constants or predefined values for loop conditions. This practice enhances code maintainability and allows for easier modification of loop conditions in the future;
- Combine while loops with other control structures: While loops can be combined with other control structures like `if` statements and `switch` statements to create more complex and dynamic behavior in your programs. Explore different combinations to achieve the desired results;
- Test and debug your while loops: As with any code, it’s essential to test and debug your while loops thoroughly. Verify that the loop executes the expected number of times, handles edge cases correctly, and produces the desired output;
- Learn from existing code and examples: Studying and analyzing existing code and examples can provide valuable insights into different ways to utilize while loops effectively. Explore open-source projects or online resources to broaden your understanding of while loop usage in real-world scenarios;
- Refactor if necessary: If you find that a while loop becomes too complex or difficult to understand, consider refactoring your code. Breaking down the logic into smaller functions or using alternative control structures may improve code readability and maintainability.
By applying these tips and best practices, you can write while loops that are more robust, efficient, and easier to comprehend. Remember to experiment, practice, and seek feedback from peers to continuously improve your Go programming skills.
With dedication and practice, you’ll become proficient in leveraging while loops and other control structures to build elegant and functional applications in Go.
Conclusion
In this article, we have explored the concept of while loops in Go and learned how to program them using the `for` statement. While Go does not have a specific `while` keyword, the flexibility of the `for` statement allows us to create while loops effectively.
We started by understanding the basic syntax of a while loop in Go, which consists of a condition that determines the loop’s continuation. We discovered that the `for` statement can be used to create while loops by omitting the initialization and post-loop statements.
We then delved into practical examples to demonstrate the usage of while loops in Go. We saw how while loops can be used for counting, scanning user input, and emptying collections. Each example provided insights into different scenarios where while loops can be effectively utilized.
Additionally, we discussed the idiomatic approach to using while loops in Go, which involves omitting semicolons altogether. This style aligns with Go’s simplicity and readability principles, making the code cleaner and easier to understand.
By mastering while loops in Go, you have gained a valuable tool for controlling flow and repetition in your programs. While loops provide a flexible and powerful mechanism to iterate and handle various scenarios, from simple counting to complex input validation.
As you continue your Go programming journey, remember to leverage while loops appropriately, adhering to the idiomatic Go style to ensure your code remains clear and maintainable.
Keep practicing and exploring the vast capabilities of Go, and soon you’ll become proficient in utilizing while loops and other programming constructs to build efficient and robust applications.
Add comment