Python programming language has become increasingly popular among both beginners and experienced programmers. Thanks to its clean syntax, readability, and versatility, it has become the go-to language in various industries, including IoT. In this article, we’ll explore how you can get started with Python programming on Raspberry Pi, a powerful microcomputer that is perfect for experimenting and learning.
Getting Started with Raspberry Pi and Python
What is Raspberry Pi?
If you haven’t heard of Raspberry Pi before, it’s a credit-card sized computer that was created to promote the teaching of basic computing skills in schools and developing countries. However, its low cost and versatility have made it a popular choice among hobbyists and developers. Raspberry Pi can be used for various applications, such as media centers, game consoles, and even home automation systems.
One of the most interesting things about Raspberry Pi is that it allows you to learn and experiment with computing in a way that was not possible before. With its small size and low cost, Raspberry Pi is accessible to anyone who wants to learn about programming and electronics. You can use it to build your own projects and explore the world of computing in a hands-on way.
Why Python for Raspberry Pi?
Python is a popular programming language for Raspberry Pi because of its ease of use and versatility. You can use it for various applications, from controlling GPIO (General Purpose Input/Output) pins to building complex projects with libraries and frameworks. Python also has a large and supportive community, which means that you can find plenty of resources and help online.
In addition to its popularity, Python is also a great choice for beginners who are just starting to learn programming. Its simple syntax and easy-to-read code make it easy to understand and learn. This is especially important for students who are using Raspberry Pi as a learning tool.
Setting up Your Raspberry Pi
Before you start programming in Python on Raspberry Pi, you need to set it up first. You can download the Raspberry Pi operating system (Raspbian) from the official website and install it on an SD card. Once you insert the SD card and boot up your Raspberry Pi, you can configure it with the desktop interface or the terminal.
Setting up your Raspberry Pi can be a fun and rewarding experience. It allows you to learn about the different components of the computer and how they work together. You can also customize your setup to meet your specific needs and preferences.
Once you have set up your Raspberry Pi, you can start exploring the world of programming with Python. There are many resources available online to help you get started, including tutorials, forums, and communities. With a little bit of practice and experimentation, you can start building your own projects and exploring the endless possibilities of Raspberry Pi and Python.
Introduction to Python Programming
Python is a high-level, interpreted programming language that was first released in 1991. It was created by Guido van Rossum and has since become one of the most popular programming languages in the world. Python is known for its simplicity, readability, and versatility, making it an excellent choice for beginners and experienced programmers alike.
Python Basics
If you’re new to Python programming, it’s essential to learn the basics first. Python has a simple syntax that is easy to read and write. In Python, you use indentation to define blocks of code instead of curly braces like in other languages. You can also use comments to explain your code or disable certain lines of code temporarily.
Python is an object-oriented language, which means that everything in Python is an object. This includes data types, variables, and functions. Python also supports procedural programming, functional programming, and other programming paradigms.
Data Types and Variables
Python supports various data types, such as integers, floats, strings, and booleans. You can use variables to store values and manipulate them later. Python also allows you to perform arithmetic operations, string operations, and logical operations on variables.
Python is a dynamically typed language, which means that you don’t have to declare the data type of a variable before using it. Python will automatically assign the appropriate data type based on the value that you assign to the variable.
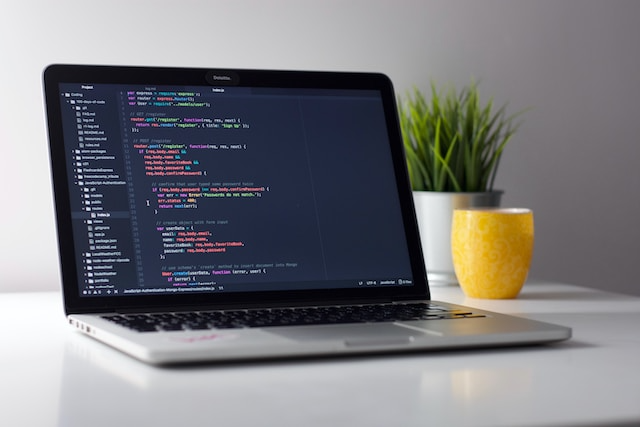
Control Structures and Loops
Control structures and loops are essential in programming because they allow you to execute code repeatedly or conditionally. Python supports if-else statements, while loops, for loops, and other logical structures that enable you to control the flow of your code.
Python also supports list comprehensions, which are a concise way of creating lists based on existing lists. List comprehensions are a powerful tool that can help you write more efficient and readable code.
Functions and Modules
Functions are reusable blocks of code that perform a specific task. In Python, you can define functions with parameters, return values, and even with default arguments. Functions are an essential part of Python programming because they allow you to break your code into smaller, more manageable pieces.
Modules, on the other hand, are files that contain Python code that you can import and use in your program. Python comes with a standard library that includes various modules that you can use. There are also many third-party modules available that you can install and use in your programs.
Python is an excellent language for scientific computing, data analysis, and machine learning. There are many libraries available for these areas, such as NumPy, Pandas, and Scikit-learn.
Python Libraries for Raspberry Pi
Raspberry Pi is a popular single-board computer that can be used for a variety of projects, from home automation to robotics. Python is a popular programming language for Raspberry Pi, and there are many Python libraries available that make it easy to control GPIO pins, interact with sensors, and build web applications. In this article, we’ll take a closer look at some of the most popular Python libraries for Raspberry Pi.
GPIO Zero Library
GPIO Zero is a Python library that allows you to control GPIO pins on Raspberry Pi easily. It provides a straightforward and intuitive API for controlling LEDs, buttons, sensors, and other input/output devices. With GPIO Zero, you can write code that is more readable and less error-prone. It’s an excellent library for beginners who want to learn about GPIO programming.
GPIO Zero is built on top of the RPi.GPIO library, which provides a low-level interface to the GPIO pins. However, GPIO Zero simplifies the interface and provides a higher-level abstraction that makes it easier to use. For example, you can control an LED with a single line of code:
from gpiozero import LEDled = LED(17)led.on()
GPIO Zero also provides support for more advanced features, such as PWM (pulse-width modulation) for controlling the brightness of LEDs and servo motors. You can even use GPIO Zero with the Raspberry Pi Camera Module to take pictures and record videos.
RPi.GPIO Library
RPi.GPIO is another popular Python library for controlling GPIO pins on Raspberry Pi. It’s a low-level library that provides more direct access to the hardware registers and the Linux kernel. RPi.GPIO is more suitable for advanced users who want to fine-tune their code and optimize it for performance.
RPi.GPIO provides a wide range of functions for controlling the GPIO pins, including setting the mode, reading and writing to the pins, and handling interrupts. Here’s an example of how to turn on an LED using RPi.GPIO:
import RPi.GPIO as GPIOGPIO.setmode(GPIO.BCM)GPIO.setup(17, GPIO.OUT)GPIO.output(17, GPIO.HIGH)
RPi.GPIO also provides support for more advanced features, such as hardware PWM and SPI (Serial Peripheral Interface) communication. However, because RPi.GPIO is a low-level library, it requires more code to accomplish simple tasks compared to GPIO Zero.
Other Useful Libraries
Apart from GPIO Zero and RPi.GPIO, there are many other Python libraries that you can use on Raspberry Pi, such as picamera for taking and recording images, sense-hat for interacting with the Sense HAT sensor board, and Flask for building web applications. With these libraries, you can explore various Raspberry Pi projects and applications.
The picamera library provides a simple interface for capturing images and videos using the Raspberry Pi Camera Module. You can control the camera settings, capture images in different formats, and even record videos with audio.
The sense-hat library provides an easy way to interact with the Sense HAT sensor board, which includes sensors for temperature, humidity, pressure, and orientation. You can use the library to read sensor data, display messages on the LED matrix, and create simple games and animations.
The Flask library is a popular web framework for Python that can be used to build web applications on Raspberry Pi. You can use Flask to create a web interface for controlling GPIO pins, displaying sensor data, and interacting with other devices on your network.
These are just a few examples of the many Python libraries available for Raspberry Pi. With the right library, you can turn your Raspberry Pi into a powerful tool for learning, experimentation, and creativity.
Building Raspberry Pi Projects with Python
Creating a Simple LED Project
Now that you know the basics of Python and GPIO programming, it’s time to build your first project. Let’s start with a simple LED project that turns an LED on and off using Python code and GPIO pins. You will need an LED, a resistor, and two jumper wires to connect the LED to the Raspberry Pi. You can follow the instructions in this tutorial to build the project and write the code.
Building a Temperature Sensor
Temperature sensors are widely used in IoT applications to monitor the temperature of a room, a building, or a device. With Raspberry Pi and Python, you can build a temperature sensor that reads the temperature from a sensor and displays it on the screen or sends it to a server. You can use the DS18B20 temperature sensor and the w1thermsensor Python library to build the project.
Developing a Home Automation System
Home automation systems allow you to control various home appliances, such as lights, heating, and security, from a central device or a mobile app. With Raspberry Pi and Python, you can build a home automation system that integrates various sensors, controllers, and devices to create a smart home. You can use MQTT, OpenHAB, or Home Assistant to build the system and control it with Python code.
In conclusion, Python programming on Raspberry Pi is an excellent way to learn about programming, electronics, and IoT. With the right resources and tools, you can build various projects and applications that showcase your skills and creativity. So, grab your Raspberry Pi and start coding!