Python, with its elegant and concise syntax, offers various ways to achieve efficient decision making. One such powerful feature is the ability to write if-else statements in a single line. Whether you want to perform a simple conditional check or evaluate multiple conditions, Python’s one-line if-else statements provide a compact and readable solution.
In this article, we’ll delve into the world of one-line if-else statements in Python. We’ll explore how to write one-line if-else statements, examine the expanded version with if-elif-else, and discuss the precautions you should take when utilizing this coding technique. Along the way, we’ll provide code examples and present a helpful table to guide you through the nuances of one-line if-else statements.
So, let’s dive in and unlock the power of concise decision making with Python’s one-line if-else statements!
One Line If-Else Statements in Python
One-line if-else statements allow you to perform a quick conditional check and execute different pieces of code based on the result. The syntax follows the pattern: [true_expression] if [condition] else [false_expression]. The true_expression is executed when the condition is true, and the false_expression is executed otherwise.
To illustrate this concept, consider the following example:
python
age = 25
message = "You are an adult" if age >= 18 else "You are a minor"
print(message)
In this code snippet, we assign a value to the message variable based on the value of the age variable. If the age is greater than or equal to 18, the true_expression “You are an adult” is assigned to message. Otherwise, the false_expression “You are a minor” is assigned.
The one-line if-else statement provides a concise and readable way to handle simple conditional checks without the need for multiline if-else blocks.
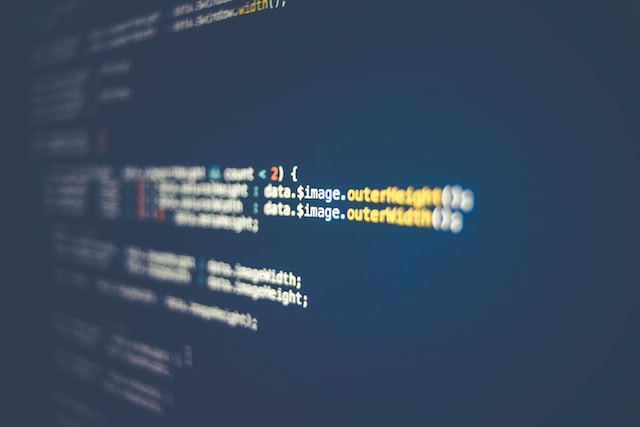
One Line If-Elif-Else in Python
Python’s one-line if-else statement can be extended to include multiple conditions using the if-elif-else structure. This allows you to evaluate different conditions sequentially and execute the corresponding code block based on the first true condition.
Let’s consider an example:
python
num = 5
classification = "Positive" if num > 0 else "Negative" if num < 0 else "Zero"
print(classification)
In this case, we use the one-line if-elif-else statement to classify the value of the num variable. If num is greater than 0, the true_expression “Positive” is assigned to the classification variable. If num is less than 0, the second condition is evaluated, and the true_expression “Negative” is assigned. Finally, if none of the previous conditions are met, the false_expression “Zero” is assigned.
The one-line if-elif-else statement provides a concise way to handle multiple conditions in a single line, reducing code clutter and enhancing readability.
Be Careful with If-Else on One Line
While one-line if-else statements offer an elegant solution for concise decision making, it’s crucial to use them judiciously and consider their impact on code readability. Here are a few precautions to keep in mind:
- Complexity and Readability: One-line if-else statements are best suited for simple conditional checks. Avoid nesting multiple conditions or complex expressions, as they can make the code hard to understand and maintain.
- Code Clarity: Strive for code clarity and maintainability. If a decision-making block becomes too long or convoluted, it’s often better to opt for a multiline if-else structure for improved readability.
- Commenting: If you find yourself using a one-line if-else statement that may not be immediately clear to others, consider adding comments to explain the logic behind the decision-making process.
By adhering to these precautions, you can leverage the power of one-line if-else statements effectively while ensuring your code remains readable and maintainable.
Code Example:
Here’s an example demonstrating the usage of a one-line if-else statement in Python:
python
num = 10
result = "Even" if num % 2 == 0 else "Odd"
print(result)
In this case, the one-line if-else statement checks if num is divisible by 2. If the condition evaluates to true, the true_expression “Even” is assigned to the result variable. Otherwise, the false_expression “Odd” is assigned.
Comparison Table: One-Line vs. Multiline If-Else
To provide a clear overview of the differences between one-line if-else statements and multiline if-else structures, let’s compare them in a table:
Criteria | One-Line If-Else Statements | Multiline If-Else Structures |
---|---|---|
Code Length | Shorter and more concise | Longer and more verbose |
Readability | Compact and easy to grasp | Potentially cluttered |
Nesting Multiple Blocks | Challenging and unreadable | Provides clear structure |
Maintenance | Simpler to modify | Easier to understand |
The table highlights the trade-offs between the two approaches, emphasizing the advantages of one-line if-else statements in terms of code length and readability, while acknowledging the benefits of multiline if-else structures in complex scenarios.
Conclusion
Python’s one-line if-else statements offer a concise and elegant way to handle decision making. Whether you need to perform a simple conditional check or evaluate multiple conditions, leveraging this feature can enhance code readability and reduce verbosity.
In this article, we explored the syntax and usage of one-line if-else statements, including the extension to if-elif-else structures. We also discussed precautions to ensure code clarity and provided a comparison table to aid your decision-making process.
By mastering the art of one-line if-else statements in Python, you can become a more efficient and expressive coder, capable of making quick decisions without compromising code readability and maintainability. Happy coding!
Add comment