In the realm of C# application development, it’s commonplace to employ if/else constructs to manage code execution paths. However, when it comes to minor operations such as modifying a variable’s value, the conventional if/else structure tends to inflate the codebase unnecessarily. Fortunately, within C#, there exists a more concise alternative: the conditional operator. Let’s delve deeper into this solution.
The Versatile Conditional Operator: A Concise Alternative to If/Else Statements
In the realm of programming, efficiency and readability are paramount. Enter the conditional operator (?:), a compact yet powerful tool that offers a succinct alternative to traditional if/else statements. Let’s delve deeper into this operator’s functionality, syntax, and practical applications.
Understanding the Syntax:
The conditional operator operates with three operands, following a simple syntax:
BooleanExpression ? expression1 : expression2
Here’s what each component entails:
- BooleanExpression: This is the condition evaluated for its truthfulness. If true, it triggers the execution of expression1; otherwise, it executes expression2;
- Expression1: The code block executed if BooleanExpression evaluates to true;
- Expression2: The code block executed if BooleanExpression evaluates to false.
Key Features and Functionality:
Inline if/else Statement: The conditional operator serves as a shorthand version of an if/else statement, condensing logic into a single line of code.
- Binary Decision Making: It facilitates binary decision-making by executing one of two expressions based on the evaluation of a Boolean condition;
- Conciseness: Compared to traditional if/else statements, the conditional operator offers brevity and clarity, reducing the amount of code needed to convey the same logic;
- Mutual Exclusivity: With the conditional operator, only one expression is executed, ensuring mutual exclusivity between expression1 and expression2.
Comparing with If/Else Statements:
While akin to an if/else statement, the conditional operator exhibits distinct characteristics:
- Length and Readability: The conditional operator streamlines code, making it more concise and easier to comprehend, particularly for simple conditional scenarios;
- Single Line Execution: Unlike if/else statements, which can encompass multiple lines of code within each block, the conditional operator strictly executes one expression per condition.
Practical Applications:
- Assigning Values: Use the conditional operator to assign values based on conditions, eliminating the need for verbose if/else blocks;
- Display Logic: Employ the operator in rendering dynamic content on user interfaces, such as displaying different messages or styles based on specific conditions;
- Validation Checks: Validate user input or system states efficiently by employing the conditional operator to execute appropriate actions;
- Error Handling: Utilize the operator for concise error handling, directing program flow based on error conditions without cluttering the code.
Navigating the Conditional Operator (?:) in Java Programming
The conditional operator (?:), also known as the ternary operator, is a fundamental construct in programming that allows for concise decision-making within code. It operates by selecting between two options, executing one of them based on a given condition. Here, we delve deeper into the intricacies of this operator and explore its applications.
Understanding the Conditional Operator (?:)
The conditional operator plays a pivotal role in streamlining code logic by offering a compact alternative to traditional if-else statements. Key characteristics and usage scenarios include:
- Binary Selection: It functions by evaluating a condition and selecting between two values;
- Efficient Decision Making: Particularly useful when a straightforward binary decision needs to be made;
- Variable Assignment: Often employed to assign values to variables based on certain conditions.
Practical Example: Consider the scenario where we need to determine the number of items to order based on whether it’s Monday:
int itemsToOrder = isMonday ? 10 : 3;
If isMonday evaluates to true, itemsToOrder is assigned the value 10.
If isMonday is false, itemsToOrder receives the value 3.
Best Practices and Considerations
When using the conditional operator, it’s essential to adhere to certain guidelines to ensure code clarity and maintainability:
- Consistent Type: The values returned by both options must be of the same type;
- Type Compatibility: Mixing incompatible types will result in compilation errors.
Example:
var value = b ? 10.23 : "example"; // Incompatible types: double and string
To address this, ensure consistency in types:
For string types:
String value = b ? "hello" : "world";
For numeric types:
double value = b ? 10.23 : 234;
Utilizing Conditional Operators in Method Execution
Conditional operators, often denoted as ?:, offer a concise means of executing methods conditionally, presenting a powerful tool in programming logic. Beyond mere variable assignment, these operators seamlessly integrate into method arguments, enhancing code readability and efficiency. Let’s delve into scenarios where this technique finds application:
1. Conditional Method Execution Based on Day of Week
Consider a scenario where you need to calculate sales data differently depending on the day of the week. Here’s how you can employ the conditional operator inline within method calls:
int kitchensSold = (DateTime.Now.DayOfWeek == DayOfWeek.Saturday) ?
WeekCount() : DayCount();
Expanded Explanation:
- Day-of-Week Logic: The conditional statement checks if the current day is Saturday;
- Method Invocation: If it’s Saturday, WeekCount() calculates the total weekly sales; otherwise, DayCount() computes daily sales;
- Enhanced Flexibility: This approach adapts dynamically to the day, ensuring accurate sales reporting.
Tips for Implementation:
- Clear Documentation: Comment on the rationale behind different calculations for different days;
- Testing and Validation: Verify the accuracy of each method under varying conditions.
2. Dynamic Appliance Requirement Determination
Imagine a situation where the number of appliances required varies based on specific conditions, such as whether a kitchen is involved. Here’s how the conditional operator can facilitate this:
Console.WriteLine(“Number of appliances needed: {0}”,
isKitchen ? ApplCount() : 0);
Expanded Explanation:
- Boolean Condition: The isKitchen variable serves as the condition for method execution;
- Method Invocation: If isKitchen evaluates to true, ApplCount() calculates the required appliances; otherwise, it returns 0;
- Efficient Resource Allocation: This technique optimizes resource usage by invoking the method only when necessary.
Navigating Complex Conditions: Mastering Multiple Conditional Operators in JavaScript
Testing multiple conditions with conditional operators (? 🙂 offers a powerful tool for evaluating various scenarios in code. By chaining these operators together, developers can efficiently handle multiple conditions and provide fallback options if none of the conditions are met. Let’s delve deeper into how this works and explore some examples.
Understanding Multiple Conditional Operators
When employing multiple conditional operators, each subsequent operator serves as a fallback in case the preceding condition evaluates to false. This cascading structure allows for the evaluation of several conditions in sequence until a true condition is found, or a default value is reached.
Syntax Overview:
firstCondition ? firstExpression :
secondCondition ? secondExpression :
thirdCondition ? thirdExpression :
fourthCondition ? fourthExpression :
defaultExpression;
Example Scenario: Handling Multiple Conditions
Let’s consider a scenario where we need to evaluate up to four possible conditions and provide a default value if none of them are true.
const result =
condition1 ? value1 :
condition2 ? value2 :
condition3 ? value3 :
condition4 ? value4 :
defaultValue;
In this setup:
If condition1 is true, value1 is returned.
If condition1 is false but condition2 is true, value2 is returned.
If neither condition1 nor condition2 are true but condition3 is true, value3 is returned.
If none of the conditions are true, defaultValue is returned.
Optimizing Code Clarity and Conciseness: Harnessing the Power of Multiple Conditional Operators
In software development, the utilization of multiple conditional operators can significantly streamline code, enhancing its readability and conciseness. Let’s delve into a practical example to elucidate this concept further.
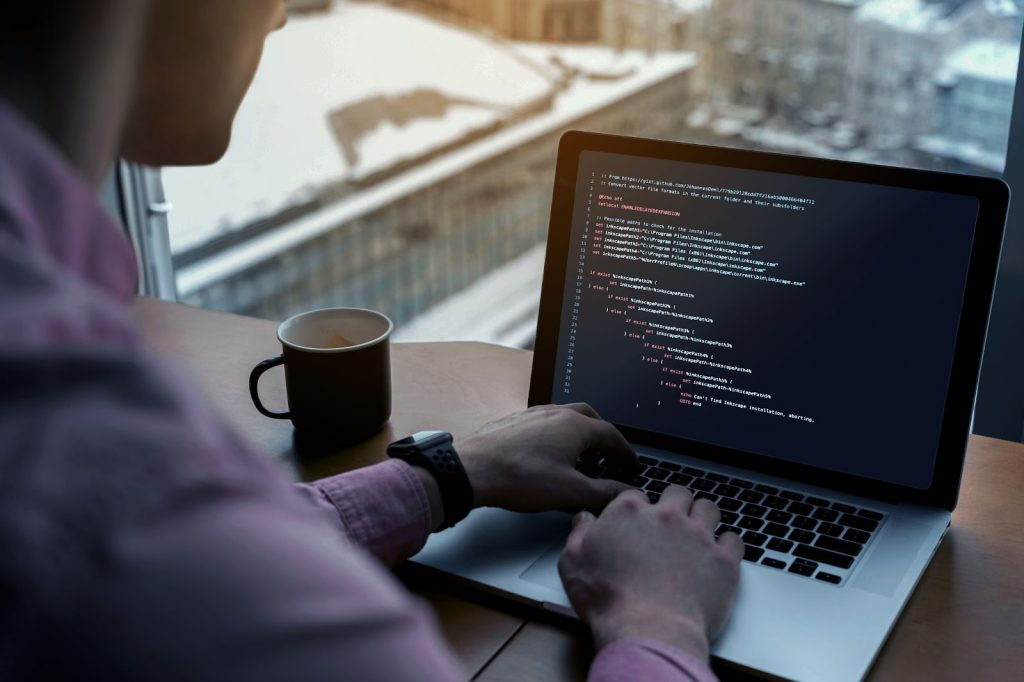
Streamlining Grading System with Multiple Conditional Operators
Consider a scenario where we need to assign letter grades to students based on their numerical scores. Instead of resorting to verbose cascaded if statements, we can leverage multiple conditional operators for a more elegant solution:
string gradeLetter = (grade <= 55) ? "F" :
(grade <= 65) ? "D" :
(grade <= 75) ? "C" :
(grade <= 85) ? "B" :
(grade <= 95) ? "A" :
"A+";
Let’s break down how this works:
- Initialization: We initialize the gradeLetter string variable;
- Conditional Tiers: The conditional operator (condition) ? true_expression : false_expression is used successively;
- For instance, if the grade is less than or equal to 55, “F” is assigned;
- If the grade is greater than 55 but less than or equal to 65, “D” is assigned, and so on;
- Final Default: If none of the previous conditions match, the default grade is set to “A+”.
Advantages of Using Multiple Conditional Operators:
- Code Conciseness: Compared to cascaded if statements, this method requires significantly less code, making it more concise and visually appealing;
- Readability: The logic is expressed in a linear, easily understandable manner, facilitating comprehension even for those new to the codebase;
- Efficiency: Execution is straightforward, with each condition being evaluated sequentially until a match is found, optimizing performance.
Considerations for Usage:
While multiple conditional operators offer clear benefits, it’s essential to weigh their appropriateness against the context:
- Complexity: Highly nested or convoluted conditional operators can obscure readability, potentially leading to maintenance challenges;
- Debugging: Identifying and rectifying issues in complex conditional logic may be time-consuming, especially for larger teams or projects;
- Team Consensus: Ensure that the chosen approach aligns with the team’s coding standards and preferences. If clarity is compromised, consider alternatives such as if/else statements or cascaded if statements.
Conclusion
In conclusion, while if/else statements are indispensable for controlling code flow in C# applications, they can lead to verbosity, especially for simple tasks like variable updates. The conditional operator offers a succinct and efficient alternative, allowing developers to streamline their code without sacrificing readability or functionality. Embracing this approach can enhance code maintainability and improve overall development efficiency in C# projects.
Add comment