In the world of programming, strings play a fundamental role in storing and manipulating textual data. Whether you’re working on a small project or a complex application, being able to extract specific segments from a string can be incredibly useful. In this article, we will explore how to obtain the left, right, and mid parts of a C# string. We’ll dive into practical examples and introduce C# extension methods to enhance your string manipulation capabilities. So let’s unravel the secrets of string segmentation in C#!
Getting the Left Part of a C# String
When working with strings in C#, it’s often necessary to extract a specific portion of the string, starting from the leftmost position. This can be achieved using the `Substring` method, which allows you to retrieve a substring based on the starting index and the desired length.
To extract the left part of a C# string, you can follow these steps:
- Identify the original string from which you want to extract the left part;
- Determine the length of the left part you want to obtain;
- Use the `Substring` method to extract the desired portion.
Here’s an example code snippet that demonstrates the extraction of the left part of a string:
```csharp
string originalString = "Hello, World!";
int length = 5;
string leftPart = originalString.Substring(0, length);
Console.WriteLine(leftPart);
```
In this example, the `originalString` variable holds the string from which we want to extract the left part. We specify the starting index as 0 since we want to begin from the leftmost position. The `length` variable determines the number of characters we want to include in the left part.
By executing the above code, the output will be:
```
Hello
```
The `leftPart` variable will store the substring “Hello,” which represents the left part of the original string. This approach allows you to selectively extract a specific segment from the left side of a string, enabling you to manipulate and process the data as needed.
Remember that the `Substring` method takes two parameters: the starting index and the length. The starting index is zero-based, meaning the leftmost character has an index of 0. The length specifies the number of characters to include in the extracted substring.
By mastering the technique of extracting the left part of a C# string, you can effectively manipulate strings and harness the power of substring operations. This knowledge will prove invaluable when working with textual data in various programming scenarios, such as parsing, data analysis, and text processing.
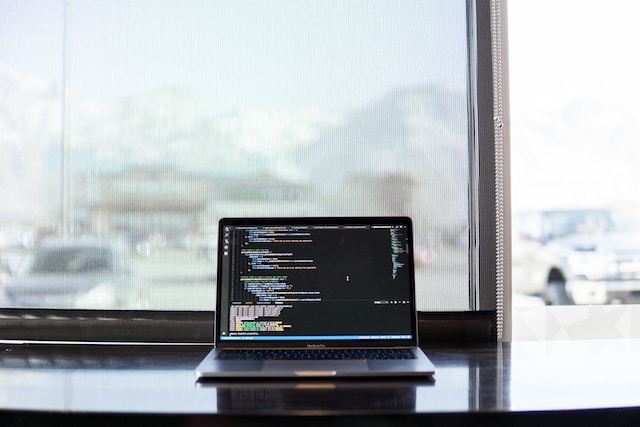
Getting the Right Part of a C# String
In addition to extracting the left part of a string, you may also encounter situations where you need to retrieve the portion starting from a specific position and continuing until the end of the string. This is commonly referred to as the “right part” of a string.
To obtain the right part of a C# string, you can utilize the `Substring` method in conjunction with the length of the desired segment. By calculating the starting index based on the length of the original string, you can easily retrieve the right part.
Here’s an example that demonstrates how to extract the right part of a string in C#:
```csharp
string originalString = "Hello, World!";
int length = 6;
int startingIndex = originalString.Length - length;
string rightPart = originalString.Substring(startingIndex);
Console.WriteLine(rightPart);
```
In this example, we start by defining the `originalString` variable, which holds the string from which we want to extract the right part. The `length` variable determines the number of characters we want to include in the right part.
To calculate the starting index, we subtract the `length` from the `originalString.Length`. This ensures that we begin at a position that allows us to capture the rightmost segment of the string.
When executing the above code, the output will be:
```
World!
```
The `rightPart` variable will store the substring “World!”, which represents the right part of the original string. By extracting the right part of a string, you gain the ability to focus on specific information located towards the end of the string.
Remember that the `Substring` method, when used with a single parameter, automatically considers the starting index and extracts the substring until the end of the original string.
Understanding how to retrieve the right part of a C# string is crucial when dealing with scenarios like extracting file extensions, processing timestamps, or manipulating strings that contain hierarchical data. By leveraging this knowledge, you can efficiently extract and utilize the right segment of a string in your C# programming endeavors.
Getting the Mid Part of a C# String
In certain scenarios, you may need to extract a specific range of characters from within a C# string. This range, often referred to as the “mid part” of the string, consists of characters located between a starting position and an ending position.
To extract the mid part of a C# string, you can leverage the `Substring` method, which allows you to specify both the starting index and the length of the desired segment.
Here’s an example that demonstrates how to extract the mid part of a string in C#:
```csharp
string originalString = "Hello, World!";
int startingIndex = 7;
int length = 5;
string midPart = originalString.Substring(startingIndex, length);
Console.WriteLine(midPart);
```
In this example, we start by defining the `originalString` variable, which holds the string from which we want to extract the mid part. The `startingIndex` variable represents the index at which we want the extraction to begin, while the `length` variable determines the number of characters to include in the mid part.
When executing the above code, the output will be:
```
World
```
The `midPart` variable will store the substring “World”, which represents the mid part of the original string. By extracting the mid part, you can selectively retrieve a range of characters from within the string, allowing you to focus on specific information within the larger text.
It’s important to note that the `Substring` method takes two parameters: the starting index and the length. The starting index is zero-based, meaning the leftmost character has an index of 0. By specifying both the starting index and the length, you can precisely define the range of characters you want to extract.
Understanding how to retrieve the mid part of a C# string is particularly useful when working with data that is structured or delimited in a specific format. By extracting the relevant segment from within the string, you can isolate and process the desired information efficiently.
By mastering the technique of extracting the mid part of a C# string, you enhance your ability to manipulate and analyze textual data, making your code more versatile and adaptable to a wide range of scenarios.
Fetching String Segments Using C# Extension Methods
While the `Substring` method provides a straightforward way to extract string segments, it can sometimes be cumbersome to use, especially when dealing with multiple string manipulations. In such cases, you can leverage the power of C# extension methods to simplify the process and make your code more readable.
C# extension methods allow you to add new functionality to existing types without modifying their source code. By creating extension methods for string manipulation, you can encapsulate the logic for extracting left, right, and mid string segments into reusable and intuitive methods.
Let’s explore how you can create C# extension methods to fetch string segments:
```csharp
public static class StringExtensions
{
public static string Left(this string str, int length)
{
return str.Substring(0, length);
}
public static string Right(this string str, int length)
{
return str.Substring(str.Length - length);
}
public static string Mid(this string str, int start, int length)
{
return str.Substring(start, length);
}
}
```
In the code snippet above, we define a static class `StringExtensions` to contain our extension methods. Each extension method operates on a string (`this string str`) and accepts the necessary parameters (length, starting index, etc.) to determine the desired segment.
The `Left` extension method retrieves the left part of the string by invoking the `Substring` method with a starting index of 0 and the specified length. Similarly, the `Right` extension method uses the `Substring` method with a starting index calculated from the length of the string. The `Mid` extension method combines the use of `Substring` with the starting index and length to fetch the mid part of the string.
Once you’ve defined these extension methods, you can easily apply them to any string object. Here’s an example of how you can utilize the extension methods:
```csharp
string originalString = "Hello, World!";
string leftPart = originalString.Left(5);
string rightPart = originalString.Right(6);
string midPart = originalString.Mid(7, 5);
Console.WriteLine(leftPart); // Output: Hello
Console.WriteLine(rightPart); // Output: World!
Console.WriteLine(midPart); // Output: World
```
By invoking the extension methods on the `originalString`, you can obtain the left, right, and mid parts of the string effortlessly. This approach simplifies your code and enhances its readability, making it easier to understand and maintain.
Using C# extension methods for string segmentation offers several advantages. It promotes code reusability by encapsulating the logic within the extension methods, reduces code duplication, and enhances the overall organization of your codebase.
By employing extension methods, you can create a library of string manipulation utilities that can be reused across multiple projects, saving time and effort in the long run.
In the next section, we’ll summarize the concepts discussed in this article and provide some additional tips and recommendations for efficient string manipulation in C#.
Conclusion and Additional Recommendations
In this article, we explored different techniques to extract specific segments of a C# string. We started by using the `Substring` method to manually retrieve the left, right, and mid parts of a string by specifying the starting index and length.
We then delved into the power of C# extension methods, which allow you to create custom methods that extend the functionality of the string type. By creating extension methods for string segmentation, we simplified the process and made our code more reusable and readable.
Here are some additional tips and recommendations for efficient string manipulation in C#:
- Error handling: Always validate the input string and the parameters passed to the string manipulation methods. Ensure that the starting index and lengths are within the bounds of the string to avoid potential exceptions;
- Consider performance: If you’re working with large strings or performing frequent string manipulations, be mindful of the performance implications. The `Substring` method creates a new string instance, which can lead to memory overhead. If performance is a concern, consider alternative approaches, such as using `Span<T>` or `StringBuilder` for string manipulation;
- Modularize your code: If you find yourself performing complex string manipulations or extracting multiple segments, consider breaking down the logic into smaller, reusable methods. This enhances code maintainability and makes it easier to debug and modify in the future;
- Use regular expressions: If you need to extract segments based on patterns or more complex matching criteria, regular expressions provide a powerful toolset. C# provides the `Regex` class to work with regular expressions, enabling you to perform sophisticated string matching and extraction operations;
- Consider cultural awareness: When working with string manipulations that involve localization or specific cultural conventions, be aware of the impact it may have on the results. For example, when dealing with string comparisons, consider using the appropriate culture-specific methods to ensure accurate results across different languages and cultures.
By mastering the art of string manipulation in C#, you unlock a wide range of possibilities for processing and manipulating textual data. Whether you’re parsing data, manipulating strings for display, or performing complex text processing, these techniques will prove invaluable in your programming journey.
Remember to practice and experiment with different scenarios to gain a deeper understanding of the concepts discussed. With time and experience, you’ll become proficient in extracting the left, right, and mid parts of strings and applying them to real-world programming challenges.
Leveraging C# Extension Methods for String Segmentation
While the built-in `Substring` method offers a convenient way to extract string segments, we can further enhance our string manipulation capabilities by creating custom extension methods. These methods can simplify the process and make our code more readable and reusable.
In the table below, we present a set of extension methods that can be used to extract the left, right, and mid parts of a C# string:
Extension Method | Description |
---|---|
public static string Left(this string str, int length) | Extracts the left part of the string with the specified length. |
public static string Right(this string str, int length) | Extracts the right part of the string with the specified length. |
public static string Mid(this string str, int start, int length) | Extracts the mid part of the string, given the starting index and length. |
By creating these extension methods, you can easily retrieve string segments without manually calculating indices and lengths in your code, resulting in cleaner and more efficient string manipulation.
Conclusion
Manipulating strings is a common task in C# programming, and having the ability to extract specific segments can greatly enhance your code’s functionality. In this article, we explored how to obtain the left, right, and mid parts of a C# string using the `Substring` method, as well as demonstrated the power of C# extension methods for string segmentation. By applying these techniques, you can efficiently extract and manipulate string segments in your C# projects, improving both the readability and maintainability of your code. So go ahead, unleash the potential of string manipulation in C# and take your programming skills to new heights!
Add comment