In the ever-evolving world of coding, developers rely on various tools and resources to streamline their workflows and create efficient, high-quality applications. One such invaluable resource that plays a fundamental role in software development is the library. If you’re new to coding or looking to expand your programming knowledge, understanding what libraries are and how they can empower your projects is crucial.
In this comprehensive guide, we will delve into the realm of libraries, exploring their purpose, usage, and significance in coding. We’ll examine how developers leverage libraries to enhance productivity, compare libraries with frameworks, delve into the process of finding suitable libraries, and provide practical examples and a handy reference table to illustrate their functionality.
How Do Developers Use Libraries?
Libraries serve as a treasure trove of pre-written code that developers can leverage to expedite the development process. They encapsulate reusable functions, classes, and modules, designed to perform specific tasks or address common programming challenges. By utilizing libraries, developers can avoid reinventing the wheel and focus on building their application’s unique features.
Developers integrate libraries into their code by importing or including them, depending on the programming language. Once imported, they gain access to a wide range of functions and functionalities that simplify complex tasks, such as handling database connections, managing user authentication, processing data, or creating intuitive user interfaces. Libraries can significantly enhance development efficiency, saving developers precious time and effort.
Can You Code Without Libraries?
While it is technically possible to code without libraries, doing so would be akin to embarking on a perilous journey without a map or compass. Libraries provide developers with a wealth of resources and solutions that have been tried, tested, and optimized over time. They offer battle-tested algorithms, robust data structures, and specialized tools that can accelerate development and improve the quality of the final product.
By forgoing the use of libraries, developers would need to invest considerable effort in reinventing existing solutions, resulting in wasted time and increased complexity. Libraries, with their extensive functionality, not only save developers from unnecessary coding but also facilitate collaboration, as they provide a standardized way to achieve common goals.
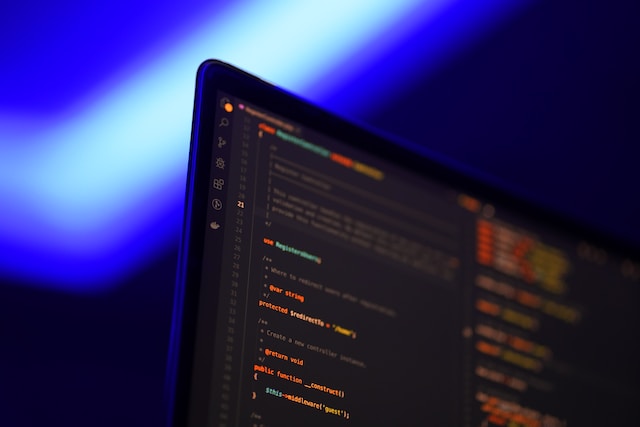
Library vs Framework
Although libraries and frameworks are both valuable tools in the coding world, it’s essential to understand their distinctions and choose the right approach for your projects. Let’s delve deeper into the comparison between libraries and frameworks:
3.1 Flexibility vs. Structure:
Libraries grant developers flexibility, allowing them to pick and choose specific functions or components to incorporate into their codebase. They serve as a collection of tools that can be used independently, providing developers with freedom and versatility in designing their application architecture. Developers have control over how they integrate and utilize libraries, enabling them to tailor the functionality according to their specific requirements.
On the other hand, frameworks offer a more structured approach. They provide a complete architecture and a set of rules that guide the development process. Frameworks often have an opinionated approach, enforcing a specific way of designing and organizing code. While this may restrict flexibility, it simplifies decision-making, promotes code consistency, and streamlines development by eliminating the need to make extensive choices at every step.
3.2 Development Speed and Learning Curve:
Libraries excel at accelerating development speed. By utilizing pre-existing code and functionalities, developers can bypass the need to implement complex features from scratch. Libraries are often designed to address specific tasks or challenges, enabling developers to leverage their expertise and save time. They serve as time-tested solutions that have been refined and optimized over time by a community of developers.
Frameworks, while providing a higher learning curve initially, can expedite development in the long run. By offering a predefined structure, frameworks eliminate the need for developers to make overarching design decisions. This allows them to focus on implementing the unique features of their application rather than spending time on setting up foundational components. Frameworks also provide conventions and best practices, making it easier for developers to collaborate and maintain codebases as projects grow.
3.3 Control and Customization:
Libraries give developers more control over their codebase. They can select specific libraries based on their needs and incorporate them seamlessly into their projects. This granular control allows developers to fine-tune functionalities, optimize performance, and integrate libraries into existing codebases without requiring significant changes.
Frameworks, while limiting control in certain aspects, provide a cohesive structure that ensures consistency and promotes code reuse. They often come with built-in components, such as routing systems, database abstraction layers, and authentication modules, which simplify the development process. Frameworks may also offer extensive customization options within the established framework boundaries, allowing developers to tailor the application to their specific requirements.
3.4 Learning Curve and Dependency Management:
When it comes to the learning curve, libraries typically have a shallower curve as developers can pick and choose specific functionalities they need. Learning individual libraries is usually less time-consuming than mastering an entire framework, as developers can focus on understanding and utilizing specific functions or modules. However, developers may need to manage multiple dependencies when utilizing multiple libraries in a project, which requires careful version control and dependency management.
Frameworks, on the other hand, often require developers to invest time in understanding the entire framework’s architecture, conventions, and concepts. This learning curve can be steeper but can pay off in the long run, especially for larger projects or teams where a standardized structure and workflow are crucial. Frameworks often come bundled with the necessary dependencies and handle dependency management internally, making it easier for developers to maintain consistent versions.
Ultimately, the choice between libraries and frameworks depends on the specific project requirements, development goals, and personal preferences. Some projects may benefit from the flexibility and customization options offered by libraries, while others may thrive with the structure and conventions provided by frameworks. As a developer, it’s important to evaluate the trade-offs and make informed decisions based on the project’s unique needs.
How to Find Libraries?
With the vast array of libraries available, finding the right one for your project can be a daunting task. However, several resources and strategies can help simplify the process. Here are some additional methods for discovering libraries:
- Social Media and Developer Communities: Engaging with coding communities on social media platforms like Twitter, LinkedIn, and Facebook can provide valuable insights into popular libraries and emerging trends. Join relevant groups, follow influential developers, and participate in discussions to gain recommendations and stay updated on the latest library releases;
- Online Tutorials and Blogs: Many programming tutorials and blogs feature recommendations for libraries and provide step-by-step guides on their usage. Exploring platforms like Medium, Dev.to, and YouTube can lead you to informative articles, videos, and tutorials that highlight libraries and their practical application;
- GitHub: As a vast repository of open-source projects, GitHub is an excellent resource for discovering libraries. Utilize GitHub’s search functionality to find repositories related to your project’s programming language or topic of interest. Explore the repository’s documentation, star ratings, and community engagement to assess the library’s popularity and reliability;
- Tech Conferences and Events: Attending conferences, workshops, and meetups dedicated to your programming language or specific domains can expose you to new libraries and innovative solutions. Networking with fellow developers and attending talks or presentations can provide valuable insights and recommendations;
- Language-Specific Resources: Many programming languages have dedicated websites, forums, or resources that curate and showcase popular libraries. For example, the official Python website hosts the Python Package Index (PyPI), a comprehensive catalog of Python libraries. Explore language-specific resources to find curated lists, community-recommended libraries, and specialized tools;
- Ask Peers and Mentors: Reach out to experienced developers, mentors, or colleagues who have worked on similar projects or possess domain expertise. Seeking their advice and recommendations can help you discover libraries that have been proven effective in real-world scenarios.
Remember, when searching for libraries, consider factors such as the library’s popularity, community support, documentation quality, active development, and compatibility with your programming language and project requirements. It’s also important to review the library’s licensing terms to ensure they align with your project’s needs and any potential restrictions.
By combining multiple methods and resources, you can broaden your search for libraries and increase your chances of finding the most suitable ones for your projects. Don’t hesitate to experiment, evaluate, and iterate on different libraries to identify the ones that best meet your development needs.
Practical Example and Reference Table
To illustrate the practical implementation of libraries, let’s consider a common scenario: image processing. Suppose you are building an image manipulation application. Instead of manually implementing complex algorithms for resizing, cropping, or applying filters, you can leverage libraries like OpenCV, Pillow, or GraphicsMagick. These libraries offer a range of functions and tools for image processing, drastically simplifying your development process.
Here’s an example of using the OpenCV library in Python to resize an image:
python
import cv2
# Read the image file
image = cv2.imread('input_image.jpg')
# Resize the image
resized_image = cv2.resize(image, (800, 600))
# Save the resized image
cv2.imwrite('output_image.jpg', resized_image)
Reference Table: Image Processing Libraries
Library | Language | Features |
---|---|---|
OpenCV | Python | Comprehensive image processing and computer vision library |
Pillow | Python | Image manipulation and processing library |
GraphicsMagick | Various | Powerful image processing and conversion tool |
Conclusion
Libraries are a fundamental resource in coding, empowering developers by providing ready-to-use code and efficient solutions to common programming challenges. By utilizing libraries, developers can expedite their development process, improve code quality, and collaborate more effectively. Understanding the role and significance of libraries, along with their distinction from frameworks, is essential for any aspiring or experienced programmer.
Remember to explore online communities, package managers, and official documentation to find the most suitable libraries for your projects. By harnessing the power of libraries, you can unlock new possibilities and elevate your coding skills to new heights. So, dive in, experiment, and embrace the vast world of libraries to supercharge your coding journey.
Add comment