Exponents play a crucial role in mathematical computations, allowing us to raise numbers to specific powers. In the realm of Python programming, harnessing the power of exponents opens up a world of possibilities. Whether you’re working on simple calculations or complex algorithms, understanding how to manipulate exponents in Python is essential.
In this comprehensive guide, we will delve into the fascinating world of exponents in Python. We will explore different methods to calculate exponents, such as the ** operator, pow() function, and the versatile math library. Additionally, we’ll provide practical examples to illustrate these techniques and show you how to process lists or arrays, leverage list comprehensions, and use for loops for exponentiation.
So, let’s dive into the intricacies of exponents in Python and equip ourselves with the knowledge to perform efficient and accurate calculations.
Calculate Exponents in Python using the ** Operator
The ** operator in Python is a straightforward and efficient way to calculate exponents. It allows you to raise a number to a specific power with a concise syntax. Let’s delve deeper into this operator and explore its functionalities.
Syntax:
To raise a number to a power using the ** operator, the syntax is as follows:
```python
base ** exponent
```
Here, the “base” represents the number you want to raise to a power, and the “exponent” indicates the power to which you want to raise the base.
Precedence Rules:
In Python, the ** operator has the highest precedence, meaning it is evaluated first in an expression. However, you can use parentheses to enforce a specific order of operations if needed.
Code Examples:
Let’s look at a few examples to see the ** operator in action:
```python
# Example 1: Basic exponentiation
result = 2 ** 3
print(result) # Output: 8
# Example 2: Negative exponent
result = 4 ** -2
print(result) # Output: 0.0625
# Example 3: Decimal exponent
result = 5 ** 0.5
print(result) # Output: 2.23606797749979
```
In the first example, we calculate 2 raised to the power of 3, resulting in 8. The second example demonstrates using a negative exponent, which is equivalent to taking the reciprocal of the base raised to the positive exponent. Finally, the third example shows how the ** operator can handle decimal exponents, yielding the square root of the base.
Tips and Best Practices:
Here are some tips and best practices to keep in mind when using the ** operator for exponentiation in Python:
- Understand operator precedence: As mentioned earlier, the ** operator has the highest precedence. However, it’s always a good practice to use parentheses when working with complex expressions to ensure the desired order of operations;
- Be cautious with large exponents: When dealing with very large exponents, the ** operator might result in extremely large numbers or even cause memory errors. Consider alternative approaches, such as using logarithms or specialized libraries, when working with massive exponentiation operations;
- Integers vs. floating-point numbers: Depending on the input types, the ** operator can produce different results. For integer inputs, the ** operator performs integer exponentiation, while for floating-point inputs, it returns a float value;
- Consider modular exponentiation: In certain scenarios, such as cryptography or working with large numbers, modular exponentiation can be more efficient. Python provides the pow() function with an optional modulus parameter for such cases.
By familiarizing yourself with the ** operator and keeping these best practices in mind, you can confidently perform exponentiation operations in Python efficiently and accurately.
Next, let’s explore another method for calculating exponents in Python using the pow() function.
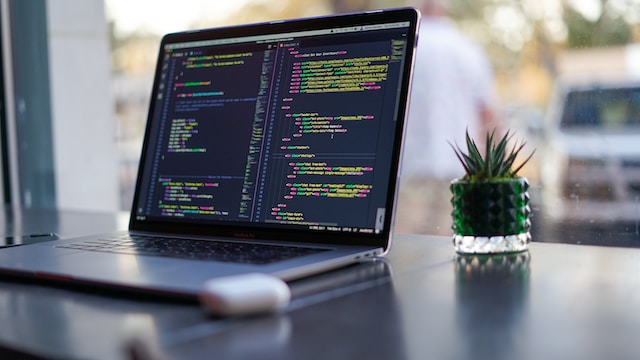
Calculate Python Exponents with the pow() Function
While the ** operator provides a convenient way to calculate exponents in Python, the pow() function offers an alternative method with added flexibility. The pow() function allows you to raise a number to a specific power and also supports modular exponentiation. Let’s delve into the details of this function and explore its capabilities.
Syntax:
The syntax for using the pow() function is as follows:
```python
pow(base, exponent[, modulus])
```
Here, the “base” parameter represents the number you want to raise to a power, the “exponent” parameter indicates the power to which you want to raise the base, and the optional “modulus” parameter defines the modulus value for modular exponentiation.
Code Examples:
Let’s take a look at some examples to understand how to use the pow() function for exponentiation:
```python
# Example 1: Basic exponentiation
result = pow(2, 3)
print(result) # Output: 8
# Example 2: Modular exponentiation
result = pow(3, 4, 5)
print(result) # Output: 1
# Example 3: Floating-point exponent
result = pow(4.5, 2)
print(result) # Output: 20.25
```
In the first example, we calculate 2 raised to the power of 3 using the pow() function, resulting in 8. The second example demonstrates modular exponentiation by calculating 3 raised to the power of 4, with a modulus of 5. In this case, the result is 1 since 3^4 is congruent to 1 modulo 5. The third example showcases how the pow() function can handle floating-point exponents, returning 20.25 as the result of 4.5 raised to the power of 2.
Modular Exponentiation:
Modular exponentiation is particularly useful in cryptographic algorithms and scenarios involving large numbers. It allows you to calculate the remainder when a number is raised to a power modulo another number. By specifying the modulus parameter in the pow() function, you can easily perform modular exponentiation in Python.
Here’s an example that illustrates modular exponentiation using the pow() function:
```python
# Example: Modular exponentiation
result = pow(2, 10, 7)
print(result) # Output: 4
```
In this example, we calculate 2 raised to the power of 10 modulo 7, which results in 4. The remainder of 2^10 divided by 7 is 4.
Tips and Best Practices:
Consider the following tips and best practices when using the pow() function for exponentiation in Python:
- Utilize the modulus parameter: The pow() function’s modulus parameter is optional but highly valuable when working with modular exponentiation. It allows you to perform computations efficiently and handle large numbers effectively;
- Leverage the ** operator for simple exponents: If you’re dealing with basic exponentiation without the need for modular calculations, the ** operator might offer a more concise and readable solution compared to the pow() function;
- Be aware of performance implications: In some cases, using the ** operator might be faster than the pow() function, especially for small integer exponents. Consider your specific use case and performance requirements when choosing between the ** operator and the pow() function.
By understanding the syntax, capabilities, and best practices of the pow() function, you can confidently calculate exponents in Python, both for standard exponentiation and modular operations. Now, let’s move on to exploring another powerful method for exponentiation using Python’s math library.
Raise Numbers to a Power with Python’s math.pow()
When it comes to advanced mathematical operations, Python’s math library provides a wide range of functions to enhance your exponentiation capabilities. One such function is math.pow(), which offers precise floating-point exponentiation. In this section, we will delve into the details of math.pow() and explore its usage through practical examples.
Syntax:
To use the math.pow() function for exponentiation, the syntax is as follows:
```python
math.pow(base, exponent)
```
Here, the “base” parameter represents the number you want to raise to a power, and the “exponent” parameter indicates the power to which you want to raise the base.
Code Examples:
Let’s explore some examples to illustrate the usage of math.pow() for exponentiation:
```python
import math
# Example 1: Basic exponentiation
result = math.pow(2, 3)
print(result) # Output: 8.0
# Example 2: Floating-point exponent
result = math.pow(4.5, 2)
print(result) # Output: 20.25
```
In the first example, we calculate 2 raised to the power of 3 using math.pow(), which results in 8.0 as the output. The second example showcases how math.pow() handles floating-point exponents, returning 20.25 as the result of 4.5 raised to the power of 2.
Advantages of math.pow():
Python’s math.pow() function offers several advantages when compared to other exponentiation methods:
- Precise floating-point calculations: math.pow() provides accurate results for floating-point exponentiation, ensuring precise calculations even for complex operations involving decimal powers;
- Consistency with mathematical conventions: The math.pow() function aligns with standard mathematical notation, making it easier to understand and use when working with mathematical equations and formulas;
- Seamless integration with the math library: math.pow() is part of the math module, which offers a comprehensive set of mathematical functions. Leveraging math.pow() allows you to harness the full power of the math library in your exponentiation tasks;
- Support for advanced mathematical functions: The math library provides additional mathematical functions that can be combined with math.pow() for more advanced computations. These functions include logarithms, trigonometric functions, and more.
It’s worth noting that while math.pow() excels in floating-point exponentiation, it might not be the most efficient choice for large integer exponents or modular calculations. In such cases, alternative methods like the ** operator or the pow() function with a modulus parameter might be more suitable.
By leveraging the precision and flexibility of math.pow(), you can perform accurate and sophisticated exponentiation operations in Python. Now, let’s explore how to process a list or array and calculate exponents for each value in Python.
Process a List or Array: Calculate the Exponent for Each Value
In many scenarios, you may encounter the need to calculate exponents for multiple values stored in a list or array. Python provides powerful techniques to efficiently process such collections and perform exponentiation for each value. In this section, we will explore different methods to calculate the exponent for each value in a list or array.
Method 1: List Comprehensions
List comprehensions offer a concise and elegant way to apply exponentiation to each element in a list or array. The general syntax for using list comprehensions for exponentiation is as follows:
```python
exponentiated_list = [base ** exponent for base in original_list]
```
Here, “original_list” represents the input list or array, “base” is a placeholder for each value in the original list, and “exponent” represents the desired power to raise each base to. The resulting “exponentiated_list” will contain the exponentiated values.
Let’s see an example to understand how list comprehensions can be used for exponentiation:
```python
# Example:
numbers = [2, 3, 4, 5]
exponentiated_numbers = [num ** 2 for num in numbers]
print(exponentiated_numbers) # Output: [4, 9, 16, 25]
```
In this example, we have a list of numbers [2, 3, 4, 5]. By using a list comprehension with the ** operator, we raise each number to the power of 2, resulting in the exponentiated list [4, 9, 16, 25].
Method 2: Using a for Loop
An alternative approach to calculate exponents for each value in a list or array is by using a for loop. This method provides more flexibility and control over the exponentiation process. Here’s the general structure of using a for loop for exponentiation:
```python
exponentiated_list = []
for base in original_list:
exponentiated_value = base ** exponent
exponentiated_list.append(exponentiated_value)
```
In this structure, “original_list” represents the input list or array, “base” iterates through each value in the list, “exponent” represents the desired power, and “exponentiated_list” stores the resulting exponentiated values.
Let’s look at an example that demonstrates the use of a for loop for exponentiation:
```python
# Example:
numbers = [2, 3, 4, 5]
exponentiated_numbers = []
for num in numbers:
exponentiated_value = num ** 2
exponentiated_numbers.append(exponentiated_value)
print(exponentiated_numbers) # Output: [4, 9, 16, 25]
```
In this example, we iterate through each number in the list [2, 3, 4, 5] using a for loop. For each number, we calculate the square by raising it to the power of 2, and the resulting values are stored in the exponentiated_numbers list.
Both list comprehensions and for loops provide efficient ways to process lists or arrays and calculate exponents for each value. The choice between the two methods depends on your preference, coding style, and the complexity of the exponentiation logic.
Next, let’s explore how list comprehensions and for loops can be utilized to perform exponentiation and create powerful tables or visualizations.
Exponentiate List Values with Python’s List Comprehension
Python’s list comprehensions offer a powerful and concise way to perform operations on list elements, including exponentiation. By leveraging list comprehensions, you can easily exponentiate each value in a list and create a new list with the resulting exponentiated values. In this section, we will explore how to use list comprehensions for exponentiation and showcase their effectiveness.
Syntax:
The syntax for using list comprehensions to exponentiate list values is as follows:
```python
exponentiated_list = [base ** exponent for base in original_list]
```
Here, “original_list” represents the input list containing the values to be exponentiated, “base” is a placeholder for each element in the original list, and “exponent” indicates the desired power to raise each base to. The resulting “exponentiated_list” will contain the exponentiated values.
Code Examples:
Let’s take a look at a few examples to illustrate how list comprehensions can be used for exponentiation:
```python
# Example 1: Exponentiate values in a list
numbers = [2, 3, 4, 5]
exponentiated_numbers = [num ** 2 for num in numbers]
print(exponentiated_numbers) # Output: [4, 9, 16, 25]
# Example 2: Exponentiate using a different exponent
numbers = [1, 2, 3, 4, 5]
exponentiated_numbers = [num ** 3 for num in numbers]
print(exponentiated_numbers) # Output: [1, 8, 27, 64, 125]
```
In the first example, we have a list of numbers [2, 3, 4, 5]. By using a list comprehension with the ** operator, we exponentiate each number to the power of 2, resulting in the exponentiated list [4, 9, 16, 25]. In the second example, we use the same list of numbers but exponentiate them to the power of 3, yielding the list [1, 8, 27, 64, 125].
Advantages of List Comprehensions:
List comprehensions offer several advantages when exponentiating list values:
1. Concise and readable code: List comprehensions provide a compact and expressive syntax, making the code more readable and reducing the lines of code required for exponentiation operations.
2. Efficiency and performance: List comprehensions are optimized for speed and efficiency, allowing you to process large lists quickly. They leverage Python’s built-in mechanisms for performance, making them an efficient choice for exponentiating list values.
3. Flexibility and versatility: List comprehensions can be easily customized to fit different scenarios. You can incorporate conditionals and other operations within the comprehension to perform complex calculations and transformations on list elements.
4. Seamless integration with other Python constructs: List comprehensions work seamlessly with other Python constructs, such as conditional statements, nested loops, and function calls. This flexibility allows you to combine exponentiation with other operations or data manipulations effortlessly.
By harnessing the power of list comprehensions, you can efficiently exponentiate list values and create new lists containing the resulting exponentiated values. This technique is especially useful when dealing with large datasets or when you need to transform a list of values based on specific exponentiation requirements.
Next, let’s explore how a for loop can be utilized to raise values to a power and perform exponentiation in Python.
Raise Values to a Power with Python’s for Loop
For more complex scenarios that require fine-grained control over the exponentiation process, a for loop can be an excellent choice. In this section, we’ll walk you through the process of using a for loop to raise values to a specific power. We’ll provide detailed examples, discuss optimizations, and highlight scenarios where a for loop can be particularly advantageous.
Conclusion:
Exponents are an essential mathematical concept that finds extensive applications in various fields. Python provides multiple tools and techniques to calculate exponents efficiently, catering to different use cases. In this article, we explored the ** operator, pow() function, math library, list comprehensions, and for loops.
for exponentiation in Python. Armed with this knowledge, you can tackle a wide range of exponent-related tasks, from simple calculations to complex algorithms.
Remember to leverage the appropriate technique based on your specific requirements and optimize your code for efficiency. By mastering exponents in Python, you unlock the potential to solve intricate problems, analyze data, and build powerful applications. So, embrace the power of exponents and elevate your Python programming skills to new heights.
Add comment