Arduino, an open-source electronics platform, has revolutionized the world of DIY electronics and automation. Its flexibility, ease of use, and vast community support have made it a go-to choice for hobbyists, enthusiasts, and professionals alike. At the core of this innovative platform lies Arduino code, which empowers users to control various electronic components and create interactive projects with limitless possibilities.
In this comprehensive guide, we will explore the intricacies of Arduino code, unraveling its features, functionalities, and practical applications. Whether you’re a beginner taking your first steps in the world of Arduino or an experienced coder looking to enhance your skills, this article will equip you with the knowledge and tools needed to harness the full potential of Arduino.
Arduino Coding Environment and Basic Tools
The Arduino Coding Environment provides a user-friendly interface that simplifies the process of writing, compiling, and uploading code to your Arduino board. Let’s delve deeper into its various components and basic tools that make coding a breeze.
1. Integrated Development Environment (IDE):
The Arduino IDE is the central hub of the coding environment. It is a software application that allows you to write and manage your Arduino sketches. The IDE supports multiple operating systems and provides a consistent platform for beginners and experienced users alike.
2. Code Editor:
Within the Arduino IDE, you’ll find a powerful code editor that offers features like syntax highlighting, auto-completion, and error checking. These features enhance the coding experience, making it easier to write clean and error-free code.
3. Sketch:
In Arduino, a sketch is the term used for a program written for the Arduino board. It consists of two primary functions: setup() and loop(). The setup() function runs once when the board starts up, allowing you to initialize variables, configure pins, and perform any necessary setup tasks. The loop() function, on the other hand, runs continuously after the setup() function and forms the core of your program’s functionality.
4. Serial Monitor:
The Serial Monitor is a valuable tool for debugging and communication. It allows you to send and receive data between the Arduino board and your computer through the serial port. This is particularly useful when you want to monitor sensor readings, debug code, or establish two-way communication with your Arduino project.
5. Board and Port Selection:
Before uploading your code to the Arduino board, you need to ensure that the correct board and serial port are selected in the IDE. The board selection determines the specific Arduino model you’re using, while the port selection establishes the communication between your computer and the Arduino board.
6. Examples and Tutorials:
The Arduino IDE comes bundled with a plethora of examples and tutorials that cover a wide range of functionalities. These examples serve as a great starting point for beginners, offering hands-on experience with different sensors, actuators, and communication protocols. They provide valuable insights into the syntax and structure of Arduino code, helping you grasp key concepts quickly.
7. Version Control:
As your Arduino projects grow in complexity, it becomes essential to manage different versions of your code. The Arduino IDE supports version control systems like Git, enabling you to track changes, collaborate with others, and revert to previous versions if needed.
By familiarizing yourself with the Arduino Coding Environment and its basic tools, you’ll gain the confidence to start coding your own projects. Remember, practice makes perfect, so don’t hesitate to experiment and explore the vast possibilities that Arduino code offers. In the next section, we’ll dive into the structure of Arduino code, laying the foundation for creating robust and efficient programs.
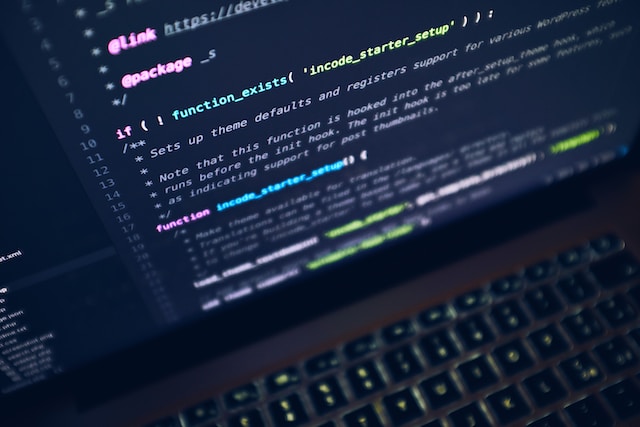
Code Structure
Understanding the structure of Arduino code is crucial for effectively programming your Arduino board. Let’s explore the different elements and concepts that form the building blocks of Arduino code.
1. Preprocessor Directives:
At the beginning of your Arduino sketch, you may include preprocessor directives, which provide instructions to the compiler before the code is compiled. These directives start with the ‘#’ symbol and are used to define constants, include libraries, or set configuration options. For example, the ‘#include’ directive is used to include external libraries in your code.
2. Setup() Function:
The setup() function is called only once when the Arduino board starts up or is reset. It is typically used to initialize variables, set pin modes, configure communication interfaces, and perform any necessary setup tasks. Anything defined within the setup() function will be executed at the beginning of your program.
3. Loop() Function:
The loop() function is the heart of your Arduino code. Once the setup() function has completed, the loop() function runs continuously, repeatedly executing the code within its curly braces. This allows your program to perform tasks in a repetitive manner, making it ideal for reading sensors, controlling actuators, and monitoring inputs.
4. Variables and Data Types:
Arduino code involves working with variables to store and manipulate data. Variables hold different types of data, such as integers, floating-point numbers, characters, and Boolean values. Arduino supports several data types, including int, float, char, bool, and more. Understanding data types is essential for memory management and performing mathematical operations.
5. Control Structures:
Control structures in Arduino code allow you to make decisions and control the flow of your program. Common control structures include:
- If-Else Statements: These statements are used for conditional execution. They check a condition and execute specific blocks of code based on whether the condition is true or false. If the condition is true, the code within the if block is executed; otherwise, the code within the else block is executed;
- Loops: Loops enable you to execute a block of code repeatedly. The two primary loop structures in Arduino code are the ‘for’ loop and the ‘while’ loop. The ‘for’ loop repeats a block of code for a specified number of times, while the ‘while’ loop repeats a block of code as long as a given condition is true.
6. Functions:
Functions are reusable blocks of code that perform specific tasks. They allow you to modularize your code, improve readability, and promote code reusability. Arduino provides built-in functions, such as pinMode(), digitalWrite(), and analogRead(), which simplify common tasks. You can also create your own custom functions to encapsulate complex operations and enhance the organization of your code.
By understanding the structure of Arduino code, you’ll be able to write clean, well-organized programs that are easy to maintain and understand. In the next section, we’ll delve into the process of programming an Arduino board, guiding you through the essential steps to bring your code to life on the physical hardware.
How to Program Arduino
Programming an Arduino board involves a step-by-step process that combines writing code, setting up hardware connections, and uploading the code to the board. In this section, we’ll guide you through the essential steps of programming an Arduino, empowering you to bring your projects to life.
1. Hardware Setup:
Before you begin programming, ensure that you have the necessary hardware components and connections in place. This typically involves connecting sensors, actuators, LEDs, and other electronic components to specific pins on the Arduino board. Refer to the documentation or datasheets of your components to identify the correct pins and wiring configurations. Double-check your connections to avoid any potential issues later on.
2. Launch Arduino IDE:
Launch the Arduino IDE on your computer. If you haven’t installed it yet, you can download the IDE from the Arduino website. Once the IDE is open, you’ll see a blank sketch ready for you to start writing code.
3. Writing Code:
Begin by writing the code that defines the behavior of your Arduino project. Utilize the concepts and structures we discussed in Section 2, such as variables, data types, control structures, and functions. You can start with simple examples provided by the Arduino IDE or build your code from scratch.
4. Verify and Compile:
After writing your code, click on the “Verify” button (checkmark icon) in the Arduino IDE. This step compiles your code and checks for any syntax errors or compilation issues. If there are errors, review the error messages in the console and fix them accordingly. It’s crucial to address these errors before proceeding to the next step.
5. Select Board and Port:
Before uploading the code to your Arduino board, ensure that the correct board is selected in the Arduino IDE. Navigate to the “Tools” menu and select the appropriate board model from the “Board” submenu. Additionally, choose the correct port to establish communication between your computer and the Arduino board.
6. Upload Code:
With the correct board and port selected, you’re ready to upload your code to the Arduino board. Click on the “Upload” button (right arrow icon) in the Arduino IDE. The IDE will compile your code again and then upload it to the Arduino board. A progress bar will indicate the status of the upload process. Once the upload is complete, you’ll see a “Done uploading” message in the IDE.
7. Serial Monitor:
To interact with your Arduino project and monitor its behavior, you can utilize the Serial Monitor. In the Arduino IDE, click on the magnifying glass icon in the top-right corner to open the Serial Monitor. It allows you to send and receive data between your Arduino board and the computer. You can use the Serial Monitor to display sensor readings, debug your code, or communicate with external devices.
8. Testing and Iteration:
After uploading your code, test your Arduino project to ensure that it behaves as expected. Monitor the output in the Serial Monitor, observe the behavior of connected components, and make any necessary adjustments to your code. Arduino programming often involves an iterative process of testing, refining, and improving the code until the desired functionality is achieved.
By following these steps, you’ll be able to program your Arduino board effectively, unleashing its capabilities and turning your ideas into reality. In the next section, we’ll explore Arduino code libraries, which can significantly expand the functionality of your projects by providing ready-to-use code snippets and functions.
Arduino Code Libraries
Arduino code libraries are pre-written sets of code that provide ready-to-use functions, classes, and examples. These libraries simplify complex tasks, save time, and expand the capabilities of your Arduino projects. In this section, we’ll explore Arduino code libraries and guide you through the process of installing and utilizing them effectively.
1. What are Arduino Libraries?
Arduino libraries are collections of code that encapsulate specific functionalities and provide a standardized interface to interact with external components or perform complex tasks. These libraries are created and shared by the Arduino community, allowing users to leverage the expertise and contributions of others. Libraries can range from simple ones that handle basic tasks to comprehensive libraries that support advanced functionalities like communication protocols, displays, sensors, and more.
2. Finding and Installing Libraries:
To find libraries for your Arduino projects, you can visit the official Arduino Library Manager or explore external repositories such as GitHub. The Arduino Library Manager, accessible from the “Sketch” menu in the Arduino IDE, provides a convenient way to search for libraries, view their descriptions, and install them with a few clicks. Alternatively, you can manually download libraries and install them by placing them in the “libraries” folder within your Arduino sketchbook directory.
3. Popular Arduino Libraries:
There is a vast collection of Arduino libraries available, catering to various needs and requirements. Here are a few popular libraries widely used in Arduino projects:
- Servo Library: The Servo library allows you to control servo motors, enabling precise positioning and movement in robotics and automation projects;
- Wire Library (I2C): The Wire library facilitates communication over the I2C (Inter-Integrated Circuit) protocol, enabling you to interface with I2C devices such as sensors, displays, and EEPROMs;
- Adafruit NeoPixel Library: This library simplifies the control of NeoPixel RGB LEDs, enabling you to create stunning lighting effects and animations;
- Ethernet Library: The Ethernet library provides a set of functions to establish network connections and communicate with Ethernet-enabled devices, enabling IoT applications and web connectivity;
- DHT Library: The DHT library simplifies working with DHT series temperature and humidity sensors, providing convenient functions to read sensor data accurately.
4. Utilizing Libraries:
Once you have installed a library, you can include it in your Arduino sketch using the ‘#include’ directive. This makes the library’s functions and classes available for use within your code. Refer to the library’s documentation or examples to understand how to utilize its functionalities effectively. By leveraging libraries, you can significantly reduce the development time and focus on implementing higher-level logic specific to your project.
5. Contributing to Libraries:
The Arduino community thrives on collaboration and contributions. If you have expertise in a specific domain or have created a useful set of code, consider sharing it as an Arduino library. Contributing to libraries not only helps others but also adds value to the community as a whole.
By exploring and utilizing Arduino code libraries, you can enhance the functionality of your projects, leverage existing code, and speed up development. Libraries provide a wealth of resources, examples, and standardized interfaces, enabling you to focus on the unique aspects of your project. In the next section, we’ll delve into the versatility of Arduino as a platform, exploring its applications in various domains and industries.
Arduino: An Extremely Versatile Platform
Arduino has gained immense popularity due to its versatility and adaptability across a wide range of applications. In this section, we’ll explore the diverse domains and industries where Arduino finds extensive use, highlighting its flexibility and potential for innovation.
1. Education and Learning:
Arduino has revolutionized the way electronics and programming are taught in educational institutions. Its user-friendly interface, vast community support, and extensive learning resources make it an ideal platform for students and beginners. Arduino’s simplicity allows learners to grasp fundamental concepts of electronics and coding while encouraging hands-on experimentation and creativity. With Arduino, educators can introduce students to robotics, automation, sensor integration, and Internet of Things (IoT) concepts, fostering a deeper understanding of technology.
2. Hobbyist and Maker Community:
Arduino has become the go-to platform for hobbyists, makers, and do-it-yourself (DIY) enthusiasts worldwide. Its affordability, accessibility, and abundant online resources make it an excellent choice for turning ideas into tangible prototypes. Whether it’s building robots, home automation systems, interactive art installations, or custom electronics projects, Arduino provides a solid foundation for bringing creative visions to life. The thriving Arduino community further facilitates knowledge sharing, collaboration, and inspiration among like-minded individuals.
3. Internet of Things (IoT):
Arduino’s versatility extends to the realm of IoT, where it plays a pivotal role in connecting physical devices to the digital world. By combining Arduino with wireless communication modules like Wi-Fi or Bluetooth, developers can create IoT devices that gather data from sensors, communicate with cloud platforms, and interact with the internet. Arduino’s compatibility with various IoT protocols and its integration with popular IoT platforms make it an essential tool for building smart homes, environmental monitoring systems, wearable devices, and industrial IoT applications.
4. Automation and Robotics:
Arduino empowers the automation and robotics industry by providing a cost-effective and flexible platform for control systems. With its wide range of input and output options, Arduino boards can interface with sensors, actuators, motors, and other electronic components, enabling the creation of autonomous machines. From simple line-following robots to complex robotic arms, Arduino’s programmability and expandability make it an attractive choice for prototyping and developing robotic systems.
5. Prototyping and Product Development:
Arduino’s rapid prototyping capabilities make it a valuable asset in product development cycles. Its ease of use and quick iteration process allow designers and engineers to rapidly test and validate concepts. Arduino boards serve as a starting point for proof-of-concept prototypes, enabling the evaluation of product functionality, user interaction, and feasibility. As the project progresses, Arduino can be utilized as a prototyping tool to develop custom circuit boards or integrate with other embedded systems.
6. Arts and Interactive Installations:
Arduino has become a staple in the world of interactive art and installations. Its ability to sense and respond to the environment, control lighting and sound, and interact with users in real-time makes it an ideal platform for creating immersive experiences. Artists and designers leverage Arduino to build interactive sculptures, kinetic installations, interactive exhibits, and audiovisual performances, blurring the boundaries between art and technology.
7. Industrial and Commercial Applications:
Arduino’s versatility extends to industrial and commercial applications, where its cost-effectiveness and adaptability make it an attractive choice for various projects. Arduino-based solutions can be found in automation, monitoring systems, data acquisition, agricultural technology, environmental sensing, and more. Arduino’s ability to interface with different sensors, actuators, and communication protocols allows for customized solutions tailored to specific industrial needs.
Arduino’s versatility as a platform stems from its open-source nature, extensive community support, and a vast ecosystem of compatible hardware and software. It continues to evolve, with new boards, sensors, and libraries being introduced regularly. Whether you’re a student, hobby
ist, entrepreneur, or industry professional, Arduino offers a playground for innovation and empowers you to turn your ideas into reality.
Conclusion
Arduino code forms the backbone of every Arduino project, enabling you to bring your ideas to life and create interactive systems with ease. By understanding the Arduino coding environment, mastering code structure, exploring programming techniques, leveraging libraries, and embracing Arduino’s versatility, you’ll embark on a transformative journey of innovation and discovery. With the knowledge gained from this article, you’ll be equipped to tackle complex projects, push boundaries, and contribute to the ever-growing Arduino community. So, grab your Arduino board, dive into the world of code, and unlock the full potential of this remarkable platform.
Add comment