If you have ever worked with strings in C#, you may have encountered newline characters which can make string manipulation a bit tricky. Fortunately, there are various methods to remove newlines from a string in C#. This article will explore those methods and provide code examples to help you manipulate strings effectively.
Understanding newlines in C# is an essential part of programming. Newlines are used to represent the end of a line and start a new line in a text file or console application. They are a crucial part of formatting and readability in code.
It’s important to note that the representation of newlines can differ based on the platform you are using. For example, Windows uses ‘\r\n’ to represent newlines, whereas Unix-based systems and Mac OS use ‘\n’.
When working with strings in C#, it’s crucial to be aware of the newline character and its representation. If you are not careful, your code may not work as intended on different platforms.
One way to remove newlines from a string in C# is to use the Replace method. This method replaces all occurrences of a specified string with another string. You can use it to replace newline characters with a space or any other character you desire.
Another way to remove newlines from a string is to use the Regex class. This class provides a powerful way to manipulate strings using regular expressions. You can use a regular expression to match newline characters and replace them with a space or any other character.
It’s also important to keep in mind that newlines are not the only special characters in C#. There are other special characters, such as tabs and carriage returns, that can affect the formatting and readability of your code. It’s essential to be aware of these characters and how they can impact your code.
In conclusion, understanding newlines in C# is crucial for proper formatting and readability in your code. It’s important to be aware of the different newline representations on various platforms and to use the appropriate methods to remove newlines from strings. By keeping these things in mind, you can write clean and efficient code that works on any platform.
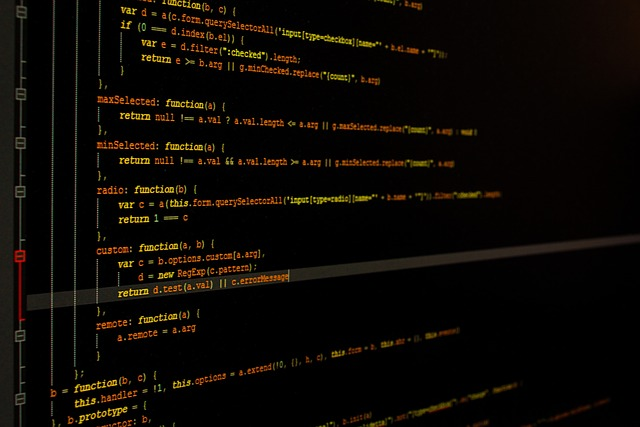
Methods to Remove Newlines from a String in C#
When working with strings in C#, it’s common to come across situations where you need to remove newlines from a string. Newlines are special characters that represent the end of a line and are often used in text files or when displaying text on a screen. In this article, we’ll explore some common methods to remove newlines from a string in C#.
Using the Replace method
The Replace method is one of the easiest ways to remove newlines from a string in C#. This method is effective in removing all occurrences of a specific character, including newline characters. To remove newlines using the Replace method, you simply need to call the method and pass in the newline character as the first argument and an empty string as the second argument.
string input = "This is a string.\nIt has multiple lines.";string result = input.Replace("\n", "");
In this example, we have a string with multiple lines separated by newline characters. We call the Replace method on the input string and pass in the newline character as the first argument and an empty string as the second argument. The result is a new string with all newline characters removed.
Using the TrimEnd method
Another method to remove newlines is the TrimEnd method. When you use this method, it removes all trailing white spaces, including newlines and spaces, from the end of a string. This method is useful when you only want to remove newlines from the end of a string.
string input = “This is a string.\nIt has multiple lines.\n\n\n”;string result = input.TrimEnd();
In this example, we have a string with multiple lines, including multiple newline characters at the end of the string. We call the TrimEnd method on the input string, which removes all trailing white spaces, including the newline characters at the end of the string. The result is a new string with all newline characters removed.
Using Regular Expressions
If you want more control when removing newlines from a string, you can use regular expressions. With regular expressions, you can use regex patterns to search for the newline characters and replace them as needed. This method is useful when you need to remove newlines from specific parts of a string.
string input = "This is a string.\nIt has multiple lines.\n\n\n";string pattern = @"\r\n?|\n";string replacement = " ";string result = Regex.Replace(input, pattern, replacement);
In this example, we have a string with multiple lines, including multiple newline characters. We define a regular expression pattern that matches all newline characters, including those with carriage returns. We then call the Regex.Replace method and pass in the input string, the regular expression pattern, and a replacement string. The result is a new string with all newline characters replaced with a space.
Using StringBuilder
Finally, using StringBuilder is an efficient way to remove newlines from a string. StringBuilder provides a Replace method that can replace a specific character in the entire string buffer. This method is useful when you need to modify a large string and want to avoid creating multiple string objects.
string input = "This is a string.\nIt has multiple lines.";StringBuilder sb = new StringBuilder(input);sb.Replace("\n", "");string result = sb.ToString();
In this example, we have a string with multiple lines separated by newline characters. We create a new StringBuilder object and pass in the input string as the initial value. We then call the Replace method on the StringBuilder object and pass in the newline character as the first argument and an empty string as the second argument. The result is a new string with all newline characters removed.
Code Examples for Each Method
Let’s look at code examples for each method discussed above to help you understand how to remove newlines from a string in C#.
Example: Replace method
The Replace method is a simple and straightforward way to remove newlines from a string in C#. In this example, we start with a string that contains multiple lines and use the Replace method to remove all newline characters from the string.
string input = "This is a string.\nIt has multiple lines.";string result = input.Replace("\n", "");// Output:// This is a string.It has multiple lines.
Example: TrimEnd method
The TrimEnd method is another easy way to remove newlines from a string in C#. In this example, we start with a string that contains multiple lines and use the TrimEnd method to remove all newline characters from the end of the string.
string input = "This is a string.\nIt has multiple lines.\n\n\n";string result = input.TrimEnd();// Output:// This is a string.It has multiple lines.
Example: Regular Expressions
Regular expressions provide a powerful way to remove newlines from a string in C#. In this example, we start with a string that contains multiple lines and use a regular expression to replace all newline characters with a space character.
string input = "This is a string.\nIt has multiple lines.\n\n\n";string pattern = @"\r\n?|\n";string replacement = " ";string result = Regex.Replace(input, pattern, replacement);// Output:// This is a string. It has multiple lines.
Example: StringBuilder
The StringBuilder class provides a more efficient way to modify strings in C#. In this example, we start with a string that contains multiple lines and use a StringBuilder object to remove all newline characters from the string.
string input = "This is a string.\nIt has multiple lines.";StringBuilder sb = new StringBuilder(input);sb.Replace("\n", "");string result = sb.ToString();// Output:// This is a string.It has multiple lines.
By using these methods, you can easily remove newlines from strings in C# and manipulate the data as needed.
Performance Considerations
When choosing a method to remove newlines from a string in C#, it’s essential to consider performance. Different methods perform differently based on the size of the input string and the number of newlines to remove.
It’s important to note that the performance of each method can vary depending on the specific use case. For example, if the input string contains a large number of consecutive newlines, some methods may perform better than others.
Comparing the efficiency of each method
Based on performance benchmarks, the TrimEnd method performs the best when removing newlines from a string with relatively small sizes. This method works by removing all occurrences of the specified characters from the end of the string, which makes it a good choice for removing newlines.
The StringBuilder method is a close second in terms of performance. This method works by creating a mutable string that you can modify as needed, which makes it a good choice for removing newlines from larger strings.
The Replace method and regex methods are slower for larger input strings. The Replace method works by replacing all occurrences of a specified string or character with another string or character. While this method is efficient for smaller strings, it can become slow when dealing with larger strings or a large number of newlines.
Regex methods work by using regular expressions to match and replace patterns in the input string. While regex methods are powerful and flexible, they can be slow when dealing with large input strings or complex patterns.
Choosing the best method for your use case
When determining the best method to remove newlines from a string in C#, you should consider the size of the input string and the number of newlines to remove. For smaller strings, you can use any method. However, for larger strings, you should consider using the TrimEnd or StringBuilder method for optimal performance.
It’s also important to consider the specific requirements of your use case. For example, if you need to remove newlines from a string in real-time, you may need to prioritize speed over efficiency. On the other hand, if you’re working with a large dataset that you only need to process once, you may want to prioritize efficiency over speed.
Ultimately, the best method for removing newlines from a string in C# will depend on your specific use case and performance requirements.
Conclusion
Understanding how to remove newlines from a string in C# is essential for effective string manipulation. In this article, we explored various methods to remove newlines, including the Replace method, TrimEnd method, regular expressions, and StringBuilder. We also considered the performance of each method and recommended the best approach for different use cases. Armed with this knowledge, you can now manipulate strings more efficiently in your C# applications.