JSON (JavaScript Object Notation) is a popular data interchange format widely used for transmitting and storing structured information. It has become a go-to format for many applications due to its simplicity, readability, and interoperability across different programming languages. When working with Go (Golang), a statically-typed and efficient programming language, efficiently reading JSON files is a crucial skill to have. In this article, we will explore the techniques and best practices for reading JSON files in Golang, enabling you to handle complex data structures with ease.
Understanding JSON Files
Before diving into the code, let’s take a moment to understand the structure of JSON files. JSON files consist of key-value pairs, where the keys are strings and the values can be of various types, including strings, numbers, booleans, arrays, and nested objects. These files provide a human-readable and lightweight way to represent data.
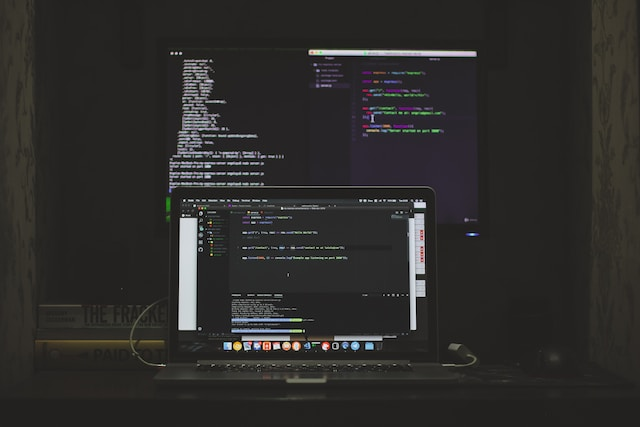
Reading JSON Files in Golang
To read a JSON file in Golang, we’ll leverage the built-in encoding/json package. This package provides functionalities to encode and decode JSON data. To start, we need to open the JSON file and read its contents. Here’s an example code snippet:
package main
import (
"encoding/json"
"fmt"
"io/ioutil"
"log"
)
func main() {
filePath := "data.json"
// Read the JSON file
data, err := ioutil.ReadFile(filePath)
if err != nil {
log.Fatal(err)
}
// Declare a struct to hold the JSON data
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
Email string `json:"email"`
}
// Unmarshal the JSON data into the struct
var person Person
err = json.Unmarshal(data, &person)
if err != nil {
log.Fatal(err)
}
// Access the data
fmt.Println("Name:", person.Name)
fmt.Println("Age:", person.Age)
fmt.Println("Email:", person.Email)
}
In this example, we define a Person struct that corresponds to the structure of the JSON data. We then use the json.Unmarshal function to map the JSON data to the struct. Finally, we can access the individual fields of the struct.
Handling Complex JSON Structures
JSON files often contain complex nested structures, such as arrays and nested objects. To handle such structures, we can leverage the power of Go’s data types. For instance, if the JSON file contains an array of objects, we can declare a slice of structs to unmarshal the data into. By appropriately defining the struct fields, we can access the data in a structured manner.
Dealing with Unknown JSON Structures
Sometimes, JSON files may have dynamic structures, making it difficult to define corresponding Go structs in advance. In such cases, we can use the json.RawMessage type to unmarshal the JSON data into a raw message, which can then be parsed further based on the specific requirements.
Best Practices and Additional Considerations
When working with JSON files in Golang, it’s essential to handle errors properly. Always check for potential errors during file reading, JSON unmarshaling, and data access. Additionally, consider using third-party libraries like jsoniter for improved performance when dealing with large JSON files.
Conclusion:
In this comprehensive guide, we explored the process of reading JSON files in Golang. We learned about the structure of JSON files, examined code examples for reading and parsing JSON data, and discussed techniques for handling complex and unknown JSON structures. By mastering these techniques and following best practices, you can confidently handle JSON files in your Golang applications, enabling seamless data integration and manipulation. Happy coding!
Add comment