Welcome to the world of C#, where elegance meets efficiency in the form of the conditional operator. In C#, this powerful tool provides a shorthand if/else alternative, allowing you to make decisions and control program flow with concise and expressive code. By mastering the conditional operator, you can streamline your development process and write cleaner, more readable code.
Unleashing the Power of the Conditional Operator
In this section, we will delve deeper into the capabilities of the conditional operator in C# and understand how it can enhance your coding experience. The conditional operator offers several advantages over traditional if-else statements, such as its compact syntax and ability to be used within expressions.
One of the primary benefits of the conditional operator is its conciseness. By using a single line of code, you can perform conditional operations and assign values based on the outcome. This brevity not only reduces clutter in your code but also makes it more readable and easier to maintain.
Another advantage of the conditional operator is its versatility. It can be used within expressions, allowing you to assign the result directly to a variable or use it as part of a larger expression. This flexibility enables you to incorporate conditional logic seamlessly into your code without breaking the flow.
Furthermore, the conditional operator promotes efficiency in your coding process. With its concise syntax, you can quickly express conditional checks and assign values, resulting in faster development and improved productivity. This efficiency becomes particularly beneficial when dealing with repetitive or straightforward decision-making scenarios.
It is important to note that while the conditional operator offers brevity and efficiency, it should be used judiciously. Overusing the conditional operator or nesting it excessively can lead to complex and hard-to-read code. It is essential to strike a balance between leveraging its power and maintaining code readability and maintainability.
By mastering the conditional operator, you can unlock a world of possibilities in your C# programming endeavors. Its elegance and expressiveness make it a valuable tool for making decisions, assigning values, and controlling program flow. Now, let’s dive into practical examples to see the conditional operator in action.
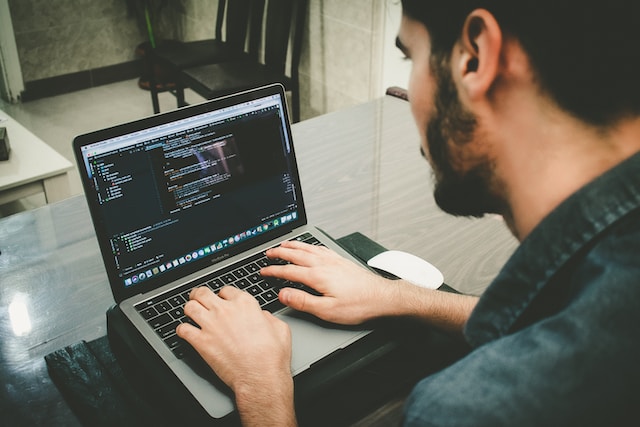
Choosing Between Two Options
The conditional operator shines when it comes to choosing between two options based on a condition. It provides a concise and intuitive way to express such decisions in your code. Let’s explore some practical examples to understand how the conditional operator works in these scenarios.
Example 1: Checking a Number’s Sign
Suppose you have a variable `number` that represents an integer. You want to determine whether the number is positive or negative. Here’s how you can accomplish this using the conditional operator:
```csharp
int number = -5;
string sign = (number >= 0) ? "positive" : "negative";
Console.WriteLine($"The number is {sign}.");
```
In this example, we use the conditional operator to check if `number` is greater than or equal to zero. If the condition is true, the value “positive” is assigned to the `sign` variable; otherwise, the value “negative” is assigned. The result is then displayed on the console.
Example 2: Determining Pass or Fail
Imagine you have a variable `score` representing a student’s exam score. You want to determine whether the student has passed or failed based on a passing threshold. Let’s see how the conditional operator can help:
```csharp
int score = 85;
string result = (score >= 70) ? "pass" : "fail";
Console.WriteLine($"The student's result is {result}.");
```
In this case, we use the conditional operator to check if `score` is greater than or equal to 70, the passing threshold. If the condition is true, the value “pass” is assigned to the `result` variable; otherwise, the value “fail” is assigned. The outcome is then printed on the console.
The conditional operator allows you to condense these decision-making processes into a single line of code. It promotes code readability by eliminating the need for multiple if-else statements and provides a clear and concise representation of your intentions.
Remember, you can use the conditional operator with any expressions that evaluate to a boolean value. This flexibility opens up numerous possibilities for making decisions and choosing between different options in your code.
In the next section, we will explore how the conditional operator can handle multiple conditions, allowing for more complex decision-making scenarios.
Checking Multiple Conditions
The conditional operator in C# can handle multiple conditions, providing a compact and expressive way to evaluate complex decision-making scenarios. By nesting conditional operators within parentheses, you can chain together multiple conditions and achieve the desired outcome. Let’s dive into some examples to illustrate the power of the conditional operator with multiple conditions.
Categorizing a Number
Suppose you have a variable `number` that represents an integer. You want to categorize the number into three groups: positive, negative, or zero. Here’s how you can accomplish this using the conditional operator:
```csharp
int number = 10;
string category = (number > 0) ? "positive" : (number < 0) ? "negative" : "zero";
Console.WriteLine($"The number is {category}.");
```
In this example, we nest two conditional operators to check the value of `number`. The first condition checks if `number` is greater than 0, assigning the value “positive” if true. If the first condition is false, the second condition checks if `number` is less than 0, assigning the value “negative” if true. Finally, if both conditions are false, the value “zero” is assigned. The resulting category is then displayed on the console.
Determining Letter Grade
Let’s say you have a variable `score` representing a student’s exam score. You want to determine the corresponding letter grade based on score ranges. Here’s how you can utilize the conditional operator for this task:
```csharp
int score = 85;
string grade = (score >= 90) ? "A" : (score >= 80) ? "B" : (score >= 70) ? "C" : "F";
Console.WriteLine($"The student's grade is {grade}.");
```
In this example, we employ three nested conditional operators to evaluate `score` against different score ranges. Starting from the highest range, if `score` is greater than or equal to 90, it receives the grade “A”. Otherwise, if the first condition is false and `score` is greater than or equal to 80, it receives the grade “B”. Similarly, the process continues for “C” and, ultimately, “F” if none of the conditions are met. The determined grade is then printed to the console.
By nesting conditional operators, you can create a hierarchical decision-making structure, enabling you to handle multiple conditions in a concise and readable manner. However, it’s essential to strike a balance between readability and complexity. Excessive nesting can make the code hard to understand, so use your judgment and consider refactoring if the conditions become too convoluted.
In the next section, we will summarize the key points covered in this article and provide related C# tutorials to further expand your knowledge.
Summary
In this article, we have explored the power and versatility of the conditional operator in C#. We began by understanding its role as a shorthand if/else alternative, offering concise and expressive decision-making capabilities. The conditional operator proves beneficial in scenarios where you need to choose between two options or evaluate multiple conditions.
We discussed how the conditional operator allows you to make decisions based on a single condition, assigning different values accordingly. This streamlined approach eliminates the need for lengthy if-else statements and enhances code readability. Whether you’re categorizing data, determining pass/fail outcomes, or handling other binary decisions, the conditional operator offers an elegant solution.
Furthermore, we examined how the conditional operator can handle multiple conditions by nesting them within parentheses. This nesting allows you to create complex decision-making structures without sacrificing readability. By chaining together conditional operators, you can categorize data into multiple groups, assign letter grades, or perform other intricate evaluations.
Throughout this article, we emphasized the importance of maintaining code readability. While the conditional operator provides concise and expressive code, it’s essential to strike a balance and avoid excessive nesting or overly complex conditions. Clarity and maintainability should always be prioritized, even when leveraging the power of the conditional operator.
To continue expanding your knowledge of C# and decision-making techniques, consider exploring the following related tutorials:
- Mastering Decision Making in C# with If-Else Statements: Understand the traditional if-else statements and learn best practices for making decisions in your C# code;
- Simplify Your Code with Switch Statements in C#: Explore the switch statement as an alternative decision-making approach and discover its advantages in specific scenarios;
- Understanding Comparison Operators in C#: Dive deeper into the world of comparison operators and learn how they can be utilized alongside the conditional operator for more sophisticated evaluations.
By combining your understanding of these decision-making techniques, you can become a proficient C# developer, capable of writing elegant and efficient code.
Related C# Tutorials:
– Mastering Decision Making in C# with If-Else Statements
– Simplify Your Code with Switch Statements in C#
– Understanding Comparison Operators in C#
Conclusion:
The conditional operator in C# provides a concise and powerful tool for decision-making and value assignment. By leveraging its capabilities, you can streamline your code, enhance readability, and improve coding efficiency. Remember to use the conditional operator judiciously, ensuring your code remains maintainable and easy to understand.
As you continue your journey in C# development, practice incorporating the conditional operator into your projects. Explore different scenarios, experiment with nested conditions, and seek opportunities to optimize your code. With time and experience, you will become adept at harnessing the full potential of the conditional operator and elevating your coding skills in C#. The conditional operator in C# is a valuable addition to your coding arsenal. By harnessing its power, you can write more expressive and efficient code. Remember to use it judiciously, ensuring that your code remains readable and maintainable. With practice, you will become adept at leveraging the conditional operator to streamline your C# projects and unlock new possibilities in your coding journey.
Add comment