C# offers a powerful tool for iterating through collections and arrays, known as the foreach loop. While it simplifies the process of accessing each element in a sequence, there are situations where you may need to track the index of the current item during iteration. In this article, we will explore different techniques to incorporate an index variable into the foreach loop, allowing you to enhance your code’s functionality and efficiency.
Option 1 – Create and Manage an Integer Loop Variable
In certain scenarios, you may prefer to have explicit control over the index variable in the foreach loop. This option allows you to create and manage an integer counter that keeps track of the current index during iteration. Let’s dive into the details of how you can implement this approach effectively.
To begin, declare an integer variable before the foreach loop, which will serve as your index counter. You can initialize it to zero or any other desired starting value based on your requirements. For example:
```csharp
int index = 0;
```
Next, within the foreach loop, you can increment the index variable by one with each iteration. This can be achieved using the increment operator (`++`). Here’s an example that demonstrates this approach:
```csharp
string[] fruits = { "apple", "banana", "orange" };
foreach (string fruit in fruits)
{
Console.WriteLine($"Fruit: {fruit}, Index: {index}");
index++;
}
```
In the above code snippet, we have an array of fruits, and we want to print each fruit along with its corresponding index. By incrementing the index variable manually, we ensure that it accurately represents the current index during each iteration.
It’s important to note that this approach provides you with full control over the index variable. You can modify it as per your specific requirements within the foreach loop. This flexibility can be advantageous in situations where you need to perform additional operations based on the index, such as conditional branching or complex calculations.
However, it’s crucial to handle edge cases carefully. Ensure that you don’t inadvertently modify the index variable outside the loop or encounter index out-of-bounds errors. Pay attention to the size of your collection or array to avoid accessing elements beyond their valid indices.
By utilizing this option, you gain fine-grained control over the index variable in the foreach loop. This approach is particularly useful when you need explicit management of the index and additional control flow logic during iteration. However, keep in mind that it requires careful handling to prevent potential errors and edge cases.
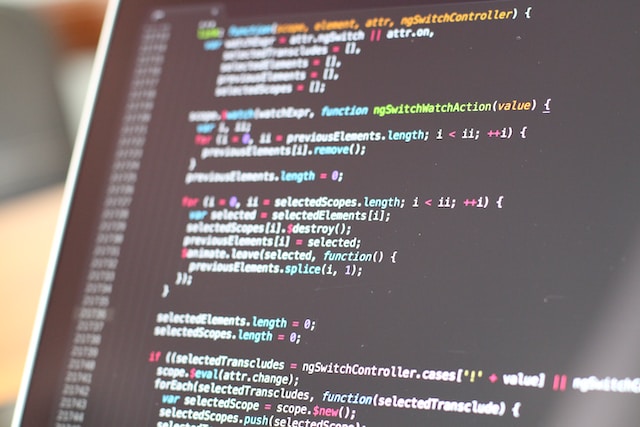
Option 2 – Use a Tuple to Retrieve the Value and Index
Another approach to incorporating an index variable in the foreach loop is by leveraging tuples. C# provides a convenient way to retrieve both the value and index using a tuple within the foreach loop. This approach simplifies your code and offers an elegant solution for accessing the index while iterating.
To implement this option, you need to modify the foreach loop statement slightly. Instead of declaring a single variable to hold the loop value, you can define a tuple that captures both the value and the index. Here’s an example:
```csharp
string[] colors = { "red", "green", "blue" };
foreach ((string color, int index) in colors.Select((value, index) => (value, index)))
{
Console.WriteLine($"Color: {color}, Index: {index}");
}
```
In the above code snippet, we use the `Select()` method from LINQ to create a tuple that contains both the value and index. The `value` represents the current element in the iteration, while the `index` corresponds to its index in the collection.
By deconstructing the tuple within the foreach loop declaration, we can easily access the value using the `color` variable and the index using the `index` variable. This allows us to directly use these variables within the loop body, eliminating the need for an additional index counter.
Using tuples in this manner provides a clean and concise way to retrieve the index while iterating through a collection. It enhances the readability of your code by clearly expressing your intention to include the index in the loop logic.
Moreover, this approach is particularly useful when you need both the value and index for performing operations or making decisions within the loop. It simplifies your code by avoiding the need for manual index management and separate counter variables.
However, it’s important to note that this approach requires C# 7.0 or later versions to support tuple deconstruction in foreach loops. Ensure that your development environment and target platforms are compatible before using this technique.
By leveraging tuples to retrieve the value and index within the foreach loop, you can streamline your code and make it more expressive. This approach provides a concise and elegant solution, eliminating the need for separate index counters and manual management.
Option 3 – Replace foreach with the for Statement
In certain situations, replacing the foreach loop with a traditional for statement can be a viable option to access both the value and index within the loop effortlessly. By making this switch, you can retain full control over the index variable and take advantage of the flexibility offered by the for loop structure.
To implement this option, we need to modify the foreach loop statement and replace it with a for loop that iterates over the indices of the collection or array. Here’s an example:
```csharp
string[] animals = { "dog", "cat", "elephant" };
for (int index = 0; index < animals.Length; index++)
{
string animal = animals[index];
Console.WriteLine($"Animal: {animal}, Index: {index}");
}
```
In the above code snippet, we declare an integer `index` variable and initialize it to zero. The for loop condition checks whether the index is less than the length of the collection or array. By incrementing the index at the end of each iteration, we can iterate through each element of the collection.
Within the loop body, we can access both the value and the index. In this case, we assign the current element to the `animal` variable and output both the animal and its corresponding index using `Console.WriteLine()`.
Replacing the foreach loop with a for loop allows you to have complete control over the index variable, giving you the flexibility to manipulate it as needed. This approach is advantageous when you require more complex index calculations or when you want to perform operations based on specific index ranges.
Additionally, the for loop approach can be more performant in certain scenarios. Since it directly operates on the indices, it eliminates the need for additional lookups, resulting in potential performance improvements, especially for large collections.
However, it’s important to note that by using the for loop, you lose some of the conveniences provided by the foreach loop, such as automatic enumeration and protection against modification of the collection during iteration. Ensure that you handle edge cases carefully and avoid modifying the collection while iterating.
By replacing the foreach loop with a for statement, you can retain full control over the index variable and harness the flexibility of the for loop structure. This approach is suitable for scenarios that require more advanced index manipulation or when performance optimization is crucial.
Comparison Table
To aid in your decision-making process, let’s present a comparison table that highlights the strengths and weaknesses of each option discussed for incorporating an index variable in the foreach loop. This table will help you evaluate and choose the approach that best aligns with your specific requirements and coding style.
Option | Description | Advantages | Disadvantages |
---|---|---|---|
Option 1: Integer Loop | Create and manage an integer loop variable manually. | – Provides explicit control over the index variable. – Enables additional operations based on the index. | – Requires manual management of the index variable. – Potential for index out-of-bounds errors. |
Option 2: Tuple | Use a tuple to retrieve both the value and index within the foreach loop. | – Simplifies code and eliminates the need for a separate index variable. – Enables easy access to both value and index. | – Requires C# 7.0 or later versions. – Limited to value-type tuples. |
Option 3: for Loop | Replace the foreach loop with a for statement that iterates over the indices. | – Provides full control over the index variable. – Enables more complex index calculations. – Potential for performance improvements. | – Requires manual iteration and index management. – Eliminates the conveniences of the foreach loop. |
By examining the comparison table, you can evaluate the trade-offs associated with each option. Consider your specific use case, the level of control required over the index, the need for additional operations based on the index, and the performance considerations.
For example, if you require explicit control over the index and need to perform complex operations based on the index, Option 1: Integer Loop might be the most suitable. However, be cautious about index out-of-bounds errors and ensure proper bounds checking.
If you prefer a cleaner and more concise approach, Option 2: Tuple can simplify your code by providing easy access to both the value and the index. Just make sure your development environment supports the required C# version.
On the other hand, if you seek fine-grained control over the index and want to optimize performance, Option 3: for Loop can be a suitable choice. However, be mindful of the increased responsibility for managing the loop and potential loss of convenience.
Consider these factors and choose the option that best fits your specific requirements, coding style, and the trade-offs you are willing to make. The goal is to strike a balance between code readability, maintainability, performance, and control.
Real-World Examples and Code Snippets
To solidify your understanding of incorporating an index variable in the foreach loop, let’s explore some real-world examples and provide code snippets that demonstrate the practical implementation of each technique discussed.
Example 1: Option 1 – Create and Manage an Integer Loop Variable
Suppose you have a list of names, and you want to display each name along with its corresponding index. Here’s how you can achieve this using Option 1:
```csharp
List<string> names = new List<string> { "John", "Mary", "David" };
int index = 0;
foreach (string name in names)
{
Console.WriteLine($"Name: {name}, Index: {index}");
index++;
}
```
In this example, we declare an integer `index` variable and increment it manually within the foreach loop. This allows us to display each name with its corresponding index accurately.
Example 2: Option 2 – Use a Tuple to Retrieve the Value and Index
Let’s consider a scenario where you have an array of temperatures, and you want to find the maximum temperature along with its index. Here’s an example of how Option 2 can simplify this task:
```csharp
double[] temperatures = { 25.6, 30.2, 28.8, 27.4 };
int maxIndex = -1;
double maxValue = double.MinValue;
foreach ((double temperature, int index) in temperatures.Select((value, index) => (value, index)))
{
if (temperature > maxValue)
{
maxValue = temperature;
maxIndex = index;
}
}
Console.WriteLine($"Max Temperature: {maxValue}, Index: {maxIndex}");
```
In this code snippet, we utilize a tuple to retrieve both the temperature value and its index within the foreach loop. By comparing each temperature with the current maximum value, we update the `maxValue` and `maxIndex` variables accordingly.
Example 3: Option 3 – Replace foreach with the for Statement
Let’s consider a practical scenario where you have a string and want to capitalize every character at an even index. Here’s an example of using Option 3 to accomplish this:
```csharp
string text = "hello world";
char[] characters = text.ToCharArray();
for (int index = 0; index < characters.Length; index++)
{
if (index % 2 == 0)
{
characters[index] = char.ToUpper(characters[index]);
}
}
string result = new string(characters);
Console.WriteLine(result); // Output: HeLlO wOrLd
```
In this code snippet, we replace the foreach loop with a for loop to iterate through the indices of the characters array. By checking if the index is even using the modulo operator `%`, we capitalize the character at even indices, resulting in the desired output.
By examining these real-world examples and code snippets, you can gain practical insights into implementing each technique discussed. Experiment with different scenarios, modify the code to suit your specific requirements, and observe the behavior to deepen your understanding of utilizing an index variable in the foreach loop.
Best Practices and Recommendations
In this section, we will share some best practices and recommendations when it comes to utilizing index variables in the foreach loop. You will learn about error handling, performance considerations, and how to choose the most appropriate technique based on your specific use cases.
Conclusion:
In conclusion, incorporating an index variable into the foreach loop can greatly enhance the power and flexibility of your C# code. We have explored three distinct approaches: creating and managing an integer loop variable, using a tuple to retrieve the value and index, and replacing foreach with the for statement. By leveraging these techniques in the appropriate context, you can optimize your iteration process and achieve more efficient code. Experiment with these methods, weigh their pros and cons, and embrace the one that best suits your coding style and project requirements. With these newfound skills, you will become a proficient C# developer capable of tackling complex iterative tasks with ease.
Add comment