Rounding numbers is a fundamental operation in programming that allows us to simplify and manipulate numerical values. In Python, there are several methods available to round numbers to the nearest whole integer. Whether you need to round up or down, Python offers versatile built-in functions to help you achieve precise results.
In this comprehensive guide, we will delve into the various techniques and functions for rounding to whole numbers in Python. We’ll explore the popular round() function, along with the math.floor() and math.ceil() functions, which provide more specific rounding behaviors. Additionally, we’ll cover rounding values in lists and arrays, demonstrating both list comprehensions and for loops for efficient processing.
By the end of this article, you’ll be equipped with the knowledge and practical examples necessary to confidently round numerical values in Python. Let’s embark on this rounding journey and unravel the secrets of precision!
Round Values Up and Down: Python’s round() Function
Python’s built-in round() function is a versatile tool for rounding numerical values to the nearest whole integer. It follows the standard rounding rules, where values ending in .5 are rounded to the nearest even number.
The round() function takes two parameters: the number to be rounded and an optional parameter specifying the number of decimal places to round to. If the second parameter is omitted, the function rounds to the nearest whole number.
It’s important to note that the behavior of round() can sometimes be surprising due to the rounding rules it follows. For instance, rounding 2.5 using round() will result in 2, while rounding 3.5 will give 4. This is because, in cases of ties, round() rounds to the nearest even number.
To overcome this behavior and achieve more predictable rounding results, other functions like math.floor() and math.ceil() can be used, which we’ll explore in subsequent sections.
Example Code:
```python
value = 7.9
rounded_value = round(value)
print(rounded_value) # Output: 8
value2 = 4.5
rounded_value2 = round(value2)
print(rounded_value2) # Output: 4
```
In the first example, the value 7.9 is rounded to the nearest whole number, resulting in 8. However, in the second example, the value 4.5 is rounded down to 4 due to the tie-breaking rule of rounding to the nearest even number.
By understanding the behavior and limitations of the round() function, you can effectively round numerical values in Python while considering the specific requirements of your application.
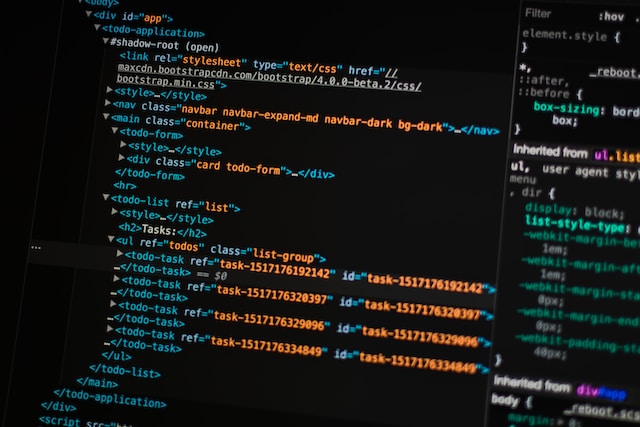
Round Down to the Next Integer: Python’s math.floor() Function
In situations where you need to round values down to the nearest whole number, Python provides the math.floor() function as a reliable tool. Unlike the round() function, math.floor() always rounds down to the next integer, regardless of the decimal portion of the value.
The math.floor() function is part of the math module, so you need to import it before using it in your code. Once imported, you can apply math.floor() to any numerical value, and it will return the largest integer less than or equal to the given value.
Example Code:
```python
import math
value = 9.6
rounded_value = math.floor(value)
print(rounded_value) # Output: 9
value2 = -3.2
rounded_value2 = math.floor(value2)
print(rounded_value2) # Output: -4
```
In the first example, the value 9.6 is rounded down to 9 because math.floor() always rounds towards negative infinity. Similarly, in the second example, the value -3.2 is rounded down to -4. The math.floor() function provides consistent and predictable rounding behavior, making it suitable for various applications.
One common use case for math.floor() is when you need to truncate decimal values without rounding. For instance, if you’re working with monetary calculations and need to discard the fractional part of a value, math.floor() ensures that you always round down, maintaining the integrity of your calculations.
By utilizing math.floor() appropriately, you can effectively handle scenarios that require rounding down to the next whole integer in your Python programs.
Round Up to the Next Integer: Python’s math.ceil() Function
When you need to round values up to the nearest whole number in Python, the math.ceil() function comes to the rescue. This function, available within the math module, always rounds up to the next integer, regardless of the decimal portion of the value.
To use math.ceil(), you first need to import the math module into your code. Once imported, you can apply math.ceil() to any numerical value, and it will return the smallest integer greater than or equal to the given value.
Example Code:
```python
import math
value = 5.1
rounded_value = math.ceil(value)
print(rounded_value) # Output: 6
value2 = -2.8
rounded_value2 = math.ceil(value2)
print(rounded_value2) # Output: -2
```
In the first example, the value 5.1 is rounded up to 6, as math.ceil() always rounds towards positive infinity. Similarly, in the second example, the value -2.8 is rounded up to -2. The math.ceil() function provides consistent and predictable rounding behavior, making it a valuable tool in various scenarios.
One common application of math.ceil() is when you need to allocate resources or quantities that must be whole numbers. By rounding up, you ensure that you have enough resources or quantities to cover the required amount.
It’s worth noting that math.ceil() may not always be suitable for scenarios that involve financial calculations or precision requirements. In such cases, alternative rounding techniques or functions should be considered to meet the specific needs of your application.
By leveraging the power of math.ceil(), you can effectively handle situations that require rounding up to the next whole integer in your Python programs.
Round All Values in a Python List or Array
When working with collections of numerical data, such as lists or arrays, it is common to encounter the need to round all the values simultaneously. Python provides efficient methods to round all values in a list or array, allowing you to process the data in a concise and straightforward manner.
4.1 Rounding with List Comprehension:
List comprehension is a concise and powerful technique in Python that allows you to create new lists based on existing lists’ elements. It provides an elegant solution for rounding all the values in a list at once.
Example Code:
```python
numbers = [3.7, 2.1, 4.9, 6.5]
rounded_numbers = [round(num) for num in numbers]
print(rounded_numbers) # Output: [4, 2, 5, 7]
```
In the example above, the list comprehension iterates over each element in the `numbers` list, applying the `round()` function to round each value to the nearest whole number. The resulting rounded values are collected in a new list called `rounded_numbers`. This approach is concise, efficient, and allows you to perform rounding operations on large lists with ease.
4.2 Rounding with a For Loop:
If you prefer a more traditional approach or need additional control over the rounding process, using a `for` loop is a suitable option. It provides flexibility and allows for custom rounding logic when rounding values in a list or array.
Example Code:
```python
numbers = [3.7, 2.1, 4.9, 6.5]
rounded_numbers = []
for num in numbers:
rounded_numbers.append(round(num))
print(rounded_numbers) # Output: [4, 2, 5, 7]
```
In this example, we create an empty list called `rounded_numbers`. The `for` loop iterates over each element in the `numbers` list, applying the `round()` function to round each value and then appending it to the `rounded_numbers` list. This approach provides more flexibility and allows for custom rounding logic if needed.
By employing either list comprehensions or `for` loops, you can easily round all the values in a Python list or array. These techniques are not limited to rounding to whole numbers; you can adapt them to perform rounding to specific decimal places or customize the rounding behavior according to your requirements.
It’s important to note that the specific rounding technique you choose should align with the rounding rules and precision needed for your dataset. Additionally, consider the potential impacts on subsequent calculations or data analyses when applying rounding operations.
Overall, rounding all values in a Python list or array using list comprehensions or `for` loops provides a convenient and efficient way to process numerical data in bulk while maintaining code readability and flexibility.
Additional Tips and Considerations for Rounding in Python
When working with rounding operations in Python, there are several additional tips and considerations that can help you achieve accurate and reliable results. Let’s explore these tips to enhance your rounding experience:
5.1 Handling Ties:
Python’s built-in round() function follows a tie-breaking rule where values ending in .5 are rounded to the nearest even number. While this behavior is generally suitable for most scenarios, there might be cases where you need different rounding behavior, such as always rounding up in the case of a tie.
To achieve consistent rounding behavior, you can employ other rounding techniques like math.ceil() or math.floor(). Additionally, you can implement custom rounding logic using conditional statements to handle ties according to your specific requirements.
5.2 Rounding with Specific Precision:
In some cases, you may need to round values to a specific number of decimal places rather than rounding to the nearest whole number. Python’s round() function provides the flexibility to specify the number of decimal places for rounding. Simply pass the desired number of decimal places as the second parameter to the round() function.
Example Code:
```python
value = 3.14159
rounded_value = round(value, 2)
print(rounded_value) # Output: 3.14
```
In this example, the value 3.14159 is rounded to 2 decimal places, resulting in 3.14. Specifying the precision allows you to control the level of detail in your rounding operations.
5.3 Rounding Large Numbers or Numbers in Scientific Notation:
When dealing with large numbers or numbers expressed in scientific notation, it’s crucial to consider the scale and magnitude of the values. Rounding such numbers directly may result in unexpected outcomes due to the limitations of floating-point arithmetic.
To round large numbers or numbers in scientific notation accurately, it is recommended to convert them to appropriate data types like Decimal or Fraction from the decimal module. These data types provide precise decimal arithmetic and enable accurate rounding operations even with extremely large or small numbers.
5.4 Considerations for Financial Calculations:
Rounding is often encountered in financial calculations, where precision and accuracy are critical. However, due to the nature of decimal representation in computers, rounding errors can occur, leading to inaccurate results.
To handle financial calculations effectively, it is recommended to use specialized modules like Decimal or fractions instead of standard floating-point arithmetic. These modules offer precise decimal calculations and rounding methods suitable for financial contexts.
Additionally, consult relevant financial regulations or standards to ensure compliance with specific rounding rules applicable to your jurisdiction or industry.
5.5 Document Rounding Decisions:
When rounding values in your code, it is good practice to document and communicate the rounding decisions made. By explicitly mentioning the rounding strategy or precision used, you can enhance the transparency and reproducibility of your code.
Clear documentation helps others understand the rounding process, reduces confusion, and ensures consistency when working with numerical data.
By considering these additional tips and considerations, you can handle rounding operations effectively and achieve accurate results in your Python programs. Adapt the techniques to match the specific requirements of your application and always maintain awareness of potential rounding errors or implications for subsequent calculations.
Additional Applications of Rounding in Python
Rounding in Python extends beyond basic numerical operations. It finds applications in various domains and scenarios. Let’s explore some additional applications where rounding plays a significant role:
7.1 Data Visualization:
Data visualization is a powerful technique for conveying information and insights visually. Rounding can be valuable when working with data that requires discrete representation, such as creating histograms or bar charts. By rounding values before plotting, you can group data points into meaningful categories, enhancing the interpretability of the visualizations.
7.2 Statistical Analysis:
In statistical analysis, rounding can be useful for simplifying calculations and interpreting results. Rounding data to a specific precision can reduce the complexity of computations without significantly affecting the overall analysis. However, it’s crucial to consider the impact of rounding on statistical measures like means, medians, or standard deviations, as it may introduce bias or distort the accuracy of the analysis.
7.3 Algorithm Design:
Rounding plays a role in algorithm design, particularly when dealing with discrete quantities or constraints. For example, in optimization problems, rounding variables or objective function values may be necessary to ensure the feasibility or practicality of solutions. Rounding can also be applied in algorithms that involve numerical approximations or discretization.
7.4 User Interface and Display Formatting:
When presenting numerical values in user interfaces or reports, rounding can improve readability and aesthetic appeal. Displaying excessive decimal places can clutter the interface or make the information harder to comprehend. By rounding values to an appropriate level of precision, you can enhance the user experience and make the displayed data more visually appealing.
7.5 Resource Allocation and Planning:
Rounding plays a vital role in resource allocation and planning tasks. For example, when allocating resources such as inventory, staff, or budgets, rounding up can ensure that you have sufficient resources to meet demand or accommodate uncertainties. Rounding can also be applied in planning scenarios to determine the number of units required for manufacturing or the duration of tasks.
7.6 Game Development and Simulations:
In game development and simulations, rounding is often used to discretize continuous values or to simulate random events. For instance, when simulating a dice roll in a game, rounding can be applied to generate a random integer within a specific range. Rounding is also useful for determining hit points, scores, or any other game mechanics that require whole number representations.
By considering these additional applications of rounding in Python, you can expand your understanding of how rounding operations can be utilized across diverse fields and tasks. Rounding is a versatile tool that can bring precision, simplicity, and practicality to various computational scenarios.
In conclusion, rounding is not only a basic mathematical operation but also a powerful technique with wide-ranging applications. By harnessing the capabilities of rounding in Python, you can manipulate data, enhance visualizations, simplify calculations, and make informed decisions in your projects across different domains.
Best Practices for Rounding in Python
To ensure accurate and reliable results when rounding in Python, it is important to follow certain best practices. Let’s explore some recommended practices to help you achieve precision and consistency in your rounding operations:
8.1 Understand Rounding Methods and Rules:
Before applying rounding in your code, make sure you have a clear understanding of the rounding methods and rules being used. Familiarize yourself with Python’s built-in round() function as well as the math.ceil() and math.floor() functions, their behavior for positive and negative numbers, and their handling of ties. This knowledge will enable you to choose the appropriate rounding technique for each specific scenario.
8.2 Document Rounding Policies and Precision Requirements:
When working on projects that involve rounding, it is essential to document the rounding policies and precision requirements. Clearly define and communicate the rounding rules, tie-breaking strategies, and the desired level of precision. This documentation serves as a reference for yourself and others who may work on the codebase in the future, ensuring consistency in rounding decisions.
8.3 Consider Data Precision and Scale:
Take into account the precision and scale of the data you are rounding. Ensure that the precision used in rounding aligns with the nature of the data. For example, financial calculations often require higher precision to avoid rounding errors, while certain scientific computations may demand specific decimal places. Understanding the characteristics of your data will guide you in selecting the appropriate rounding techniques and precision levels.
8.4 Test and Validate Rounding Results:
Before deploying your code, thoroughly test and validate the rounding results. Create test cases that cover various scenarios and edge cases to ensure that rounding behaves as expected. Compare the rounded values with manual calculations or expected results to verify accuracy. Automated testing frameworks can assist in streamlining the testing process and improving code reliability.
8.5 Consider Performance Implications:
While rounding operations are generally efficient, they can impact performance when dealing with large datasets or complex computations. Consider the performance implications of your rounding approach, especially when working with loops or nested operations. In such cases, optimizing the code or exploring alternative algorithms may be necessary to achieve the desired performance.
8.6 Maintain Consistency in Rounding Methods:
To avoid confusion and inconsistencies, maintain consistency in the rounding methods used throughout your codebase. Stick to a specific rounding approach, whether it’s using the built-in round() function, math.ceil(), math.floor(), or other custom rounding techniques. Mixing different rounding methods within the same codebase can lead to unpredictable results and make the code harder to understand and maintain.
8.7 Consider Decimal or Fraction Modules for Precision:
For scenarios that demand precise decimal arithmetic or fractional calculations, consider utilizing Python’s decimal or fractions modules. These modules offer precise decimal arithmetic, enabling accurate rounding operations even with extremely large or small numbers. By using these modules, you can ensure precision and avoid potential rounding errors inherent in floating-point arithmetic.
By adhering to these best practices, you can enhance the accuracy, reliability, and readability of your rounding operations in Python. Consistent rounding policies, thorough testing, and consideration of precision requirements will help you achieve precise and reliable results in your code.
Rounding is a powerful tool, but it should be applied with care and consideration. By following best practices, you can harness the full potential of rounding in Python and leverage its benefits across a wide range of applications and scenarios.
Common Pitfalls and Troubleshooting Tips
When working with rounding operations in Python, it’s important to be aware of potential pitfalls and challenges that may arise. Understanding these common issues and having troubleshooting strategies in place will help you overcome obstacles and ensure accurate rounding results. Let’s explore some common pitfalls and provide tips for troubleshooting:
9.1 Rounding Errors in Floating-Point Arithmetic:
Python, like many programming languages, uses floating-point arithmetic to represent and manipulate decimal numbers. However, floating-point arithmetic can introduce small rounding errors due to the limited precision of binary representations. These errors can accumulate and affect rounding results, especially in complex calculations or iterative processes.
To mitigate rounding errors, consider using the decimal module, which provides precise decimal arithmetic. The decimal module allows you to control the precision and rounding methods, ensuring accurate calculations even with decimal fractions.
9.2 Unexpected Results with Negative Numbers:
When rounding negative numbers, be mindful of the rounding behavior and its impact on the sign. Python’s built-in round() function follows the tie-breaking rule of rounding to the nearest even number for values ending in .5. This can lead to unexpected results when rounding negative numbers, as the rounding direction may differ from what you anticipate.
To achieve consistent rounding behavior for negative numbers, consider using math.floor() or math.ceil() based on your desired rounding direction. This will ensure that negative numbers are rounded in the expected manner.
9.3 Inconsistent Rounding Behavior with tiebreaker=’round_half_up’:
Python’s round() function accepts an optional argument, tiebreaker, which allows you to specify the rounding behavior for values ending in .5. The default tiebreaker=’round_half_even’ rounds to the nearest even number. However, if you explicitly set tiebreaker=’round_half_up’ to always round up in case of a tie, be aware that this behavior may not be consistent across different Python versions or implementations.
To ensure consistent rounding behavior with tiebreaker=’round_half_up’, consider using math.ceil() instead. This will guarantee that values ending in .5 are always rounded up, regardless of the Python version or implementation.
9.4 Unexpected Results with Large Numbers:
Rounding large numbers or numbers in scientific notation can introduce unexpected results due to the limitations of floating-point arithmetic. The precision of floating-point numbers is finite, which means that extremely large or small numbers may not be represented accurately.
To round large numbers or numbers in scientific notation reliably, consider using the decimal module’s Decimal data type. Decimal provides precise decimal arithmetic and can handle extremely large or small numbers without loss of precision.
9.5 Rounding and Integer Division:
When performing division operations and rounding the result, be cautious of the potential impact of integer division. Integer division in Python truncates the decimal part and returns an integer result. If you round the result of an integer division, the rounding operation may have no effect since the value is already an integer.
To ensure accurate rounding, convert one or both of the operands to float or use the decimal module for decimal division. This will produce a floating-point result that can be rounded to the desired precision.
9.6 Incorrect Rounding Due to Intermediate Calculations:
If your rounding results are unexpected, inspect the intermediate calculations leading up to the rounding operation. Rounding errors can propagate from preceding calculations and affect the final result. Double-check the precision and rounding behavior at each step to identify any potential issues.
Consider adding debug statements or using a debugger to inspect intermediate values and verify the correctness of the calculations. This can help pinpoint where rounding errors may be introduced and guide you in finding solutions.
9.7 Verifying Rounding Results with Manual Calculations:
When in doubt about the correctness of rounding results, perform manual calculations to verify the expected rounded values. By independently performing the rounding using pen and paper or
a calculator, you can confirm whether the code’s output matches the manually calculated results.
Comparing the manual calculations with the code’s output will help identify any discrepancies or errors in the rounding process. This verification step is particularly useful when dealing with critical calculations or scenarios where precision is crucial.
By being aware of these common pitfalls and having troubleshooting strategies at your disposal, you can tackle rounding challenges with confidence. Understanding the limitations of floating-point arithmetic, handling negative numbers appropriately, and verifying results through manual calculations will ensure accurate rounding outcomes in your Python code.
Rounding is a nuanced operation, and being mindful of these potential issues will help you avoid errors and produce reliable results in your numerical computations.
Conclusion
Rounding numerical values to whole integers is a fundamental aspect of data processing and mathematical computations in Python. With the knowledge gained from this comprehensive guide, you are now equipped with various techniques and functions to round values with precision and control.
We began by exploring Python’s built-in round() function, which allows you to round values to the nearest whole number. We learned about the tie-breaking rule of rounding to the nearest even number for values ending in .5 and discussed alternatives like math.floor() and math.ceil() to achieve different rounding behaviors.
Furthermore, we delved into rounding all values in a Python list or array using powerful techniques such as list comprehensions and for loops. These methods enable you to process collections of numerical data efficiently and maintain code readability.
Throughout the article, we highlighted additional tips and considerations for rounding in Python. We discussed handling ties, rounding with specific precision, dealing with large numbers or scientific notation, and considerations for financial calculations. By taking these factors into account, you can ensure accurate and reliable results in your rounding operations.
Remember to select the appropriate rounding technique based on your specific requirements, whether it’s rounding to whole numbers, decimal places, or custom rounding behavior. Consider the potential impacts on subsequent calculations, and document your rounding decisions to enhance code transparency and reproducibility.
Rounding values in Python is a powerful tool for data manipulation, statistical analysis, and various scientific computations. By mastering the techniques presented in this article and considering the additional tips provided, you can confidently apply rounding operations in your Python projects.
So, embrace the versatility of Python’s rounding capabilities, and let precision be your guide in achieving accurate results in your numerical calculations.
Add comment